Note
Click here to download the full example code
Structured Deformations to Model Basipetal Growth¶
Curve and dots registration using implicit modules of order 1, learning the growth model tensor.
Curve registration using implicit modules of order with learned growth model tensor.
Import relevant Python modules.
import sys
sys.path.append("../../")
import math
import copy
import pickle
import torch
import matplotlib.pyplot as plt
torch.set_default_dtype(torch.float64)
import imodal
imodal.Utilities.set_compute_backend('torch')
Learning the growth model tensor¶
We load the data (shape and dots of the source and target leaves), rescale it and center it.
with open("../../data/basipetal.pickle", 'rb') as f:
data = pickle.load(f)
dots_source = torch.tensor(data['dots_source'], dtype=torch.get_default_dtype())
dots_target = torch.tensor(data['dots_target'], dtype=torch.get_default_dtype())
shape_source = imodal.Utilities.close_shape(torch.tensor(data['shape_source']).type(torch.get_default_dtype()))
shape_target = imodal.Utilities.close_shape(torch.tensor(data['shape_target']).type(torch.get_default_dtype()))
aabb_source = imodal.Utilities.AABB.build_from_points(shape_source)
aabb_target = imodal.Utilities.AABB.build_from_points(shape_target)
Plot source and target.
plt.title("Source and target")
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), color='black')
plt.plot(dots_source[:, 0].numpy(), dots_source[:, 1].numpy(), '.', color='black')
plt.plot(shape_target[:, 0].numpy(), shape_target[:, 1].numpy(), color='red')
plt.plot(dots_target[:, 0].numpy(), dots_target[:, 1].numpy(), '.', color='red')
plt.axis('equal')
plt.show()
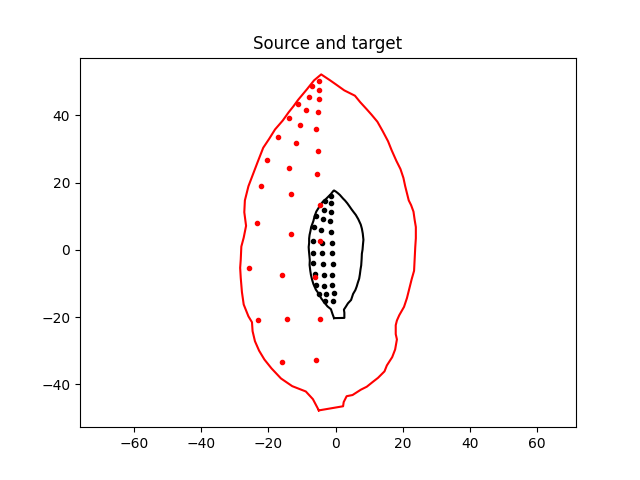
We now sample the points that will be used by the implicit deformation module of order 1 (growth module).
# Build AABB (Axis Aligned Bounding Box) around the source shape and uniformly sample points for the growth module.
#
growth_scale = 30.
points_density = 0.25
aabb_source = imodal.Utilities.AABB.build_from_points(shape_source)
points_growth = imodal.Utilities.fill_area_uniform_density(imodal.Utilities.area_shape, aabb_source, points_density, shape=shape_source)
# Initial normal frames for the growth module.
rot_growth = torch.stack([imodal.Utilities.rot2d(0.)]*points_growth.shape[0], axis=0)
Plot points of the growth module.
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), color='black')
plt.plot(points_growth[:, 0].numpy(), points_growth[:, 1].numpy(), 'o', color='blue')
plt.axis('equal')
plt.show()
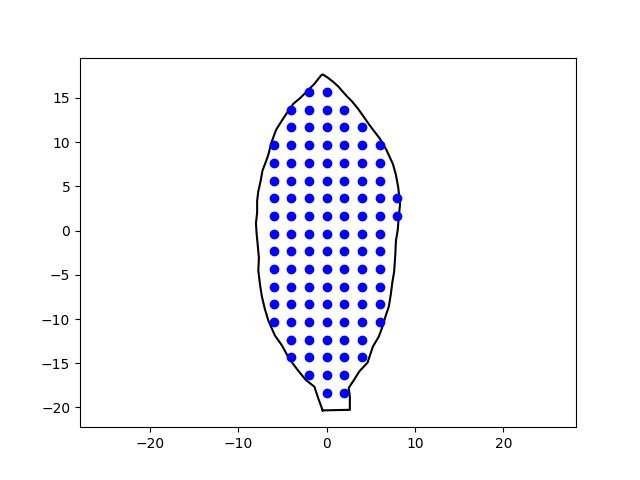
Create the deformation model with a combination of 3 modules : implicit module of order 1 (growth model), implicit module of order 0 (small corrections) and a global translation.
Create and initialize the global translation module.
global_translation = imodal.DeformationModules.GlobalTranslation(2)
Create and initialize the growth module.
nu = 0.001
coeff_growth = 0.001
scale_growth = 30.
C = torch.empty(points_growth.shape[0], 2, 1)
growth = imodal.DeformationModules.ImplicitModule1(2, points_growth.shape[0], scale_growth, C, coeff=coeff_growth, nu=nu, gd=(points_growth, rot_growth))
Create and initialize local translations module.
coeff_small = 1.
scale_small = 5.
points_small = shape_source.clone()
small_scale_translations = imodal.DeformationModules.ImplicitModule0(2, points_small.shape[0], scale_small, coeff=coeff_small, nu=nu, gd=points_small)
Define our growth model tensor function.
# The polynomial model for our growth factor.
def pol(pos, a, b, c, d):
return a + b*pos[:, 1] + c*pos[:, 1]**2 + d*pos[:, 1]**3
# Callback called when evaluating the model to compute the growth factor from parameters.
def callback_compute_c(init_manifold, modules, parameters, deformables):
abcd = parameters['abcd']['params'][0]
a = abcd[0].unsqueeze(1)
b = abcd[1].unsqueeze(1)
c = abcd[2].unsqueeze(1)
d = abcd[3].unsqueeze(1)
modules[3].C = pol(init_manifold[3].gd[0], a, b, c, d).transpose(0, 1).unsqueeze(2)
# Initial values of our growth model tensor parameters
abcd = torch.zeros(4, 2)
abcd[0] = 0.1 * torch.ones(2)
abcd.requires_grad_()
Out:
tensor([[0.1000, 0.1000],
[0.0000, 0.0000],
[0.0000, 0.0000],
[0.0000, 0.0000]], requires_grad=True)
Define deformables used by the registration model.
deformable_shape_source = imodal.Models.DeformablePoints(shape_source)
deformable_shape_target = imodal.Models.DeformablePoints(shape_target)
deformable_dots_source = imodal.Models.DeformablePoints(dots_source)
deformable_dots_target = imodal.Models.DeformablePoints(dots_target)
Registration¶
Define the registration model.
model = imodal.Models.RegistrationModel(
[deformable_shape_source, deformable_dots_source],
[global_translation, growth, small_scale_translations],
[imodal.Attachment.VarifoldAttachment(2, [20., 120.], backend='torch'),
imodal.Attachment.EuclideanPointwiseDistanceAttachment(10.)],
lam=10., other_parameters={'abcd': {'params': [abcd]}},
precompute_callback=callback_compute_c)
Fitting using Torch LBFGS optimizer.
shoot_solver = 'euler'
shoot_it = 10
costs = {}
fitter = imodal.Models.Fitter(model, optimizer='torch_lbfgs')
fitter.fit([deformable_shape_target, deformable_dots_target], 50, costs=costs, options={'shoot_solver': shoot_solver, 'shoot_it': shoot_it, 'line_search_fn': 'strong_wolfe'})
Out:
Starting optimization with method torch LBFGS, using solver euler with 10 iterations.
Initial cost={'deformation': 0.0, 'attach': 260650.27318793812}
1e-10
Evaluated model with costs=260650.27318793812
Evaluated model with costs=395766.89758865355
Evaluated model with costs=68185.7880412277
Evaluated model with costs=224881.5772645637
Evaluated model with costs=124231.83859028533
Evaluated model with costs=62087.138263158544
Evaluated model with costs=54453.26589377561
Evaluated model with costs=51993.379608900155
Evaluated model with costs=49067.37625584326
Evaluated model with costs=47960.60467256488
Evaluated model with costs=192610.95594750595
Evaluated model with costs=47127.857868695406
Evaluated model with costs=46191.893914818196
Evaluated model with costs=44652.25851315569
Evaluated model with costs=41995.80047684307
Evaluated model with costs=37732.476019983704
Evaluated model with costs=32320.946325746074
Evaluated model with costs=35359.02275547691
Evaluated model with costs=29642.67347493438
Evaluated model with costs=26746.117134686974
Evaluated model with costs=23177.66945431049
Evaluated model with costs=21716.51269850582
Evaluated model with costs=21328.825385398715
Evaluated model with costs=21143.586988600728
Evaluated model with costs=21110.9022486214
================================================================================
Time: 44.48841466400336
Iteration: 0
Costs
deformation=12.677747600215872
attach=21098.224501021185
Total cost=21110.9022486214
1e-10
Evaluated model with costs=21110.9022486214
Evaluated model with costs=21050.557194984485
Evaluated model with costs=20951.144091729373
Evaluated model with costs=20543.837289708492
Evaluated model with costs=19230.974581345818
Evaluated model with costs=17374.60889644207
Evaluated model with costs=14868.818716593183
Evaluated model with costs=13189.92486585529
Evaluated model with costs=12202.385543354923
Evaluated model with costs=11786.331168346072
Evaluated model with costs=11731.739474064145
Evaluated model with costs=11727.418708467303
Evaluated model with costs=11727.155355528066
Evaluated model with costs=11725.541224124754
Evaluated model with costs=11720.211610014125
Evaluated model with costs=11534.439551612519
Evaluated model with costs=14312.6623290006
Evaluated model with costs=11276.152285669394
Evaluated model with costs=11050.507417628887
Evaluated model with costs=10932.972841143132
Evaluated model with costs=10669.288660219434
Evaluated model with costs=10611.688822667867
================================================================================
Time: 81.5583022910032
Iteration: 1
Costs
deformation=12.65835505520919
attach=10599.030467612658
Total cost=10611.688822667867
1e-10
Evaluated model with costs=10611.688822667867
Evaluated model with costs=10576.555525471207
Evaluated model with costs=10489.550818276228
Evaluated model with costs=10584.144301499815
Evaluated model with costs=10421.780529911028
Evaluated model with costs=10390.578294963001
Evaluated model with costs=10317.466501726189
Evaluated model with costs=10394.576721745058
Evaluated model with costs=10301.268201725683
Evaluated model with costs=10282.622324552909
Evaluated model with costs=10289.255675390845
Evaluated model with costs=10276.033710574678
Evaluated model with costs=10273.179331806692
Evaluated model with costs=10266.78462076542
Evaluated model with costs=10262.960250332964
Evaluated model with costs=10260.190152698493
Evaluated model with costs=10259.259012817027
Evaluated model with costs=10259.0768043578
Evaluated model with costs=10260.066063978473
Evaluated model with costs=10258.953865172367
Evaluated model with costs=10258.871967549987
Evaluated model with costs=10258.846175759492
Evaluated model with costs=10258.781067143274
Evaluated model with costs=10258.711364529174
Evaluated model with costs=10258.59840750541
================================================================================
Time: 122.7708115580026
Iteration: 2
Costs
deformation=16.473600856937406
attach=10242.124806648471
Total cost=10258.59840750541
1e-10
Evaluated model with costs=10258.59840750541
Evaluated model with costs=10258.320815684066
Evaluated model with costs=10257.864302019365
Evaluated model with costs=10256.519436467024
Evaluated model with costs=10253.55745318024
Evaluated model with costs=10246.83103968207
Evaluated model with costs=10234.005565101967
Evaluated model with costs=10218.953920296643
Evaluated model with costs=10246.524524646937
Evaluated model with costs=10207.36228165654
Evaluated model with costs=10201.17143918451
Evaluated model with costs=10194.277703492311
Evaluated model with costs=10182.225622463651
Evaluated model with costs=10105.903496570932
Evaluated model with costs=15002.158717828246
Evaluated model with costs=10059.814373082148
Evaluated model with costs=10022.856412240712
Evaluated model with costs=9963.41385704409
Evaluated model with costs=10582.282026606947
Evaluated model with costs=9934.876581093495
Evaluated model with costs=9891.844323861797
Evaluated model with costs=9853.562983315956
Evaluated model with costs=9808.887734766802
Evaluated model with costs=9675.422691796113
Evaluated model with costs=9506.804622142563
================================================================================
Time: 164.46450480200292
Iteration: 3
Costs
deformation=15.393655253742567
attach=9491.41096688882
Total cost=9506.804622142563
1e-10
Evaluated model with costs=9506.804622142563
Evaluated model with costs=9396.369667719047
Evaluated model with costs=9348.047026536233
Evaluated model with costs=9276.592990109784
Evaluated model with costs=9271.865516524527
Evaluated model with costs=9259.188838856377
Evaluated model with costs=9234.001309562147
Evaluated model with costs=9191.66689270496
Evaluated model with costs=9149.212480016922
Evaluated model with costs=9092.725550383624
Evaluated model with costs=9078.683661104664
Evaluated model with costs=9058.972767744466
Evaluated model with costs=9052.662881318862
Evaluated model with costs=9047.336621773926
Evaluated model with costs=9045.931756594082
Evaluated model with costs=9045.2243141524
Evaluated model with costs=9045.12755988828
Evaluated model with costs=9045.11061644338
Evaluated model with costs=9045.108194845421
Evaluated model with costs=9045.107757387206
Evaluated model with costs=9045.107219316084
================================================================================
Time: 199.8913683690007
Iteration: 4
Costs
deformation=18.052172804543414
attach=9027.05504651154
Total cost=9045.107219316084
1e-10
Evaluated model with costs=9045.107219316084
Evaluated model with costs=9045.10400488066
Evaluated model with costs=9045.097943863944
Evaluated model with costs=9045.081065306424
Evaluated model with costs=9045.039609338988
Evaluated model with costs=9044.931499811835
Evaluated model with costs=9044.652760829238
Evaluated model with costs=9043.921852666928
Evaluated model with costs=9041.99698704456
Evaluated model with costs=9036.924416098174
Evaluated model with costs=9023.909565693286
Evaluated model with costs=8993.490164472563
Evaluated model with costs=8937.843291790181
Evaluated model with costs=8856.439207538751
Evaluated model with costs=8845.008408232457
Evaluated model with costs=8795.779126978823
Evaluated model with costs=8827.05715944348
Evaluated model with costs=8728.358278499056
Evaluated model with costs=8706.881128664101
Evaluated model with costs=8676.573388463632
Evaluated model with costs=8650.093255406418
Evaluated model with costs=8627.21904084052
Evaluated model with costs=8612.438934765047
================================================================================
Time: 238.38453926500006
Iteration: 5
Costs
deformation=25.706769914572188
attach=8586.732164850475
Total cost=8612.438934765047
1e-10
Evaluated model with costs=8612.438934765047
Evaluated model with costs=8607.946751868483
Evaluated model with costs=8604.380887712572
Evaluated model with costs=8603.503158678448
Evaluated model with costs=8603.814424314995
Evaluated model with costs=8603.143191668576
Evaluated model with costs=8605.569029589098
Evaluated model with costs=8603.059307199312
Evaluated model with costs=8603.162540213718
Evaluated model with costs=8602.94242234108
Evaluated model with costs=8602.937636315623
Evaluated model with costs=8602.906405842346
Evaluated model with costs=8603.87681360368
Evaluated model with costs=8602.9031313192
Evaluated model with costs=8602.893840359184
Evaluated model with costs=8602.892023309014
Evaluated model with costs=8605.840587604367
Evaluated model with costs=8602.888245770879
Evaluated model with costs=8602.97121270638
Evaluated model with costs=8602.88216250732
Evaluated model with costs=8602.880938558174
Evaluated model with costs=8602.879670588056
Evaluated model with costs=8602.87911639309
Evaluated model with costs=8602.878039608446
Evaluated model with costs=8602.877713307344
Evaluated model with costs=8602.876784777485
Evaluated model with costs=8602.876573618672
Evaluated model with costs=8602.875770852319
Evaluated model with costs=8602.875630457762
Evaluated model with costs=8602.874975863184
================================================================================
Time: 290.97346405200005
Iteration: 6
Costs
deformation=27.13343826684887
attach=8575.741537596336
Total cost=8602.874975863184
1e-10
Evaluated model with costs=8602.874975863184
Evaluated model with costs=8606.342549460633
Evaluated model with costs=8602.92048441962
Evaluated model with costs=8602.87468261348
Evaluated model with costs=8603.182181756618
Evaluated model with costs=8602.881968743397
Evaluated model with costs=8602.8745742986
Evaluated model with costs=8602.888243854732
Evaluated model with costs=8602.875403565946
Evaluated model with costs=8602.874192741054
Evaluated model with costs=8602.916755349508
Evaluated model with costs=8602.870618050229
Evaluated model with costs=8602.870067806944
Evaluated model with costs=8602.869977485288
Evaluated model with costs=8602.86997048333
Evaluated model with costs=8602.869956708408
Evaluated model with costs=8602.869777258342
Evaluated model with costs=8602.871321793773
Evaluated model with costs=8602.869548089602
Evaluated model with costs=8602.871227092612
Evaluated model with costs=8602.869740946584
Evaluated model with costs=8602.869507445048
Evaluated model with costs=8602.868272620042
Evaluated model with costs=8602.856979960998
Evaluated model with costs=8602.812806944405
================================================================================
Time: 349.0676652030015
Iteration: 7
Costs
deformation=27.121130684661775
attach=8575.691676259743
Total cost=8602.812806944405
1e-10
Evaluated model with costs=8602.812806944405
Evaluated model with costs=8602.707592879515
Evaluated model with costs=8602.437720381427
Evaluated model with costs=8601.723546833477
Evaluated model with costs=8599.850786328872
Evaluated model with costs=8594.958567412496
Evaluated model with costs=8582.485206864814
Evaluated model with costs=8553.438107340162
Evaluated model with costs=8507.763293231785
Evaluated model with costs=8473.20108703557
Evaluated model with costs=8459.815411682133
Evaluated model with costs=8458.496133528668
Evaluated model with costs=8458.39771319024
Evaluated model with costs=8458.387134266142
Evaluated model with costs=8458.382148353077
Evaluated model with costs=8458.373103540229
Evaluated model with costs=8458.346944162748
Evaluated model with costs=8458.280909861438
Evaluated model with costs=8458.105794766672
Evaluated model with costs=8457.649318507001
Evaluated model with costs=8456.46156252409
================================================================================
Time: 386.14507824900284
Iteration: 8
Costs
deformation=25.304790968938644
attach=8431.15677155515
Total cost=8456.46156252409
1e-10
Evaluated model with costs=8456.46156252409
Evaluated model with costs=8453.414039858015
Evaluated model with costs=8445.854593393005
Evaluated model with costs=8428.829441452059
Evaluated model with costs=8399.801289398034
Evaluated model with costs=8373.993208655038
Evaluated model with costs=8361.710421914726
Evaluated model with costs=8358.701989510126
Evaluated model with costs=8358.589603205497
Evaluated model with costs=8358.5844375176
Evaluated model with costs=8358.583655824634
Evaluated model with costs=8358.583494559465
Evaluated model with costs=8358.58336084336
Evaluated model with costs=8358.582854934648
Evaluated model with costs=8358.581691463278
Evaluated model with costs=8358.578485771395
Evaluated model with costs=8358.570261503042
Evaluated model with costs=8358.548614566469
Evaluated model with costs=8358.492372680908
Evaluated model with costs=8358.346692995246
Evaluated model with costs=8357.976789353896
================================================================================
Time: 424.8014542790006
Iteration: 9
Costs
deformation=25.802850206315284
attach=8332.17393914758
Total cost=8357.976789353896
1e-10
Evaluated model with costs=8357.976789353896
Evaluated model with costs=8357.083545482554
Evaluated model with costs=8355.205990351973
Evaluated model with costs=8352.278547805934
Evaluated model with costs=8348.983823892106
Evaluated model with costs=8346.31794624397
Evaluated model with costs=8345.61490262587
Evaluated model with costs=8345.55450286737
Evaluated model with costs=8345.538082236419
Evaluated model with costs=8345.534326201112
Evaluated model with costs=8345.533648077324
Evaluated model with costs=8345.533609899545
Evaluated model with costs=8345.533608713893
Evaluated model with costs=8345.533608533597
Evaluated model with costs=8345.533606385723
Evaluated model with costs=8345.533720767424
Evaluated model with costs=8345.533602942129
Evaluated model with costs=8345.533593569538
Evaluated model with costs=8345.533491136113
Evaluated model with costs=8345.533266891627
Evaluated model with costs=8345.532639642806
Evaluated model with costs=8345.531038141653
================================================================================
Time: 462.69400277800014
Iteration: 10
Costs
deformation=24.770692802591594
attach=8320.760345339062
Total cost=8345.531038141653
1e-10
Evaluated model with costs=8345.531038141653
Evaluated model with costs=8345.526807970882
Evaluated model with costs=8345.515783518238
Evaluated model with costs=8345.48692553761
Evaluated model with costs=8345.41160316124
Evaluated model with costs=8345.215184812168
Evaluated model with costs=8344.704547362475
Evaluated model with costs=8343.383123210817
Evaluated model with costs=8339.98981948475
Evaluated model with costs=8337.572840103956
Evaluated model with costs=8322.496617498386
Evaluated model with costs=8293.958223199352
Evaluated model with costs=8315.847988457017
Evaluated model with costs=8201.492006337372
Evaluated model with costs=8133.15495582961
Evaluated model with costs=8328.358760866897
Evaluated model with costs=8084.3196419734195
Evaluated model with costs=8062.715018652185
Evaluated model with costs=8051.757325430596
Evaluated model with costs=8021.097625566641
Evaluated model with costs=7995.9129131827885
Evaluated model with costs=7954.83465542517
Evaluated model with costs=7917.34712560384
================================================================================
Time: 501.4744493470025
Iteration: 11
Costs
deformation=19.04846079724089
attach=7898.298664806599
Total cost=7917.34712560384
1e-10
Evaluated model with costs=7917.34712560384
Evaluated model with costs=11249.281476198259
Evaluated model with costs=7910.539480927593
Evaluated model with costs=7883.3679447983895
Evaluated model with costs=7859.788051106735
Evaluated model with costs=7857.12178622736
Evaluated model with costs=7832.604138814273
Evaluated model with costs=7821.97299052278
Evaluated model with costs=7808.258847297211
Evaluated model with costs=7785.22157903835
Evaluated model with costs=7753.980505683484
Evaluated model with costs=7743.224856212178
Evaluated model with costs=7735.837388416696
Evaluated model with costs=7731.8352459052285
Evaluated model with costs=16558.28080338915
Evaluated model with costs=7731.765912550271
Evaluated model with costs=17352.721555269436
Evaluated model with costs=7737.000161054652
Evaluated model with costs=7730.764213244962
Evaluated model with costs=11168.938881812717
Evaluated model with costs=7790.742575514625
Evaluated model with costs=7725.497196161278
Evaluated model with costs=7710.666043154475
Evaluated model with costs=7692.837440377142
Evaluated model with costs=7770.6242055513
Evaluated model with costs=7681.248813921895
================================================================================
Time: 544.9536575100028
Iteration: 12
Costs
deformation=14.251849841475865
attach=7666.996964080419
Total cost=7681.248813921895
1e-10
Evaluated model with costs=7681.248813921895
Evaluated model with costs=7666.923161210761
Evaluated model with costs=7677.506763751717
Evaluated model with costs=7657.1426946887605
Evaluated model with costs=7650.2211400475735
Evaluated model with costs=7647.642605765293
Evaluated model with costs=7639.172907094357
Evaluated model with costs=7629.59965401733
Evaluated model with costs=10235.739135356014
Evaluated model with costs=7668.804455472916
Evaluated model with costs=7618.360382112744
Evaluated model with costs=7614.892115697328
Evaluated model with costs=7611.191796089405
Evaluated model with costs=7607.143744334859
Evaluated model with costs=7604.260480308525
Evaluated model with costs=7601.352240457991
Evaluated model with costs=7595.846482822681
Evaluated model with costs=7590.481311678225
Evaluated model with costs=7588.234530846351
Evaluated model with costs=7584.592580079468
Evaluated model with costs=7581.56812014326
Evaluated model with costs=7582.416924188384
Evaluated model with costs=7578.244749491399
Evaluated model with costs=7578.453793076185
Evaluated model with costs=7576.912180540788
================================================================================
Time: 586.987447853
Iteration: 13
Costs
deformation=11.970168423398484
attach=7564.942012117389
Total cost=7576.912180540788
1e-10
Evaluated model with costs=7576.912180540788
Evaluated model with costs=7574.159087090918
Evaluated model with costs=7571.6946232534865
Evaluated model with costs=7571.659803630123
Evaluated model with costs=7569.617762596124
Evaluated model with costs=7568.047130066567
Evaluated model with costs=7586.07981615099
Evaluated model with costs=7564.879559341714
Evaluated model with costs=7563.714642280749
Evaluated model with costs=7561.595580003224
Evaluated model with costs=7567.045056136782
Evaluated model with costs=7559.84854899873
Evaluated model with costs=7558.7452978966785
Evaluated model with costs=7556.265012357153
Evaluated model with costs=7558.772689808531
Evaluated model with costs=7555.361341724966
Evaluated model with costs=7554.630537623469
Evaluated model with costs=7552.630547836795
Evaluated model with costs=7551.870700528098
Evaluated model with costs=7550.770976752452
Evaluated model with costs=7551.078045215099
Evaluated model with costs=7550.220792410595
Evaluated model with costs=7549.179939032582
Evaluated model with costs=7547.660318324945
Evaluated model with costs=7547.2869661128125
================================================================================
Time: 628.9500554310034
Iteration: 14
Costs
deformation=11.231178015762545
attach=7536.05578809705
Total cost=7547.2869661128125
1e-10
Evaluated model with costs=7547.2869661128125
Evaluated model with costs=7546.695141239982
Evaluated model with costs=7546.034941007279
Evaluated model with costs=7554.569846047572
Evaluated model with costs=7545.394180218555
Evaluated model with costs=7544.839207868451
Evaluated model with costs=7546.425470142902
Evaluated model with costs=7544.360987160924
Evaluated model with costs=7544.946750064321
Evaluated model with costs=7544.112232715527
Evaluated model with costs=7543.7628344370205
Evaluated model with costs=7543.28291651284
Evaluated model with costs=7543.442401213514
Evaluated model with costs=7542.864992582556
Evaluated model with costs=7542.52863821017
Evaluated model with costs=7541.993165994433
Evaluated model with costs=7543.585112384843
Evaluated model with costs=7541.576758447182
Evaluated model with costs=7541.416691478648
Evaluated model with costs=7541.085920437691
Evaluated model with costs=7542.490867631595
Evaluated model with costs=7540.972739606254
Evaluated model with costs=7540.747792528053
Evaluated model with costs=7540.56911488583
Evaluated model with costs=7540.317177025264
================================================================================
Time: 670.9259952200009
Iteration: 15
Costs
deformation=11.055426099713568
attach=7529.261750925551
Total cost=7540.317177025264
1e-10
Evaluated model with costs=7540.317177025264
Evaluated model with costs=7540.558351431889
Evaluated model with costs=7540.232646270572
Evaluated model with costs=7540.116298256384
Evaluated model with costs=7539.870607735509
Evaluated model with costs=7539.881269140578
Evaluated model with costs=7539.805820254714
Evaluated model with costs=7539.6901280489865
Evaluated model with costs=7539.532036959425
Evaluated model with costs=7539.779833753154
Evaluated model with costs=7539.491438070574
Evaluated model with costs=7539.2166617437715
Evaluated model with costs=7539.174176060806
Evaluated model with costs=7538.971233409063
Evaluated model with costs=7538.839354552165
Evaluated model with costs=7538.685544085979
Evaluated model with costs=7538.6889992999295
Evaluated model with costs=7538.619698582686
Evaluated model with costs=7538.540960381898
Evaluated model with costs=7538.469460809946
Evaluated model with costs=7538.322935868656
Evaluated model with costs=7538.2060423747
Evaluated model with costs=7541.689228641993
Evaluated model with costs=7538.204360661448
Evaluated model with costs=7538.172490975273
================================================================================
Time: 712.8757600410026
Iteration: 16
Costs
deformation=10.967083693295848
attach=7527.205407281977
Total cost=7538.172490975273
1e-10
Evaluated model with costs=7538.172490975273
Evaluated model with costs=7538.116092807492
Evaluated model with costs=7538.019196962452
Evaluated model with costs=7537.947333601726
Evaluated model with costs=7537.826887845847
Evaluated model with costs=7537.811863172444
Evaluated model with costs=7537.746563412984
Evaluated model with costs=7540.259120834356
Evaluated model with costs=7537.731564802971
Evaluated model with costs=7537.684629568298
Evaluated model with costs=7537.686140798588
Evaluated model with costs=7537.67022885195
Evaluated model with costs=7537.578546567217
Evaluated model with costs=7541.642355150837
Evaluated model with costs=7537.582616045256
Evaluated model with costs=7537.567283321104
Evaluated model with costs=7537.542476953281
Evaluated model with costs=7537.492121665996
Evaluated model with costs=7537.463837540897
Evaluated model with costs=7537.414344819258
Evaluated model with costs=7537.685705758746
Evaluated model with costs=7537.41849980287
Evaluated model with costs=7537.412062368936
Evaluated model with costs=7537.386476242603
Evaluated model with costs=7537.377979462218
================================================================================
Time: 754.6731514780004
Iteration: 17
Costs
deformation=10.919905608672014
attach=7526.458073853546
Total cost=7537.377979462218
1e-10
Evaluated model with costs=7537.377979462218
Evaluated model with costs=7537.362380747015
Evaluated model with costs=7537.347309300622
Evaluated model with costs=7537.332982359061
Evaluated model with costs=7537.403803514714
Evaluated model with costs=7537.310862780441
Evaluated model with costs=7537.292026105672
Evaluated model with costs=7537.281178416555
Evaluated model with costs=7537.279411887486
Evaluated model with costs=7537.263257741122
Evaluated model with costs=7537.252326739875
Evaluated model with costs=7537.245662227361
Evaluated model with costs=7537.229757700308
Evaluated model with costs=7537.217206560485
Evaluated model with costs=7537.2032966152
Evaluated model with costs=7537.1781771868955
Evaluated model with costs=7537.1727164296535
Evaluated model with costs=7537.151163798444
Evaluated model with costs=7537.1867619094355
Evaluated model with costs=7537.144425486695
Evaluated model with costs=7537.13864867887
Evaluated model with costs=7537.1149515246225
Evaluated model with costs=7537.140119402121
Evaluated model with costs=7537.111724409789
Evaluated model with costs=7537.10186759184
================================================================================
Time: 796.7377717400013
Iteration: 18
Costs
deformation=10.900022555259117
attach=7526.201845036581
Total cost=7537.10186759184
1e-10
Evaluated model with costs=7537.10186759184
Evaluated model with costs=7537.088457796695
Evaluated model with costs=7537.1242496902505
Evaluated model with costs=7537.085950146662
Evaluated model with costs=7537.080045456863
Evaluated model with costs=7537.067814174632
Evaluated model with costs=7537.089319903994
Evaluated model with costs=7537.066113292818
Evaluated model with costs=7537.061366852554
Evaluated model with costs=7537.050610384779
Evaluated model with costs=7537.076726348434
Evaluated model with costs=7537.046966311186
Evaluated model with costs=7537.042224804053
Evaluated model with costs=7537.035421130188
Evaluated model with costs=7537.029921494028
Evaluated model with costs=7537.027484195504
Evaluated model with costs=7537.024372650799
Evaluated model with costs=7537.023450912486
Evaluated model with costs=7537.018408695518
Evaluated model with costs=7537.012728050128
Evaluated model with costs=7537.062702815024
Evaluated model with costs=7537.012294063331
Evaluated model with costs=7537.007884350685
Evaluated model with costs=7537.001419304989
Evaluated model with costs=7536.996585703887
================================================================================
Time: 838.7307317210034
Iteration: 19
Costs
deformation=10.888219795009682
attach=7526.108365908877
Total cost=7536.996585703887
1e-10
Evaluated model with costs=7536.996585703887
Evaluated model with costs=7536.9925701479315
Evaluated model with costs=7536.98799834395
Evaluated model with costs=7536.9846892398
Evaluated model with costs=7536.985520261309
Evaluated model with costs=7536.9827876463305
Evaluated model with costs=7536.9818936176025
Evaluated model with costs=7536.97600729215
Evaluated model with costs=7537.067034826129
Evaluated model with costs=7536.977180206311
Evaluated model with costs=7536.975972772529
Evaluated model with costs=7536.973831264862
Evaluated model with costs=7536.972111938766
Evaluated model with costs=7536.970483448569
Evaluated model with costs=7536.969813674854
Evaluated model with costs=7536.96516003027
Evaluated model with costs=7536.96516946078
Evaluated model with costs=7536.964423894197
Evaluated model with costs=7536.962999998534
Evaluated model with costs=7536.9624958820505
Evaluated model with costs=7536.961063916339
Evaluated model with costs=7536.9612857000275
Evaluated model with costs=7536.9604398623
Evaluated model with costs=7536.958398726468
Evaluated model with costs=7536.967389256661
Evaluated model with costs=7536.957800523723
================================================================================
Time: 882.4070175990018
Iteration: 20
Costs
deformation=10.882946579472616
attach=7526.07485394425
Total cost=7536.957800523723
1e-10
Evaluated model with costs=7536.957800523723
Evaluated model with costs=7536.957009716288
Evaluated model with costs=7536.954738954051
Evaluated model with costs=7536.960962858123
Evaluated model with costs=7536.9540395619515
Evaluated model with costs=7536.95304783026
Evaluated model with costs=7536.951154959156
Evaluated model with costs=7536.952223251739
Evaluated model with costs=7536.950373188891
Evaluated model with costs=7536.949173811469
Evaluated model with costs=7536.94849971884
Evaluated model with costs=7536.947383239222
Evaluated model with costs=7536.947151320452
Evaluated model with costs=7536.946165588242
Evaluated model with costs=7536.9467963602865
Evaluated model with costs=7536.9457766856585
Evaluated model with costs=7536.944649671654
Evaluated model with costs=7537.104567690511
Evaluated model with costs=7536.945094770645
Evaluated model with costs=7536.944637384279
Evaluated model with costs=7536.944044419524
Evaluated model with costs=7536.943023219389
Evaluated model with costs=7536.942273535644
Evaluated model with costs=7536.941693495142
Evaluated model with costs=7536.940710196655
================================================================================
Time: 924.3020955840002
Iteration: 21
Costs
deformation=10.87899251986135
attach=7526.061717676794
Total cost=7536.940710196655
1e-10
Evaluated model with costs=7536.940710196655
Evaluated model with costs=7536.940226564808
Evaluated model with costs=7536.939247458773
Evaluated model with costs=7536.937595356372
Evaluated model with costs=7536.936702746238
Evaluated model with costs=7536.943758007013
Evaluated model with costs=7536.936476337517
Evaluated model with costs=7536.935811351822
Evaluated model with costs=7536.935050730207
Evaluated model with costs=7536.935064147279
Evaluated model with costs=7536.934813020517
Evaluated model with costs=7536.934333522549
Evaluated model with costs=7536.934003662055
Evaluated model with costs=7536.9348326174595
Evaluated model with costs=7536.933627064742
Evaluated model with costs=7536.933369279919
Evaluated model with costs=7536.933198548969
Evaluated model with costs=7536.932667208565
Evaluated model with costs=7536.932260411655
Evaluated model with costs=7536.940233633531
Evaluated model with costs=7536.931939774384
Evaluated model with costs=7536.9315240799515
Evaluated model with costs=7536.931460739337
Evaluated model with costs=7536.930872146026
Evaluated model with costs=7536.932504336327
Evaluated model with costs=7536.930778847559
================================================================================
Time: 967.7024181190027
Iteration: 22
Costs
deformation=10.876543421429846
attach=7526.054235426129
Total cost=7536.930778847559
1e-10
Evaluated model with costs=7536.930778847559
Evaluated model with costs=7536.930720968553
Evaluated model with costs=7536.930545581017
Evaluated model with costs=7536.930357012648
Evaluated model with costs=7536.929890495754
Evaluated model with costs=7536.92960462878
Evaluated model with costs=7536.930397512073
Evaluated model with costs=7536.92925973845
Evaluated model with costs=7536.929143940145
Evaluated model with costs=7536.929013071413
Evaluated model with costs=7536.92906446343
Evaluated model with costs=7536.928863028826
Evaluated model with costs=7536.928675509969
Evaluated model with costs=7536.928424569213
Evaluated model with costs=7536.929898937792
Evaluated model with costs=7536.928248678897
Evaluated model with costs=7536.928071909873
Evaluated model with costs=7536.927785698223
Evaluated model with costs=7536.929134201043
Evaluated model with costs=7536.927698625115
Evaluated model with costs=7536.927521717542
Evaluated model with costs=7536.927317271112
Evaluated model with costs=7536.927308548827
Evaluated model with costs=7536.927167981501
Evaluated model with costs=7536.9270594041545
================================================================================
Time: 1009.670527151
Iteration: 23
Costs
deformation=10.875122015702392
attach=7526.051937388452
Total cost=7536.9270594041545
1e-10
Evaluated model with costs=7536.9270594041545
Evaluated model with costs=7536.926792141484
Evaluated model with costs=7536.926707543444
Evaluated model with costs=7536.926646546758
Evaluated model with costs=7536.926526317291
Evaluated model with costs=7536.9275926159635
Evaluated model with costs=7536.926548094174
Evaluated model with costs=7536.926515449611
Evaluated model with costs=7536.926515564931
Evaluated model with costs=7536.926500425686
Evaluated model with costs=7536.926446902667
Evaluated model with costs=7536.926420383817
Evaluated model with costs=7536.926345668603
Evaluated model with costs=7536.926343089205
Evaluated model with costs=7536.926244197029
Evaluated model with costs=7536.926061352047
Evaluated model with costs=7536.925904072902
Evaluated model with costs=7536.925844105524
Evaluated model with costs=7536.925807409038
Evaluated model with costs=7536.9257587907805
Evaluated model with costs=7536.925687290507
Evaluated model with costs=7536.925910047986
Evaluated model with costs=7536.9256772190565
Evaluated model with costs=7536.92560689584
Evaluated model with costs=7536.925474820552
================================================================================
Time: 1051.7608195510002
Iteration: 24
Costs
deformation=10.873855734120797
attach=7526.051619086431
Total cost=7536.925474820552
1e-10
Evaluated model with costs=7536.925474820552
Evaluated model with costs=7536.925412898153
Evaluated model with costs=7536.925290636076
Evaluated model with costs=7536.925144245619
Evaluated model with costs=7536.924888621058
Evaluated model with costs=7536.927168219597
Evaluated model with costs=7536.924835259568
Evaluated model with costs=7536.924603548822
Evaluated model with costs=7536.924385626685
Evaluated model with costs=7536.9241309907175
Evaluated model with costs=7536.924139738716
Evaluated model with costs=7536.924094396901
Evaluated model with costs=7536.924022253579
Evaluated model with costs=7536.923854489863
Evaluated model with costs=7536.9236986463675
Evaluated model with costs=7536.923447936594
Evaluated model with costs=7536.9230996099795
Evaluated model with costs=7536.922775594915
Evaluated model with costs=7536.922108718022
Evaluated model with costs=7536.921671287518
Evaluated model with costs=7536.925863270322
Evaluated model with costs=7536.921276023737
Evaluated model with costs=7536.920197485733
Evaluated model with costs=7536.918750339295
================================================================================
Time: 1092.297882916002
Iteration: 25
Costs
deformation=10.86950475842098
attach=7526.049245580874
Total cost=7536.918750339295
1e-10
Evaluated model with costs=7536.918750339295
Evaluated model with costs=7536.916995326211
Evaluated model with costs=7536.913983598253
Evaluated model with costs=7536.912389063539
Evaluated model with costs=7536.907545465806
Evaluated model with costs=7536.9152149323445
Evaluated model with costs=7536.901301471214
Evaluated model with costs=7536.89416110859
Evaluated model with costs=7536.867335941472
Evaluated model with costs=7536.82982976372
Evaluated model with costs=7536.908566428546
Evaluated model with costs=7536.808348997673
Evaluated model with costs=7536.743029604158
Evaluated model with costs=7536.640766326859
Evaluated model with costs=7536.514287965343
Evaluated model with costs=7536.703720049055
Evaluated model with costs=7536.427817374949
Evaluated model with costs=7536.229976721548
Evaluated model with costs=7535.829732381998
Evaluated model with costs=7536.949534939872
Evaluated model with costs=7535.645948058465
Evaluated model with costs=7535.164492402331
Evaluated model with costs=7534.4890112326875
Evaluated model with costs=7533.725031998709
Evaluated model with costs=7532.11645044285
================================================================================
Time: 1134.2284505020034
Iteration: 26
Costs
deformation=10.833726291375413
attach=7521.282724151474
Total cost=7532.11645044285
1e-10
Evaluated model with costs=7532.11645044285
Evaluated model with costs=7531.408690303235
Evaluated model with costs=7529.335674086857
Evaluated model with costs=8576.989542150766
Evaluated model with costs=7563.153039269246
Evaluated model with costs=7528.108514347942
Evaluated model with costs=7526.68763676236
Evaluated model with costs=7522.667622005601
Evaluated model with costs=21792.870741161296
Evaluated model with costs=7522.287708836872
Evaluated model with costs=7518.984689644217
Evaluated model with costs=7624.634415905626
Evaluated model with costs=7515.8555640000895
Evaluated model with costs=7515.075247569506
Evaluated model with costs=7525.366351686756
Evaluated model with costs=7514.443455710227
Evaluated model with costs=7513.64462852798
Evaluated model with costs=7523.537399331049
Evaluated model with costs=7511.762288445159
Evaluated model with costs=7514.799350798359
Evaluated model with costs=7509.354610147876
Evaluated model with costs=7506.993624512512
Evaluated model with costs=246588.41413327918
Evaluated model with costs=7506.987608296185
Evaluated model with costs=7640.325129135289
Evaluated model with costs=7507.5301922906465
Evaluated model with costs=7506.727958858134
================================================================================
Time: 1179.0232437850027
Iteration: 27
Costs
deformation=10.90530860611862
attach=7495.822650252016
Total cost=7506.727958858134
1e-10
Evaluated model with costs=7506.727958858134
Evaluated model with costs=7507.170030390435
Evaluated model with costs=7506.297562990115
Evaluated model with costs=7506.434198038731
Evaluated model with costs=7505.178277299737
Evaluated model with costs=7503.7816182338765
Evaluated model with costs=7502.627950863418
Evaluated model with costs=7509.46128057428
Evaluated model with costs=7501.011838136053
Evaluated model with costs=7502.370428658122
Evaluated model with costs=7500.565797828749
Evaluated model with costs=7499.645093206725
Evaluated model with costs=7498.827138274841
Evaluated model with costs=7497.481855520315
Evaluated model with costs=7496.259486982683
Evaluated model with costs=7497.252666861933
Evaluated model with costs=7495.454154013586
Evaluated model with costs=7494.486862316021
Evaluated model with costs=7493.755912668147
Evaluated model with costs=7493.600108126313
Evaluated model with costs=7493.382862598048
Evaluated model with costs=7492.979421810897
Evaluated model with costs=7492.830372618675
Evaluated model with costs=7494.147097532601
Evaluated model with costs=7492.660556616756
================================================================================
Time: 1220.9109083350013
Iteration: 28
Costs
deformation=10.868683234556682
attach=7481.7918733822
Total cost=7492.660556616756
1e-10
Evaluated model with costs=7492.660556616756
Evaluated model with costs=7492.297641170102
Evaluated model with costs=7490.576414465867
Evaluated model with costs=7486.1013615532875
Evaluated model with costs=8049.24069701198
Evaluated model with costs=7506.853266974626
Evaluated model with costs=7483.16728068591
Evaluated model with costs=7859.831238378471
Evaluated model with costs=7495.369881835053
Evaluated model with costs=7477.585117379808
Evaluated model with costs=7547.038124685666
Evaluated model with costs=7477.606701525534
Evaluated model with costs=7475.765800516932
Evaluated model with costs=8414.991987525073
Evaluated model with costs=7472.146156174646
Evaluated model with costs=32908.223022160186
Evaluated model with costs=7472.108383891611
Evaluated model with costs=7462.905237323715
Evaluated model with costs=19013.00369262278
Evaluated model with costs=7462.8606450065945
Evaluated model with costs=7450.53252712357
Evaluated model with costs=7450.496156909457
Evaluated model with costs=7446.667633679858
Evaluated model with costs=70280.27345322467
Evaluated model with costs=7737.1779338333245
Evaluated model with costs=7439.972055728105
Evaluated model with costs=7433.4142903363245
================================================================================
Time: 1265.9475449480015
Iteration: 29
Costs
deformation=10.9193595812676
attach=7422.494930755057
Total cost=7433.4142903363245
1e-10
Evaluated model with costs=7433.4142903363245
Evaluated model with costs=9261.01326099095
Evaluated model with costs=7428.6982195693645
Evaluated model with costs=7416.112777114258
Evaluated model with costs=15472.058530979191
Evaluated model with costs=7415.120779862922
Evaluated model with costs=7446.375315514024
Evaluated model with costs=7406.339367928998
Evaluated model with costs=214502.82215409592
Evaluated model with costs=7406.277551554057
Evaluated model with costs=7445.556550405464
Evaluated model with costs=7399.747342176832
Evaluated model with costs=7674.042795361172
Evaluated model with costs=7400.493794711572
Evaluated model with costs=7395.1793573393425
Evaluated model with costs=16576.820311438772
Evaluated model with costs=7644.763953742911
Evaluated model with costs=7399.1116444042855
Evaluated model with costs=7391.787868516347
Evaluated model with costs=8433.918302776903
Evaluated model with costs=7404.474559280675
Evaluated model with costs=7387.1182046593185
Evaluated model with costs=9322.522902269266
Evaluated model with costs=7440.802167444321
Evaluated model with costs=7381.9065761156535
================================================================================
Time: 1308.2925426620022
Iteration: 30
Costs
deformation=11.094578887433489
attach=7370.81199722822
Total cost=7381.9065761156535
1e-10
Evaluated model with costs=7381.9065761156535
Evaluated model with costs=7388.356877579407
Evaluated model with costs=7379.009906863607
Evaluated model with costs=7447.359462892457
Evaluated model with costs=7371.919573800917
Evaluated model with costs=7365.645795047073
Evaluated model with costs=7401.575354588209
Evaluated model with costs=7364.350878182682
Evaluated model with costs=7371.273723736949
Evaluated model with costs=7361.677048121081
Evaluated model with costs=7360.4194797354685
Evaluated model with costs=7359.05136695788
Evaluated model with costs=7360.5080644770405
Evaluated model with costs=7358.755055888413
Evaluated model with costs=7357.771515857558
Evaluated model with costs=7388.179693196344
Evaluated model with costs=7356.906029337364
Evaluated model with costs=7356.6016521121965
Evaluated model with costs=7405.209873121529
Evaluated model with costs=7356.028726208649
Evaluated model with costs=7355.396860773062
Evaluated model with costs=19878.277080106265
Evaluated model with costs=7404.329902683365
Evaluated model with costs=7354.516568857953
Evaluated model with costs=7353.924683497297
================================================================================
Time: 1350.3021442250028
Iteration: 31
Costs
deformation=11.222841514077382
attach=7342.70184198322
Total cost=7353.924683497297
1e-10
Evaluated model with costs=7353.924683497297
Evaluated model with costs=8576.744422693553
Evaluated model with costs=7365.009382083717
Evaluated model with costs=7353.09850313113
Evaluated model with costs=7447.157935460196
Evaluated model with costs=7352.903487722818
Evaluated model with costs=7379.169269778641
Evaluated model with costs=7352.1812163276745
Evaluated model with costs=7441.165806522063
Evaluated model with costs=7371.179132690709
Evaluated model with costs=7351.823001875811
Evaluated model with costs=7351.002097134075
Evaluated model with costs=7466.601840434938
Evaluated model with costs=7358.228911932514
Evaluated model with costs=7351.3645292882375
Evaluated model with costs=7350.186263335281
Evaluated model with costs=7372.144901907608
Evaluated model with costs=7387.215013306323
Evaluated model with costs=7350.970640809505
Evaluated model with costs=7349.950078894268
Evaluated model with costs=7955.851329473069
Evaluated model with costs=7349.939649198206
Evaluated model with costs=7353.447558234707
Evaluated model with costs=7349.899228469891
Evaluated model with costs=7599.721115240735
Evaluated model with costs=7353.335548858468
Evaluated model with costs=7349.794959930993
Evaluated model with costs=7349.721510057545
================================================================================
Time: 1397.4304207530004
Iteration: 32
Costs
deformation=11.231915266135283
attach=7338.489594791409
Total cost=7349.721510057545
1e-10
Evaluated model with costs=7349.721510057545
Evaluated model with costs=7431.3286542887745
Evaluated model with costs=7349.718570924517
Evaluated model with costs=7349.551700928015
Evaluated model with costs=7362.312641582082
Evaluated model with costs=7567.308072548272
Evaluated model with costs=7349.551421967938
Evaluated model with costs=7349.475611301116
Evaluated model with costs=7347.7902859937985
Evaluated model with costs=7346.875965792369
Evaluated model with costs=7345.32286943117
Evaluated model with costs=7337.318974691535
Evaluated model with costs=7315.859965760588
Evaluated model with costs=7244.781962847454
Evaluated model with costs=7195.9764827394
Evaluated model with costs=7175.113566848299
Evaluated model with costs=7169.2624553233
Evaluated model with costs=7168.24494174942
Evaluated model with costs=7162.0001750230185
Evaluated model with costs=7134.546119699719
Evaluated model with costs=7095.397602489797
Evaluated model with costs=7094.234901091875
Evaluated model with costs=7094.185684878637
Evaluated model with costs=7094.1770267046795
Evaluated model with costs=7094.1142716297445
================================================================================
Time: 1439.8097477240008
Iteration: 33
Costs
deformation=11.602359577835276
attach=7082.511912051909
Total cost=7094.1142716297445
1e-10
Evaluated model with costs=7094.1142716297445
Evaluated model with costs=7093.857765701278
Evaluated model with costs=7092.891898560272
Evaluated model with costs=7090.686580724138
Evaluated model with costs=7084.86596908833
Evaluated model with costs=7071.146277915243
Evaluated model with costs=7040.607567502391
Evaluated model with costs=6977.828547842378
Evaluated model with costs=6848.916335366943
Evaluated model with costs=6610.482224517169
Evaluated model with costs=6330.58637186829
Evaluated model with costs=6152.4571266524035
Evaluated model with costs=5998.874681820864
Evaluated model with costs=5975.3198743888715
Evaluated model with costs=6074.205747797848
Evaluated model with costs=5935.361240923594
Evaluated model with costs=5918.645922538064
Evaluated model with costs=5917.251081930057
Evaluated model with costs=5916.272093497611
Evaluated model with costs=5916.208318299667
Evaluated model with costs=5916.199234866162
Evaluated model with costs=5916.197258706147
================================================================================
Time: 1476.5677507390028
Iteration: 34
Costs
deformation=13.39728313652806
attach=5902.799975569619
Total cost=5916.197258706147
1e-10
Evaluated model with costs=5916.197258706147
Evaluated model with costs=5916.196156454178
Evaluated model with costs=5916.18997644286
Evaluated model with costs=5916.1756065800655
Evaluated model with costs=5916.104429404404
Evaluated model with costs=5915.9445171383695
Evaluated model with costs=5914.666164546127
Evaluated model with costs=5905.407844518342
Evaluated model with costs=5881.563054846885
Evaluated model with costs=5876.358250578397
Evaluated model with costs=5865.536922228197
Evaluated model with costs=5843.432893117732
Evaluated model with costs=5828.492824728217
Evaluated model with costs=5810.438953568614
Evaluated model with costs=5828.747651109107
Evaluated model with costs=5798.867123682484
Evaluated model with costs=5795.157302604559
Evaluated model with costs=5789.298020491784
Evaluated model with costs=5780.649638249002
Evaluated model with costs=5771.639820754353
Evaluated model with costs=5766.34436766552
Evaluated model with costs=5765.372353964438
Evaluated model with costs=5764.299924744151
Evaluated model with costs=5764.269328205179
Evaluated model with costs=5763.983948450927
Evaluated model with costs=5761.922251363685
================================================================================
Time: 1519.9129757170012
Iteration: 35
Costs
deformation=14.319188988597885
attach=5747.603062375088
Total cost=5761.922251363685
1e-10
Evaluated model with costs=5761.922251363685
Evaluated model with costs=5754.523200509156
Evaluated model with costs=5743.670057807967
Evaluated model with costs=5739.11174120492
Evaluated model with costs=5751.699535525947
Evaluated model with costs=5737.2690568329135
Evaluated model with costs=5736.643028808283
Evaluated model with costs=5736.474669353139
Evaluated model with costs=5736.415153850955
Evaluated model with costs=5736.269625855334
Evaluated model with costs=5736.215281467168
Evaluated model with costs=5736.196989263092
Evaluated model with costs=5736.196458544551
Evaluated model with costs=5736.195728246086
Evaluated model with costs=5736.192254950535
Evaluated model with costs=5736.184900172884
Evaluated model with costs=5736.130690349978
Evaluated model with costs=5735.877977410652
Evaluated model with costs=5735.066168834137
Evaluated model with costs=5733.4549407467075
Evaluated model with costs=5730.671297510698
Evaluated model with costs=5727.759774693957
Evaluated model with costs=5725.858519918005
Evaluated model with costs=5725.322588247093
================================================================================
Time: 1559.5063006520031
Iteration: 36
Costs
deformation=14.922959825546732
attach=5710.399628421546
Total cost=5725.322588247093
1e-10
Evaluated model with costs=5725.322588247093
Evaluated model with costs=5725.236141960591
Evaluated model with costs=5725.2205273834215
Evaluated model with costs=5725.211964454854
Evaluated model with costs=5725.208883090963
Evaluated model with costs=5724.940606335855
Evaluated model with costs=5724.308830616096
Evaluated model with costs=5720.0291084748815
Evaluated model with costs=5710.930062522299
Evaluated model with costs=5684.432245364411
Evaluated model with costs=5640.211256884639
Evaluated model with costs=6919.303929145323
Evaluated model with costs=5680.58592732859
Evaluated model with costs=5624.360945975732
Evaluated model with costs=5614.465955526539
Evaluated model with costs=5564.346318603825
Evaluated model with costs=5581.205845852465
Evaluated model with costs=5526.826570595162
Evaluated model with costs=5444.6574084309395
Evaluated model with costs=5541.770578551219
Evaluated model with costs=5377.484386842129
Evaluated model with costs=6064.681544010813
Evaluated model with costs=5317.8090825884265
Evaluated model with costs=5294.227279650818
Evaluated model with costs=5257.209577841452
================================================================================
Time: 1600.7495361200017
Iteration: 37
Costs
deformation=19.15770849454193
attach=5238.05186934691
Total cost=5257.209577841452
1e-10
Evaluated model with costs=5257.209577841452
Evaluated model with costs=5262.898295996999
Evaluated model with costs=5191.536506682132
Evaluated model with costs=5174.331965081349
Evaluated model with costs=5157.718019643682
Evaluated model with costs=5148.3517938044
Evaluated model with costs=5143.226654255378
Evaluated model with costs=5123.505818238842
Evaluated model with costs=4985.968195182967
Evaluated model with costs=4893.95264547432
Evaluated model with costs=4825.297450930822
Evaluated model with costs=4758.3035752418
Evaluated model with costs=4743.95967550714
Evaluated model with costs=4725.978428671742
Evaluated model with costs=4660.557306772038
Evaluated model with costs=4582.79794903854
Evaluated model with costs=4565.523901457229
Evaluated model with costs=4549.609042869082
Evaluated model with costs=4537.5524929776075
Evaluated model with costs=4528.793959425728
Evaluated model with costs=4521.820387138132
Evaluated model with costs=4516.975026548862
Evaluated model with costs=4511.534301801983
================================================================================
Time: 1638.9210350580033
Iteration: 38
Costs
deformation=26.562119940255712
attach=4484.972181861727
Total cost=4511.534301801983
1e-10
Evaluated model with costs=4511.534301801983
Evaluated model with costs=4519.857252292062
Evaluated model with costs=4504.072264546263
Evaluated model with costs=4496.37375228841
Evaluated model with costs=4489.297883995521
Evaluated model with costs=4484.769081736263
Evaluated model with costs=4484.560409385625
Evaluated model with costs=4478.99730937841
Evaluated model with costs=4472.934433000973
Evaluated model with costs=4459.724353133622
Evaluated model with costs=4452.504206752493
Evaluated model with costs=4458.183320270017
Evaluated model with costs=4445.162722065443
Evaluated model with costs=4459.466068283803
Evaluated model with costs=4440.731695749441
Evaluated model with costs=4438.786503501939
Evaluated model with costs=4439.429032674836
Evaluated model with costs=4428.113293570902
Evaluated model with costs=4419.176405417421
Evaluated model with costs=4414.41223288977
Evaluated model with costs=4404.751942571592
Evaluated model with costs=4397.791784840229
Evaluated model with costs=4390.931769746293
Evaluated model with costs=4381.291592199824
Evaluated model with costs=4386.300018351891
Evaluated model with costs=4375.234972438683
================================================================================
Time: 1681.8639997410028
Iteration: 39
Costs
deformation=23.943490381959116
attach=4351.291482056724
Total cost=4375.234972438683
1e-10
Evaluated model with costs=4375.234972438683
Evaluated model with costs=4368.248296959468
Evaluated model with costs=4359.8559005939305
Evaluated model with costs=4341.450162485294
Evaluated model with costs=4343.61007083875
Evaluated model with costs=4334.026311258592
Evaluated model with costs=4329.731047064237
Evaluated model with costs=4314.862229711925
Evaluated model with costs=4311.408636283985
Evaluated model with costs=4291.352299829885
Evaluated model with costs=4284.718458503536
Evaluated model with costs=4278.953272945933
Evaluated model with costs=4295.590544240457
Evaluated model with costs=4276.553477566663
Evaluated model with costs=4271.609958082279
Evaluated model with costs=4259.552979404654
Evaluated model with costs=4259.171200260096
Evaluated model with costs=4253.665450596041
Evaluated model with costs=4240.747704486208
Evaluated model with costs=4300.31495574437
Evaluated model with costs=4236.4410897111775
Evaluated model with costs=4228.4400959749355
Evaluated model with costs=4224.731802833267
Evaluated model with costs=4222.563811192773
Evaluated model with costs=4220.445334261888
================================================================================
Time: 1723.522406160002
Iteration: 40
Costs
deformation=22.026381269431166
attach=4198.418952992457
Total cost=4220.445334261888
1e-10
Evaluated model with costs=4220.445334261888
Evaluated model with costs=4217.472419306289
Evaluated model with costs=4214.713062192727
Evaluated model with costs=4212.9470863648485
Evaluated model with costs=4211.11597333222
Evaluated model with costs=4207.111263345616
Evaluated model with costs=4199.257733400728
Evaluated model with costs=4194.724700402663
Evaluated model with costs=4433.245886256907
Evaluated model with costs=4193.625762092534
Evaluated model with costs=4192.832128373542
Evaluated model with costs=4191.713021438655
Evaluated model with costs=4190.876321333108
Evaluated model with costs=4189.719241419528
Evaluated model with costs=4186.414589934868
Evaluated model with costs=4183.232776929933
Evaluated model with costs=4278.690096084667
Evaluated model with costs=4181.96731909268
Evaluated model with costs=4177.465133694553
Evaluated model with costs=4173.068351344608
Evaluated model with costs=4167.916946707104
Evaluated model with costs=4181.388656473248
Evaluated model with costs=4164.292872203614
Evaluated model with costs=4156.041499706217
Evaluated model with costs=4158.318522910657
Evaluated model with costs=4151.118758807045
================================================================================
Time: 1767.8362129840025
Iteration: 41
Costs
deformation=20.902545026323736
attach=4130.216213780722
Total cost=4151.118758807045
1e-10
Evaluated model with costs=4151.118758807045
Evaluated model with costs=4143.876310530678
Evaluated model with costs=4136.1213485412345
Evaluated model with costs=4154.8222811650485
Evaluated model with costs=4130.496578711049
Evaluated model with costs=4123.101140105838
Evaluated model with costs=4126.62708161796
Evaluated model with costs=4120.5102714136265
Evaluated model with costs=4122.377975719022
Evaluated model with costs=4117.099860636007
Evaluated model with costs=4110.162631931081
Evaluated model with costs=4105.875582416395
Evaluated model with costs=4100.20891356007
Evaluated model with costs=4101.467887415803
Evaluated model with costs=4099.344196454556
Evaluated model with costs=4098.695353608058
Evaluated model with costs=4096.761250751439
Evaluated model with costs=4093.9116342705042
Evaluated model with costs=4094.7907060339003
Evaluated model with costs=4093.605529328622
Evaluated model with costs=4092.00288288644
Evaluated model with costs=4091.576893698519
Evaluated model with costs=4091.2310388943347
Evaluated model with costs=4090.893106728984
Evaluated model with costs=4089.7555332530364
================================================================================
Time: 1810.2269260230023
Iteration: 42
Costs
deformation=23.045319152886876
attach=4066.7102141001496
Total cost=4089.7555332530364
1e-10
Evaluated model with costs=4089.7555332530364
Evaluated model with costs=4088.7263021029994
Evaluated model with costs=4086.539495029044
Evaluated model with costs=4085.0573677221573
Evaluated model with costs=4083.0527376750238
Evaluated model with costs=4076.8833653639326
Evaluated model with costs=4281.725713542191
Evaluated model with costs=4073.2511039528226
Evaluated model with costs=4068.671319293412
Evaluated model with costs=4065.4394229957757
Evaluated model with costs=4062.4605527961126
Evaluated model with costs=4060.7629163990637
Evaluated model with costs=4067.2614626594186
Evaluated model with costs=4059.843351175389
Evaluated model with costs=4060.6532064845314
Evaluated model with costs=4058.8729420421064
Evaluated model with costs=4062.812941777659
Evaluated model with costs=4058.797317285872
Evaluated model with costs=4057.9942978529416
Evaluated model with costs=4057.6440071184356
Evaluated model with costs=4057.493614887458
Evaluated model with costs=4057.2154937229798
Evaluated model with costs=4056.8304657505073
Evaluated model with costs=4056.4008546464115
Evaluated model with costs=4056.21978086556
================================================================================
Time: 1852.4939283480016
Iteration: 43
Costs
deformation=23.372684518040717
attach=4032.8470963475193
Total cost=4056.21978086556
1e-10
Evaluated model with costs=4056.21978086556
Evaluated model with costs=4055.6169958938003
Evaluated model with costs=4054.2839316474956
Evaluated model with costs=4053.0313272907274
Evaluated model with costs=4052.0882083402767
Evaluated model with costs=4051.3243083982625
Evaluated model with costs=4050.1730324843575
Evaluated model with costs=4049.557966564527
Evaluated model with costs=4049.0862841953085
Evaluated model with costs=4048.785395595045
Evaluated model with costs=4048.0951952149067
Evaluated model with costs=4047.303538257288
Evaluated model with costs=4046.434026629855
Evaluated model with costs=4044.0554549904523
Evaluated model with costs=4045.228688343675
Evaluated model with costs=4043.0306606333065
Evaluated model with costs=4043.402213039152
Evaluated model with costs=4042.8645176212194
Evaluated model with costs=4045.2102552177626
Evaluated model with costs=4042.501013449498
Evaluated model with costs=4042.4436630409255
Evaluated model with costs=4042.1219868694748
Evaluated model with costs=4041.863856227074
Evaluated model with costs=4043.104381504573
Evaluated model with costs=4041.876322667519
Evaluated model with costs=4041.829241508807
================================================================================
Time: 1900.227179452002
Iteration: 44
Costs
deformation=24.594913309703614
attach=4017.2343281991034
Total cost=4041.829241508807
1e-10
Evaluated model with costs=4041.829241508807
Evaluated model with costs=4041.5953491447403
Evaluated model with costs=4041.637616026106
Evaluated model with costs=4041.366714287732
Evaluated model with costs=4040.73692357468
Evaluated model with costs=4047.8325432047704
Evaluated model with costs=4037.283813747509
Evaluated model with costs=4037.5433728250555
Evaluated model with costs=4036.7200548613273
Evaluated model with costs=4036.759981699091
Evaluated model with costs=4035.998771234952
Evaluated model with costs=4035.259001060284
Evaluated model with costs=4033.709467636966
Evaluated model with costs=4031.20318820753
Evaluated model with costs=4030.6827828073547
Evaluated model with costs=26582.10343851279
Evaluated model with costs=4603.28620079557
Evaluated model with costs=4028.223415568954
Evaluated model with costs=4024.7974129275062
Evaluated model with costs=4028.1373440042566
Evaluated model with costs=4022.8676350478518
Evaluated model with costs=4022.7535904738374
Evaluated model with costs=4022.2047242955414
Evaluated model with costs=4023.35395480646
Evaluated model with costs=4021.390246268541
================================================================================
Time: 1946.4969224570013
Iteration: 45
Costs
deformation=26.100942338944378
attach=3995.2893039295964
Total cost=4021.390246268541
1e-10
Evaluated model with costs=4021.390246268541
Evaluated model with costs=4019.052488494561
Evaluated model with costs=4035.4569951169346
Evaluated model with costs=4017.626381351198
Evaluated model with costs=4015.3267959475083
Evaluated model with costs=4014.7979614017736
Evaluated model with costs=4014.2319911800396
Evaluated model with costs=4012.8506524684094
Evaluated model with costs=4011.0566400448647
Evaluated model with costs=4016.616260067067
Evaluated model with costs=4009.9013090978096
Evaluated model with costs=4007.8542230426137
Evaluated model with costs=4005.393132065551
Evaluated model with costs=4049.795078914982
Evaluated model with costs=4004.749396192207
Evaluated model with costs=4002.086387040946
Evaluated model with costs=4000.483212356007
Evaluated model with costs=3999.155616193734
Evaluated model with costs=4000.205912806618
Evaluated model with costs=3997.3928914735175
Evaluated model with costs=4004.4342475933963
Evaluated model with costs=3996.083113575896
Evaluated model with costs=3995.2565320318895
Evaluated model with costs=3994.0402597194784
Evaluated model with costs=3993.923887674637
================================================================================
Time: 1990.227481126003
Iteration: 46
Costs
deformation=27.539320296057987
attach=3966.384567378579
Total cost=3993.923887674637
1e-10
Evaluated model with costs=3993.923887674637
Evaluated model with costs=3992.9186587444133
Evaluated model with costs=3992.3368192566845
Evaluated model with costs=3991.6488587901003
Evaluated model with costs=3993.647075699416
Evaluated model with costs=3991.0228036620383
Evaluated model with costs=3989.9836525402757
Evaluated model with costs=3990.2774017904467
Evaluated model with costs=3989.771704298861
Evaluated model with costs=3989.711034591777
Evaluated model with costs=3989.6845784378506
Evaluated model with costs=3989.6587581291587
Evaluated model with costs=3989.60541914972
Evaluated model with costs=3989.5060737316335
Evaluated model with costs=3989.1834810093637
Evaluated model with costs=3988.209344183822
Evaluated model with costs=3986.544100788192
Evaluated model with costs=3984.3727748082956
Evaluated model with costs=3983.94549386294
Evaluated model with costs=3984.2827194383653
Evaluated model with costs=3983.768468600734
Evaluated model with costs=3983.524819928056
Evaluated model with costs=3983.3093906981544
Evaluated model with costs=3982.859617609388
================================================================================
Time: 2031.3035688970012
Iteration: 47
Costs
deformation=27.225069476923885
attach=3955.634548132464
Total cost=3982.859617609388
1e-10
Evaluated model with costs=3982.859617609388
Evaluated model with costs=3982.6570850502817
Evaluated model with costs=3982.5712480154652
Evaluated model with costs=3982.5088414475226
Evaluated model with costs=3982.462756815127
Evaluated model with costs=3982.3944520266264
Evaluated model with costs=3982.310426083183
Evaluated model with costs=3982.096717207498
Evaluated model with costs=3981.764524765543
Evaluated model with costs=3981.722728310687
Evaluated model with costs=3981.3701538298264
Evaluated model with costs=3981.1007535322647
Evaluated model with costs=3980.9242899294363
Evaluated model with costs=3980.37099015341
Evaluated model with costs=3980.384260046565
Evaluated model with costs=3980.2549855743755
Evaluated model with costs=3980.1768649566684
Evaluated model with costs=3980.039179313292
Evaluated model with costs=3979.950523217656
Evaluated model with costs=3979.708694710771
Evaluated model with costs=3979.39480610619
Evaluated model with costs=3979.9194321067193
Evaluated model with costs=3979.263377092278
Evaluated model with costs=3978.9312463038823
Evaluated model with costs=3978.8188830925174
================================================================================
Time: 2076.4124346030003
Iteration: 48
Costs
deformation=26.466429148331624
attach=3952.3524539441855
Total cost=3978.8188830925174
1e-10
Evaluated model with costs=3978.8188830925174
Evaluated model with costs=3978.8170740209193
Evaluated model with costs=3978.602836411073
Evaluated model with costs=3978.4431071279882
Evaluated model with costs=3978.326927208141
Evaluated model with costs=3978.5379108047764
Evaluated model with costs=3978.1821126567065
Evaluated model with costs=3978.056189248752
Evaluated model with costs=3977.717690148747
Evaluated model with costs=3978.119033201126
Evaluated model with costs=3977.6115554575026
Evaluated model with costs=3977.311173511011
Evaluated model with costs=3976.8881648573943
Evaluated model with costs=3976.6695383492806
Evaluated model with costs=3975.8632644394797
Evaluated model with costs=3975.171948352502
Evaluated model with costs=3973.6805226083125
Evaluated model with costs=3995.5085874243505
Evaluated model with costs=3973.4198828261724
Evaluated model with costs=3996.5796474538697
Evaluated model with costs=3971.9110350138644
Evaluated model with costs=3970.7103449273586
Evaluated model with costs=4807.981350186249
Evaluated model with costs=3970.3698282542655
Evaluated model with costs=3969.3729361989963
================================================================================
Time: 2120.8096821920008
Iteration: 49
Costs
deformation=27.49610875822769
attach=3941.8768274407685
Total cost=3969.3729361989963
================================================================================
Optimisation process exited with message: Total number of iterations reached.
Final cost=3969.3729361989963
Model evaluation count=1239
Time elapsed = 2120.810018872002
Compute optimized deformation trajectory.
intermediates = {}
with torch.autograd.no_grad():
deformed = model.compute_deformed(shoot_solver, shoot_it, intermediates=intermediates)
deformed_shape = deformed[0][0]
deformed_dots = deformed[1][0]
deformed_growth = intermediates['states'][-1][3].gd[0]
deformed_growth_rot = intermediates['states'][-1][3].gd[1]
global_translation_controls = [control[2] for control in intermediates['controls']]
growth_controls = [control[3] for control in intermediates['controls']]
Plot results.
plt.subplot(1, 3, 1)
plt.title("Source")
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), '-', color='black')
plt.plot(dots_source[:, 0].numpy(), dots_source[:, 1].numpy(), '.', color='black')
plt.axis('equal')
plt.subplot(1, 3, 2)
plt.title("Deformed source")
plt.plot(deformed_shape[:, 0], deformed_shape[:, 1], '-', color='blue')
plt.plot(deformed_dots[:, 0], deformed_dots[:, 1], '.', color='blue')
plt.axis('equal')
plt.subplot(1, 3, 3)
plt.title("Deformed source and target")
plt.plot(shape_target[:, 0].numpy(), shape_target[:, 1].numpy(), '-', color='red')
plt.plot(dots_target[:, 0].numpy(), dots_target[:, 1].numpy(), '.', color='red')
plt.plot(deformed_shape[:, 0], deformed_shape[:, 1], '-', color='blue')
plt.plot(deformed_dots[:, 0], deformed_dots[:, 1], '.', color='blue')
plt.axis('equal')
plt.show()
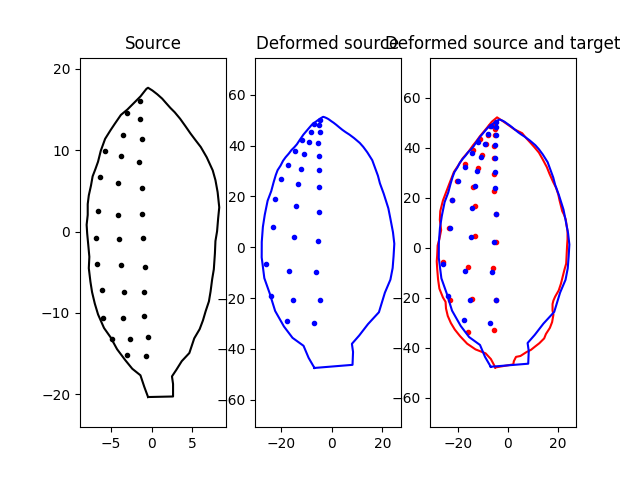
Evaluate estimated growth model tensor.
learned_abcd = abcd.detach()
learned_C = pol(model.init_manifold[3].gd[0].detach(),
learned_abcd[0].unsqueeze(1),
learned_abcd[1].unsqueeze(1),
learned_abcd[2].unsqueeze(1),
learned_abcd[3].unsqueeze(1)).transpose(0, 1).unsqueeze(2).detach()
print("Estimated growth model tensor parameters:\n {}".format(learned_abcd))
Out:
Estimated growth model tensor parameters:
tensor([[-9.6762e-01, -9.3946e-01],
[ 4.2391e-02, 6.4030e-02],
[ 9.6198e-04, 2.1804e-03],
[ 7.9189e-05, 2.3509e-05]])
Plot estimated growth factor.
ax = plt.subplot()
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), '-')
imodal.Utilities.plot_C_ellipses(ax, points_growth, learned_C, R=deformed_growth_rot, scale=1.)
plt.axis(aabb_source.squared().totuple())
plt.axis('equal')
plt.show()
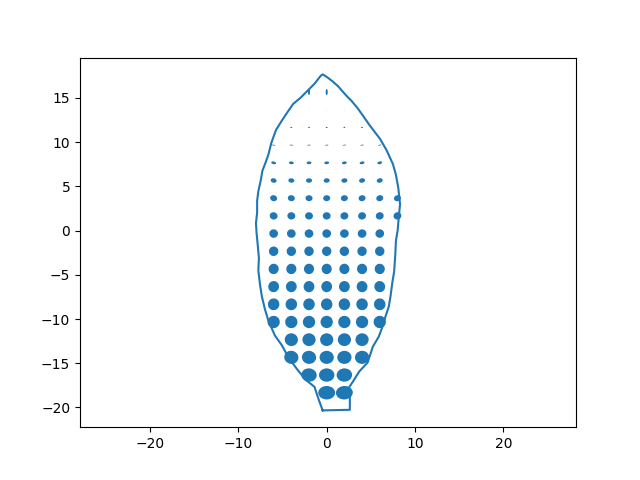
Recompute the learned deformation trajectory this time with the grid deformation to visualize growth.
# We extract the modules of the models and fill the right manifolds.
modules = imodal.DeformationModules.CompoundModule(copy.copy(model.modules))
modules.manifold.fill(model.init_manifold.clone())
silent_shape = copy.copy(modules[0])
silent_dots = copy.copy(modules[1])
global_translation = copy.copy(modules[2])
growth = copy.copy(modules[3])
# Define the deformation grid.
square_size = 1.
growth_grid_resolution = [math.floor(aabb_source.width/square_size),
math.floor(aabb_source.height/square_size)]
deformation_grid = imodal.DeformationModules.DeformationGrid(aabb_source, growth_grid_resolution)
# We construct the controls we will give while shooting.
controls = [[torch.tensor([]), torch.tensor([]), torch.tensor([]), global_translation_control, growth_control] for growth_control, global_translation_control in zip(growth_controls, global_translation_controls)]
# Reshoot.
intermediates_growth = {}
with torch.autograd.no_grad():
imodal.HamiltonianDynamic.shoot(imodal.HamiltonianDynamic.Hamiltonian([silent_shape, silent_dots, deformation_grid, global_translation, growth]), shoot_solver, shoot_it, controls=controls, intermediates=intermediates_growth)
# Store final deformation.
shoot_deformed_shape = silent_shape.manifold.gd.detach()
shoot_deformed_dots = silent_dots.manifold.gd.detach()
shoot_deformed_grid = deformation_grid.togrid()
Plot the deformation grid.
ax = plt.subplot()
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), '--', color='black')
plt.plot(dots_source[:, 0].numpy(), dots_source[:, 1].numpy(), '.', color='black')
plt.plot(shape_target[:, 0].numpy(), shape_target[:, 1].numpy(), '.-', color='red')
plt.plot(dots_target[:, 0].numpy(), dots_target[:, 1].numpy(), '.', color='black')
plt.plot(shoot_deformed_shape[:, 0].numpy(), shoot_deformed_shape[:, 1].numpy())
plt.plot(shoot_deformed_dots[:, 0].numpy(), shoot_deformed_dots[:, 1].numpy(), '.')
imodal.Utilities.plot_grid(ax, shoot_deformed_grid[0], shoot_deformed_grid[1], color='xkcd:light blue', lw=0.4)
plt.axis('equal')
plt.show()
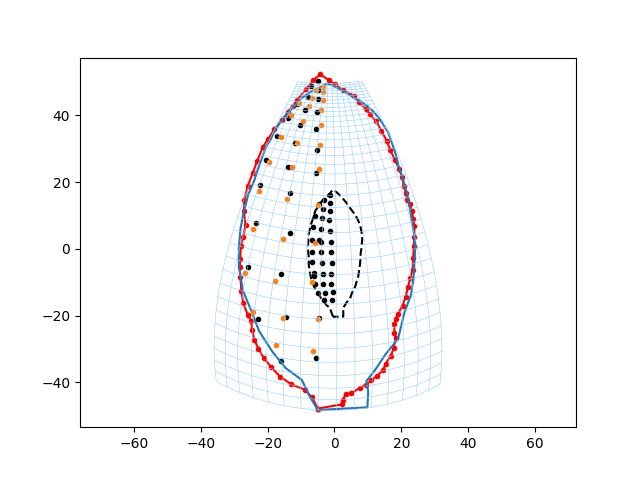
Perform curve registration using the previously learned growth factor¶
Redefine deformation modules.
global_translation = imodal.DeformationModules.GlobalTranslation(2)
growth = imodal.DeformationModules.ImplicitModule1(2, points_growth.shape[0], scale_growth, learned_C, coeff=coeff_growth, nu=nu, gd=(points_growth, rot_growth))
small_scale_translation = imodal.DeformationModules.ImplicitModule0(2, shape_source.shape[0], scale_small, coeff=coeff_small, nu=nu, gd=shape_source)
Redefine deformables and registration model.
deformable_shape_source = imodal.Models.DeformablePoints(shape_source)
deformable_shape_target = imodal.Models.DeformablePoints(shape_target)
refit_model = imodal.Models.RegistrationModel([deformable_shape_source],
[global_translation, growth, small_scale_translation],
[imodal.Attachment.VarifoldAttachment(2, [20., 120.], backend='torch')],
lam=10.)
Fitting using Torch LBFGS optimizer.
shoot_solver = 'euler'
shoot_it = 10
costs = {}
fitter = imodal.Models.Fitter(refit_model, optimizer='torch_lbfgs')
fitter.fit([deformable_shape_target], 100, costs=costs, options={'shoot_solver': shoot_solver, 'shoot_it': shoot_it, 'line_search_fn': 'strong_wolfe'})
Out:
Starting optimization with method torch LBFGS, using solver euler with 10 iterations.
Initial cost={'deformation': 0.0, 'attach': 182832.08368559513}
1e-10
Evaluated model with costs=182832.08368559513
Evaluated model with costs=20174.04435216034
Evaluated model with costs=33943.34108708341
Evaluated model with costs=6150.628038675951
Evaluated model with costs=6145.754441832058
Evaluated model with costs=6143.847364946738
Evaluated model with costs=6128.029246565492
Evaluated model with costs=6097.57605540293
Evaluated model with costs=6010.300941838441
Evaluated model with costs=5809.682626864071
Evaluated model with costs=5356.529649396763
Evaluated model with costs=4447.622200751545
Evaluated model with costs=3037.6296906924613
Evaluated model with costs=2802.7873309082006
Evaluated model with costs=2757.4671725793924
Evaluated model with costs=2752.1139628597853
Evaluated model with costs=2750.659901377933
Evaluated model with costs=2749.878933823906
Evaluated model with costs=2745.7486388714015
Evaluated model with costs=2737.133506337158
Evaluated model with costs=2712.9819122719678
Evaluated model with costs=2655.3786306858055
================================================================================
Time: 37.63140680700235
Iteration: 0
Costs
deformation=18.12314293124996
attach=2637.2554877545554
Total cost=2655.3786306858055
1e-10
Evaluated model with costs=2655.3786306858055
Evaluated model with costs=2523.79329521841
Evaluated model with costs=2278.6068304505557
Evaluated model with costs=1937.445461715731
Evaluated model with costs=1630.8700947489901
Evaluated model with costs=1542.7880718702681
Evaluated model with costs=1528.976198922468
Evaluated model with costs=1527.908514802849
Evaluated model with costs=1526.5216083531434
Evaluated model with costs=1526.4640849656796
Evaluated model with costs=1525.9887466780285
Evaluated model with costs=1525.1047921089812
Evaluated model with costs=1522.297793846651
Evaluated model with costs=1515.3041970620832
Evaluated model with costs=1496.3397688332966
Evaluated model with costs=1451.3722055507167
Evaluated model with costs=1375.00478777983
Evaluated model with costs=1267.1337770918374
Evaluated model with costs=1058.796828304833
Evaluated model with costs=955.1576383441195
Evaluated model with costs=863.641663999181
================================================================================
Time: 72.49851809200118
Iteration: 1
Costs
deformation=27.782024683722458
attach=835.8596393154585
Total cost=863.641663999181
1e-10
Evaluated model with costs=863.641663999181
Evaluated model with costs=3118.1472270569634
Evaluated model with costs=855.0615193373688
Evaluated model with costs=847.8854597872304
Evaluated model with costs=806.3241821284313
Evaluated model with costs=772.5237421897814
Evaluated model with costs=762.4298840426645
Evaluated model with costs=756.9709522395926
Evaluated model with costs=748.575843586474
Evaluated model with costs=741.0533316171741
Evaluated model with costs=740.3966171767493
Evaluated model with costs=737.7070248139679
Evaluated model with costs=736.4831769768305
Evaluated model with costs=734.6133204524366
Evaluated model with costs=733.5139079658289
Evaluated model with costs=731.6858572599643
Evaluated model with costs=727.856785742327
Evaluated model with costs=717.8177919878551
Evaluated model with costs=694.8772204423972
Evaluated model with costs=655.2919450604821
Evaluated model with costs=6819.311909448437
Evaluated model with costs=657.5813361633983
Evaluated model with costs=651.8646493106594
Evaluated model with costs=639.6794491790391
Evaluated model with costs=629.3580734145015
================================================================================
Time: 116.61448635700071
Iteration: 2
Costs
deformation=30.815063037067315
attach=598.5430103774343
Total cost=629.3580734145015
1e-10
Evaluated model with costs=629.3580734145015
Evaluated model with costs=598.7252843208826
Evaluated model with costs=586.6827804379141
Evaluated model with costs=579.8780473959728
Evaluated model with costs=542.490846789143
Evaluated model with costs=497.9710423150559
Evaluated model with costs=594.3669961673824
Evaluated model with costs=468.16742655444966
Evaluated model with costs=664.7973482966252
Evaluated model with costs=430.83633829028116
Evaluated model with costs=393.19788481470744
Evaluated model with costs=393.1581842079589
Evaluated model with costs=384.8073587916333
Evaluated model with costs=384.50278067093876
Evaluated model with costs=384.06564091048807
Evaluated model with costs=383.81295832730893
Evaluated model with costs=383.24346081469787
Evaluated model with costs=381.9529783375957
Evaluated model with costs=379.9569867952904
Evaluated model with costs=377.8344608718613
Evaluated model with costs=376.6098695559912
Evaluated model with costs=376.4167866836551
Evaluated model with costs=376.20859145119596
Evaluated model with costs=376.03942593448505
Evaluated model with costs=375.4918932771333
================================================================================
Time: 159.1889666419993
Iteration: 3
Costs
deformation=29.28999065635513
attach=346.2019026207781
Total cost=375.4918932771333
1e-10
Evaluated model with costs=375.4918932771333
Evaluated model with costs=374.83583587726247
Evaluated model with costs=372.45235110530996
Evaluated model with costs=367.070324490859
Evaluated model with costs=354.7852262662803
Evaluated model with costs=344.0063352553652
Evaluated model with costs=341.8579814755492
Evaluated model with costs=341.50921431123163
Evaluated model with costs=341.39854659756713
Evaluated model with costs=341.29190751354736
Evaluated model with costs=341.1748482490152
Evaluated model with costs=340.9572349946627
Evaluated model with costs=340.6441273065815
Evaluated model with costs=339.97854604256986
Evaluated model with costs=338.5018184409801
Evaluated model with costs=334.86788445831763
Evaluated model with costs=325.90256935002014
Evaluated model with costs=298.5261786969533
Evaluated model with costs=264.9843376882291
Evaluated model with costs=295.20121571630125
Evaluated model with costs=242.39189068647812
Evaluated model with costs=229.60373176739412
================================================================================
Time: 196.35271483500037
Iteration: 4
Costs
deformation=31.329017755716983
attach=198.27471401167713
Total cost=229.60373176739412
1e-10
Evaluated model with costs=229.60373176739412
Evaluated model with costs=224.95782885032196
Evaluated model with costs=218.5513131334383
Evaluated model with costs=211.66410166687618
Evaluated model with costs=202.53770660739673
Evaluated model with costs=198.39121497065412
Evaluated model with costs=203.19778642667936
Evaluated model with costs=196.92936022203048
Evaluated model with costs=196.62932641562804
Evaluated model with costs=196.3381255980117
Evaluated model with costs=196.16473322241703
Evaluated model with costs=196.09202752451145
Evaluated model with costs=196.07489901923313
Evaluated model with costs=196.06547645425786
Evaluated model with costs=196.02687661139274
Evaluated model with costs=195.8350723915612
Evaluated model with costs=195.51168914546525
Evaluated model with costs=194.51389530488782
Evaluated model with costs=191.9274046284364
Evaluated model with costs=186.56093245354845
Evaluated model with costs=177.52224098998516
Evaluated model with costs=168.94708414172385
================================================================================
Time: 233.39629764200072
Iteration: 5
Costs
deformation=33.53636928513482
attach=135.41071485658904
Total cost=168.94708414172385
1e-10
Evaluated model with costs=168.94708414172385
Evaluated model with costs=173.95290440491038
Evaluated model with costs=167.0323925748583
Evaluated model with costs=163.6162709457738
Evaluated model with costs=162.66666059533395
Evaluated model with costs=160.21703215411418
Evaluated model with costs=159.04047646636815
Evaluated model with costs=158.75214000954236
Evaluated model with costs=158.55692611657156
Evaluated model with costs=158.5008636799938
Evaluated model with costs=158.48266422270927
Evaluated model with costs=158.4775736458232
Evaluated model with costs=158.4622278797234
Evaluated model with costs=158.43829408902053
Evaluated model with costs=158.36717472680857
Evaluated model with costs=158.1946931584945
Evaluated model with costs=157.7400491154151
Evaluated model with costs=156.53971379999007
Evaluated model with costs=153.72447212104782
Evaluated model with costs=147.64966048074498
Evaluated model with costs=141.04275451829074
Evaluated model with costs=233.69281786214128
Evaluated model with costs=139.92034796012695
================================================================================
Time: 273.11520337000184
Iteration: 6
Costs
deformation=32.960445377014835
attach=106.95990258311213
Total cost=139.92034796012695
1e-10
Evaluated model with costs=139.92034796012695
Evaluated model with costs=145.31051581105893
Evaluated model with costs=136.67336542280538
Evaluated model with costs=136.5222344603863
Evaluated model with costs=136.03672002611276
Evaluated model with costs=135.94396204095483
Evaluated model with costs=135.83115668254155
Evaluated model with costs=135.61272999830632
Evaluated model with costs=135.305205948766
Evaluated model with costs=134.92409098134124
Evaluated model with costs=134.53383994455558
Evaluated model with costs=134.33202108326768
Evaluated model with costs=134.5298524908928
Evaluated model with costs=134.28255133362154
Evaluated model with costs=134.24083880592391
Evaluated model with costs=134.20962328727157
Evaluated model with costs=134.15054807052957
Evaluated model with costs=133.99575228658642
Evaluated model with costs=133.579879953641
Evaluated model with costs=132.46850712841442
Evaluated model with costs=129.83504327112922
Evaluated model with costs=513.8355156123964
Evaluated model with costs=129.56137180399094
Evaluated model with costs=128.43610852660197
Evaluated model with costs=125.8741456925138
================================================================================
Time: 316.7002534359999
Iteration: 7
Costs
deformation=31.680289259174273
attach=94.19385643333953
Total cost=125.8741456925138
1e-10
Evaluated model with costs=125.8741456925138
Evaluated model with costs=118.69374839338035
Evaluated model with costs=115.35255849023011
Evaluated model with costs=107.0079475123517
Evaluated model with costs=106.23925509061652
Evaluated model with costs=104.0442682454434
Evaluated model with costs=103.77876847437939
Evaluated model with costs=103.73733064991298
Evaluated model with costs=103.7104430673175
Evaluated model with costs=103.53050149321456
Evaluated model with costs=103.28801255626779
Evaluated model with costs=102.67737794530358
Evaluated model with costs=101.77031217327817
Evaluated model with costs=99.9942235380581
Evaluated model with costs=97.97603364018222
Evaluated model with costs=101.29425762065499
Evaluated model with costs=97.49479047909608
Evaluated model with costs=95.93159828173741
Evaluated model with costs=95.19980484394036
Evaluated model with costs=98.65355427393297
Evaluated model with costs=94.232119749802
Evaluated model with costs=93.69853600245918
Evaluated model with costs=93.13586621257848
================================================================================
Time: 356.5170801910026
Iteration: 8
Costs
deformation=29.6302083009504
attach=63.50565791162808
Total cost=93.13586621257848
1e-10
Evaluated model with costs=93.13586621257848
Evaluated model with costs=97.48681479075321
Evaluated model with costs=92.7353961334247
Evaluated model with costs=92.43078541145555
Evaluated model with costs=91.96840630368621
Evaluated model with costs=91.75086743112172
Evaluated model with costs=103.47897545979166
Evaluated model with costs=91.6977601710223
Evaluated model with costs=91.65469152226827
Evaluated model with costs=91.50462850131748
Evaluated model with costs=91.36953643397308
Evaluated model with costs=91.3789589376147
Evaluated model with costs=91.32423647537078
Evaluated model with costs=91.2966165530616
Evaluated model with costs=91.26256994833192
Evaluated model with costs=91.26053485628447
Evaluated model with costs=91.2560399577361
Evaluated model with costs=91.25207386780107
Evaluated model with costs=91.24978330855326
Evaluated model with costs=91.24832580745704
Evaluated model with costs=91.2449255663816
Evaluated model with costs=91.23707430927485
Evaluated model with costs=91.21643063932844
Evaluated model with costs=91.20557377224739
Evaluated model with costs=91.19008443773805
================================================================================
Time: 397.3713262570018
Iteration: 9
Costs
deformation=30.023131692622194
attach=61.16695274511585
Total cost=91.19008443773805
1e-10
Evaluated model with costs=91.19008443773805
Evaluated model with costs=91.09549222283336
Evaluated model with costs=90.91918692843808
Evaluated model with costs=94.51715670027825
Evaluated model with costs=90.77177473981756
Evaluated model with costs=95.2907782277917
Evaluated model with costs=90.28775155450126
Evaluated model with costs=88.61712069346267
Evaluated model with costs=252.06877445950687
Evaluated model with costs=88.9718994918776
Evaluated model with costs=87.5948863998912
Evaluated model with costs=87.27677372704677
Evaluated model with costs=85.42224170796806
Evaluated model with costs=83.90996450482609
Evaluated model with costs=81.42389103256252
Evaluated model with costs=83.57438761033401
Evaluated model with costs=80.14370600117732
Evaluated model with costs=78.88231467969878
Evaluated model with costs=77.9881193824188
Evaluated model with costs=77.32994651169216
Evaluated model with costs=78.89792144392267
Evaluated model with costs=77.1454681028098
Evaluated model with costs=77.77886463833774
Evaluated model with costs=76.97114340709679
Evaluated model with costs=76.7399022430994
================================================================================
Time: 438.9147459039996
Iteration: 10
Costs
deformation=30.50610245245601
attach=46.23379979064339
Total cost=76.7399022430994
1e-10
Evaluated model with costs=76.7399022430994
Evaluated model with costs=76.65608770743118
Evaluated model with costs=76.59806403918567
Evaluated model with costs=76.43741345517083
Evaluated model with costs=76.26891545959114
Evaluated model with costs=75.92827044632253
Evaluated model with costs=88.02092973805557
Evaluated model with costs=75.902946799411
Evaluated model with costs=75.42811504575992
Evaluated model with costs=75.31965854407937
Evaluated model with costs=74.78429839327072
Evaluated model with costs=74.54002440997986
Evaluated model with costs=74.18672266005399
Evaluated model with costs=73.73274701962569
Evaluated model with costs=75.43951405358716
Evaluated model with costs=73.47874496276847
Evaluated model with costs=73.30047824364112
Evaluated model with costs=73.18780330815216
Evaluated model with costs=73.01099269088988
Evaluated model with costs=72.95292829001058
Evaluated model with costs=72.86323718628323
Evaluated model with costs=72.77465225148417
Evaluated model with costs=72.71841628357923
================================================================================
Time: 481.449271933001
Iteration: 11
Costs
deformation=31.528576442115977
attach=41.189839841463254
Total cost=72.71841628357923
1e-10
Evaluated model with costs=72.71841628357923
Evaluated model with costs=72.6853810672484
Evaluated model with costs=72.65802965021156
Evaluated model with costs=72.64508494845452
Evaluated model with costs=72.64223790790872
Evaluated model with costs=72.64135349655238
Evaluated model with costs=72.6405022009919
Evaluated model with costs=72.63526477617535
Evaluated model with costs=72.63551791544091
Evaluated model with costs=72.61329431025659
Evaluated model with costs=72.59411135830263
Evaluated model with costs=72.4111025373988
Evaluated model with costs=72.14267645389023
Evaluated model with costs=71.69730242282218
Evaluated model with costs=71.48858453007641
Evaluated model with costs=71.07357263688775
Evaluated model with costs=70.44384810694602
Evaluated model with costs=70.64561449723239
Evaluated model with costs=70.36297001307898
Evaluated model with costs=74.87954010824264
Evaluated model with costs=70.30425810021616
Evaluated model with costs=70.20104311039313
Evaluated model with costs=70.19896001134722
Evaluated model with costs=70.13864471704004
Evaluated model with costs=70.06855246517704
================================================================================
Time: 521.5691081879995
Iteration: 12
Costs
deformation=31.54376553212829
attach=38.524786933048745
Total cost=70.06855246517704
1e-10
Evaluated model with costs=70.06855246517704
Evaluated model with costs=69.86235887345248
Evaluated model with costs=69.7741616306501
Evaluated model with costs=69.45556990192127
Evaluated model with costs=69.65715848997064
Evaluated model with costs=69.31105888420316
Evaluated model with costs=68.99307704805989
Evaluated model with costs=68.87160303716216
Evaluated model with costs=68.95654062589443
Evaluated model with costs=68.75537728240225
Evaluated model with costs=68.71703113679106
Evaluated model with costs=68.6717360292717
Evaluated model with costs=68.65163964504049
Evaluated model with costs=68.62792737188101
Evaluated model with costs=68.58302156762934
Evaluated model with costs=68.44757334980335
Evaluated model with costs=73.69371903165595
Evaluated model with costs=68.38293014995772
Evaluated model with costs=68.05688960483742
Evaluated model with costs=68.18516177974615
Evaluated model with costs=67.53461714708149
Evaluated model with costs=66.82517979403927
Evaluated model with costs=66.81200758891995
Evaluated model with costs=66.53990577370755
Evaluated model with costs=66.46324538132919
================================================================================
Time: 564.4461501330006
Iteration: 13
Costs
deformation=29.753514301756663
attach=36.70973107957252
Total cost=66.46324538132919
1e-10
Evaluated model with costs=66.46324538132919
Evaluated model with costs=66.40845832686304
Evaluated model with costs=66.31575903895218
Evaluated model with costs=66.2641547311559
Evaluated model with costs=66.12528276062139
Evaluated model with costs=65.35892721266603
Evaluated model with costs=64.97572790797678
Evaluated model with costs=72.79633539190074
Evaluated model with costs=64.69770727850405
Evaluated model with costs=64.37688310353964
Evaluated model with costs=64.18073626337781
Evaluated model with costs=64.04014020399092
Evaluated model with costs=63.62790627763472
Evaluated model with costs=63.484310349099886
Evaluated model with costs=63.407475646713245
Evaluated model with costs=63.132677475818724
Evaluated model with costs=62.911384056424886
Evaluated model with costs=63.10263069664889
Evaluated model with costs=62.78177623967471
Evaluated model with costs=62.627205603761865
Evaluated model with costs=62.43739664765853
Evaluated model with costs=62.620932490029375
Evaluated model with costs=62.372443766138545
Evaluated model with costs=62.290438168409054
Evaluated model with costs=62.1778250190577
================================================================================
Time: 608.4027406910027
Iteration: 14
Costs
deformation=30.24824896557714
attach=31.92957605348056
Total cost=62.1778250190577
1e-10
Evaluated model with costs=62.1778250190577
Evaluated model with costs=62.218758658249484
Evaluated model with costs=62.14313651929439
Evaluated model with costs=62.08734160807512
Evaluated model with costs=62.02191863660933
Evaluated model with costs=61.981362132200935
Evaluated model with costs=61.87468811246272
Evaluated model with costs=61.79539470541433
Evaluated model with costs=61.66773134972334
Evaluated model with costs=61.19284828518643
Evaluated model with costs=60.244856214565196
Evaluated model with costs=58.90856947005
Evaluated model with costs=117.88516143642082
Evaluated model with costs=58.78329665087627
Evaluated model with costs=58.350016624329335
Evaluated model with costs=58.24392042970459
Evaluated model with costs=58.1441180014138
Evaluated model with costs=57.900309124929784
Evaluated model with costs=57.38240601066865
Evaluated model with costs=56.671910768716444
Evaluated model with costs=55.59365633310545
Evaluated model with costs=55.259498816124214
Evaluated model with costs=56.39444047072271
Evaluated model with costs=54.97559111513047
Evaluated model with costs=54.40096613546381
================================================================================
Time: 652.3953014310027
Iteration: 15
Costs
deformation=29.948461321028724
attach=24.452504814435088
Total cost=54.40096613546381
1e-10
Evaluated model with costs=54.40096613546381
Evaluated model with costs=54.137008388332205
Evaluated model with costs=53.8523362894063
Evaluated model with costs=54.18709591936561
Evaluated model with costs=53.58903636783748
Evaluated model with costs=53.30417896438962
Evaluated model with costs=52.74683301191757
Evaluated model with costs=52.18400645444494
Evaluated model with costs=51.615507821155816
Evaluated model with costs=2066.7547670761187
Evaluated model with costs=66.01577763030232
Evaluated model with costs=51.398191938515716
Evaluated model with costs=51.215004889877456
Evaluated model with costs=51.01112660962886
Evaluated model with costs=50.8662083581433
Evaluated model with costs=50.6614363326422
Evaluated model with costs=50.44169017028324
Evaluated model with costs=50.380753835861746
Evaluated model with costs=50.341943541422715
Evaluated model with costs=50.13504816662859
Evaluated model with costs=50.066801448961805
Evaluated model with costs=49.872112347104846
Evaluated model with costs=49.774642753832914
Evaluated model with costs=49.6349634258778
Evaluated model with costs=49.847096588757296
Evaluated model with costs=49.59453013511886
================================================================================
Time: 696.5392723260011
Iteration: 16
Costs
deformation=30.102809385689778
attach=19.491720749429078
Total cost=49.59453013511886
1e-10
Evaluated model with costs=49.59453013511886
Evaluated model with costs=49.54752013576343
Evaluated model with costs=49.48086194392346
Evaluated model with costs=49.46132322807084
Evaluated model with costs=49.410419824243355
Evaluated model with costs=49.3847937094083
Evaluated model with costs=49.36222619812426
Evaluated model with costs=49.797011942281586
Evaluated model with costs=49.36001507430038
Evaluated model with costs=49.34919053237739
Evaluated model with costs=49.33455597506165
Evaluated model with costs=49.32647217022897
Evaluated model with costs=49.31903776875991
Evaluated model with costs=49.31264953076993
Evaluated model with costs=49.30683395441831
Evaluated model with costs=49.302437125894116
Evaluated model with costs=49.2969732736517
Evaluated model with costs=49.290368558530496
Evaluated model with costs=49.282859033969004
Evaluated model with costs=49.27487101521144
Evaluated model with costs=49.389630985241716
Evaluated model with costs=49.2624994682025
Evaluated model with costs=49.253647616394105
================================================================================
Time: 735.7817114909994
Iteration: 17
Costs
deformation=30.304205849285598
attach=18.94944176710851
Total cost=49.253647616394105
1e-10
Evaluated model with costs=49.253647616394105
Evaluated model with costs=49.284267925196644
Evaluated model with costs=49.234775664583196
Evaluated model with costs=49.22583672115683
Evaluated model with costs=49.16251628881562
Evaluated model with costs=49.12291964324015
Evaluated model with costs=48.928949055537984
Evaluated model with costs=48.81832706258366
Evaluated model with costs=48.7711084813434
Evaluated model with costs=48.71335242796631
Evaluated model with costs=48.56240350573918
Evaluated model with costs=48.40611682234521
Evaluated model with costs=48.67922591098566
Evaluated model with costs=48.34644640181345
Evaluated model with costs=48.188582856623064
Evaluated model with costs=48.12790251005237
Evaluated model with costs=48.10929907656904
Evaluated model with costs=48.08253899032671
Evaluated model with costs=48.06522669922751
Evaluated model with costs=48.0511440222655
Evaluated model with costs=48.032171044363906
Evaluated model with costs=48.019698307742544
Evaluated model with costs=47.993553324271566
Evaluated model with costs=47.96370750471007
================================================================================
Time: 777.6286649310023
Iteration: 18
Costs
deformation=30.662227235475907
attach=17.301480269234162
Total cost=47.96370750471007
1e-10
Evaluated model with costs=47.96370750471007
Evaluated model with costs=47.91904744131888
Evaluated model with costs=47.84328363609172
Evaluated model with costs=47.76759198865641
Evaluated model with costs=47.66461022478849
Evaluated model with costs=47.582187855944866
Evaluated model with costs=47.435856616241665
Evaluated model with costs=47.356207592834025
Evaluated model with costs=47.239804496140685
Evaluated model with costs=47.15741739339863
Evaluated model with costs=46.986805659088
Evaluated model with costs=46.90406369651476
Evaluated model with costs=46.67364559706391
Evaluated model with costs=47.45273032075031
Evaluated model with costs=46.63502626378092
Evaluated model with costs=46.44278936113923
Evaluated model with costs=46.296662566124134
Evaluated model with costs=46.2262486247649
Evaluated model with costs=46.18795417338933
Evaluated model with costs=46.12806209158869
Evaluated model with costs=46.055607610014206
Evaluated model with costs=46.0590507828393
Evaluated model with costs=45.99965279703915
Evaluated model with costs=45.921332385815504
================================================================================
Time: 818.6251541650017
Iteration: 19
Costs
deformation=30.303355267615874
attach=15.617977118199633
Total cost=45.921332385815504
1e-10
Evaluated model with costs=45.921332385815504
Evaluated model with costs=45.79538570386889
Evaluated model with costs=46.08379590772501
Evaluated model with costs=45.75068445570372
Evaluated model with costs=45.69217564577321
Evaluated model with costs=45.61343338383064
Evaluated model with costs=45.828411785414346
Evaluated model with costs=45.58594155902987
Evaluated model with costs=45.542211860381684
Evaluated model with costs=45.461760699267685
Evaluated model with costs=45.90496779223277
Evaluated model with costs=45.44347213924874
Evaluated model with costs=45.40492296703336
Evaluated model with costs=45.36319154087499
Evaluated model with costs=45.38466890123931
Evaluated model with costs=45.349978102081316
Evaluated model with costs=45.30844328871969
Evaluated model with costs=45.300625140148675
Evaluated model with costs=45.27527118136062
Evaluated model with costs=45.26936317238751
Evaluated model with costs=45.25781817098107
Evaluated model with costs=45.25265598795238
Evaluated model with costs=45.24469419130246
Evaluated model with costs=45.23886412735654
Evaluated model with costs=45.22771508038838
================================================================================
Time: 860.6131187320025
Iteration: 20
Costs
deformation=29.963550281888246
attach=15.264164798500133
Total cost=45.22771508038838
1e-10
Evaluated model with costs=45.22771508038838
Evaluated model with costs=45.21145391500008
Evaluated model with costs=45.19659786967346
Evaluated model with costs=45.196969567084025
Evaluated model with costs=45.190086977972776
Evaluated model with costs=45.18021618664285
Evaluated model with costs=45.16779561319732
Evaluated model with costs=45.1831742282678
Evaluated model with costs=45.1646292439138
Evaluated model with costs=45.158354173436805
Evaluated model with costs=45.15499264584116
Evaluated model with costs=45.15401346036619
Evaluated model with costs=45.15196144538368
Evaluated model with costs=45.145325735674604
Evaluated model with costs=45.14071710384184
Evaluated model with costs=45.12945599399992
Evaluated model with costs=45.12315517494932
Evaluated model with costs=46.68508368080255
Evaluated model with costs=45.13296608731669
Evaluated model with costs=45.119585434767785
Evaluated model with costs=45.11548409977784
Evaluated model with costs=45.11144383081812
Evaluated model with costs=45.1064125849206
Evaluated model with costs=45.10112446692449
Evaluated model with costs=45.09101106825964
================================================================================
Time: 899.9816364690014
Iteration: 21
Costs
deformation=29.858994849608088
attach=15.232016218651552
Total cost=45.09101106825964
1e-10
Evaluated model with costs=45.09101106825964
Evaluated model with costs=45.08697238181351
Evaluated model with costs=45.07405242113135
Evaluated model with costs=45.02149522767823
Evaluated model with costs=44.86860463510784
Evaluated model with costs=424.8426311570839
Evaluated model with costs=49.36857013787058
Evaluated model with costs=44.833680337721816
Evaluated model with costs=44.81493698715187
Evaluated model with costs=44.73052541708079
Evaluated model with costs=44.55021140407573
Evaluated model with costs=44.47224203294997
Evaluated model with costs=44.31299717235754
Evaluated model with costs=44.23171428203109
Evaluated model with costs=44.15619775437972
Evaluated model with costs=44.09470786309748
Evaluated model with costs=44.06308258031748
Evaluated model with costs=44.02372201192756
Evaluated model with costs=43.99736160440574
Evaluated model with costs=43.98689474645422
Evaluated model with costs=43.96681151008981
Evaluated model with costs=43.95242402621014
Evaluated model with costs=43.989006749994665
Evaluated model with costs=43.937430101398895
Evaluated model with costs=43.92452876481583
================================================================================
Time: 938.9707009350022
Iteration: 22
Costs
deformation=29.21341011818767
attach=14.711118646628165
Total cost=43.92452876481583
1e-10
Evaluated model with costs=43.92452876481583
Evaluated model with costs=43.91215305448223
Evaluated model with costs=43.908459504733486
Evaluated model with costs=43.931325419995744
Evaluated model with costs=43.897106258037766
Evaluated model with costs=43.889011697643426
Evaluated model with costs=43.88167196117991
Evaluated model with costs=43.86756043255531
Evaluated model with costs=43.87093974213904
Evaluated model with costs=43.852266079958454
Evaluated model with costs=43.82782067837893
Evaluated model with costs=43.849793109575295
Evaluated model with costs=43.799903160784204
Evaluated model with costs=43.762418723669576
Evaluated model with costs=43.7291835829865
Evaluated model with costs=43.67424933179018
Evaluated model with costs=43.65507674789242
Evaluated model with costs=43.6235987154625
Evaluated model with costs=43.61170788073389
Evaluated model with costs=43.59915386343584
Evaluated model with costs=43.5865994303813
Evaluated model with costs=43.57419990476777
Evaluated model with costs=43.55740181379346
Evaluated model with costs=43.5735341111078
Evaluated model with costs=43.53879675294523
================================================================================
Time: 981.0042447380001
Iteration: 23
Costs
deformation=29.394989426108836
attach=14.143807326836395
Total cost=43.53879675294523
1e-10
Evaluated model with costs=43.53879675294523
Evaluated model with costs=43.51198790233028
Evaluated model with costs=43.4955507022243
Evaluated model with costs=43.776734936510564
Evaluated model with costs=43.485925462449416
Evaluated model with costs=43.464193751385054
Evaluated model with costs=43.45482025585239
Evaluated model with costs=43.441316669558155
Evaluated model with costs=43.4043819958213
Evaluated model with costs=43.40342564355977
Evaluated model with costs=43.39891739583931
Evaluated model with costs=43.385966751485824
Evaluated model with costs=43.37902045960561
Evaluated model with costs=43.37436446077322
Evaluated model with costs=43.366572497090615
Evaluated model with costs=43.360600790018125
Evaluated model with costs=43.362932759752724
Evaluated model with costs=43.3534689805891
Evaluated model with costs=43.34783122123796
Evaluated model with costs=43.34550008839405
Evaluated model with costs=43.3421677810882
Evaluated model with costs=43.342725581818186
Evaluated model with costs=43.341331091809025
Evaluated model with costs=43.339403037134424
Evaluated model with costs=43.33648138818461
================================================================================
Time: 1026.1777699470003
Iteration: 24
Costs
deformation=29.61042747546088
attach=13.726053912723728
Total cost=43.33648138818461
1e-10
Evaluated model with costs=43.33648138818461
Evaluated model with costs=43.33504126117822
Evaluated model with costs=43.333323660113074
Evaluated model with costs=43.33201942050167
Evaluated model with costs=43.33116866769213
Evaluated model with costs=43.33001882747144
Evaluated model with costs=43.32955724081613
Evaluated model with costs=43.32926953400982
Evaluated model with costs=43.32901740762688
Evaluated model with costs=43.41681068561848
Evaluated model with costs=43.32889309047181
Evaluated model with costs=43.328548898494944
Evaluated model with costs=43.3275764167884
Evaluated model with costs=43.32561590035532
Evaluated model with costs=43.3215904292384
Evaluated model with costs=43.31691418436423
Evaluated model with costs=43.308022103572796
Evaluated model with costs=43.29351639017941
Evaluated model with costs=43.28509205657724
Evaluated model with costs=43.27927860632151
Evaluated model with costs=43.26518953230058
Evaluated model with costs=43.25489478468931
Evaluated model with costs=43.2370456748354
================================================================================
Time: 1068.132262164003
Iteration: 25
Costs
deformation=29.74722086157593
attach=13.489824813259474
Total cost=43.2370456748354
1e-10
Evaluated model with costs=43.2370456748354
Evaluated model with costs=43.21834033006509
Evaluated model with costs=43.20970240370224
Evaluated model with costs=43.200466042189575
Evaluated model with costs=43.18289897065906
Evaluated model with costs=43.17540903493511
Evaluated model with costs=43.16697653872731
Evaluated model with costs=43.16205728624054
Evaluated model with costs=43.15680154303461
Evaluated model with costs=43.15196014738184
Evaluated model with costs=43.147754970961316
Evaluated model with costs=43.14210306235123
Evaluated model with costs=43.136172129157664
Evaluated model with costs=43.12858676394563
Evaluated model with costs=43.11618498788053
Evaluated model with costs=43.083197062297295
Evaluated model with costs=43.06311249072919
Evaluated model with costs=80.41637242438692
Evaluated model with costs=43.474653517238835
Evaluated model with costs=43.05775322810834
Evaluated model with costs=43.039589524261224
Evaluated model with costs=43.02543864607105
Evaluated model with costs=43.0433789746217
Evaluated model with costs=43.023129949251626
Evaluated model with costs=43.01781936408648
================================================================================
Time: 1110.1623932130024
Iteration: 26
Costs
deformation=30.059909524334813
attach=12.957909839751665
Total cost=43.01781936408648
1e-10
Evaluated model with costs=43.01781936408648
Evaluated model with costs=43.011018264072845
Evaluated model with costs=43.003048042276816
Evaluated model with costs=42.990024788819326
Evaluated model with costs=42.98721179135048
Evaluated model with costs=42.980065773877726
Evaluated model with costs=42.96547242252096
Evaluated model with costs=42.950709263875154
Evaluated model with costs=42.94378892279583
Evaluated model with costs=42.93249542575096
Evaluated model with costs=42.95002847788079
Evaluated model with costs=42.920395162337265
Evaluated model with costs=42.90039410089179
Evaluated model with costs=42.87037512631706
Evaluated model with costs=43.06518072102266
Evaluated model with costs=42.85307012420613
Evaluated model with costs=42.83292853125713
Evaluated model with costs=740.1932610178404
Evaluated model with costs=53.87572741602343
Evaluated model with costs=42.95953786027076
Evaluated model with costs=42.83196750445653
Evaluated model with costs=42.79456809475363
Evaluated model with costs=43.76937751572893
Evaluated model with costs=42.77942669483516
Evaluated model with costs=42.75556243386909
================================================================================
Time: 1149.5761551780015
Iteration: 27
Costs
deformation=30.404012521996084
attach=12.351549911873008
Total cost=42.75556243386909
1e-10
Evaluated model with costs=42.75556243386909
Evaluated model with costs=42.70970796582382
Evaluated model with costs=42.66618876588796
Evaluated model with costs=42.60373605645157
Evaluated model with costs=58.00052655604533
Evaluated model with costs=42.692633053409
Evaluated model with costs=42.56495495374941
Evaluated model with costs=42.533215397611876
Evaluated model with costs=42.470685350824766
Evaluated model with costs=42.4742599267568
Evaluated model with costs=42.440695035094336
Evaluated model with costs=42.38418607998482
Evaluated model with costs=42.315811438215974
Evaluated model with costs=42.474304635973965
Evaluated model with costs=42.27780719508703
Evaluated model with costs=42.233862230323524
Evaluated model with costs=42.15957299303851
Evaluated model with costs=42.247528700442906
Evaluated model with costs=42.13013977016317
Evaluated model with costs=42.069591509601054
Evaluated model with costs=41.987606557607464
Evaluated model with costs=42.24837724417985
Evaluated model with costs=41.95387397724846
Evaluated model with costs=41.902514120629284
Evaluated model with costs=41.837452407913645
================================================================================
Time: 1188.250697544001
Iteration: 28
Costs
deformation=30.747825892309326
attach=11.089626515604323
Total cost=41.837452407913645
1e-10
Evaluated model with costs=41.837452407913645
Evaluated model with costs=41.83099555152332
Evaluated model with costs=41.79954597770214
Evaluated model with costs=41.740688197827296
Evaluated model with costs=41.70226287516739
Evaluated model with costs=41.63909030481163
Evaluated model with costs=41.6133850599813
Evaluated model with costs=41.561222105227465
Evaluated model with costs=41.506145126452445
Evaluated model with costs=41.44442527823847
Evaluated model with costs=41.381806868764386
Evaluated model with costs=42.78829647275593
Evaluated model with costs=41.34749486808738
Evaluated model with costs=41.2981035426523
Evaluated model with costs=41.252385622859315
Evaluated model with costs=41.241637579831426
Evaluated model with costs=41.20250708330693
Evaluated model with costs=41.18226551533844
Evaluated model with costs=41.15436364408326
Evaluated model with costs=41.132920184085144
Evaluated model with costs=41.11330475253418
Evaluated model with costs=41.09348114699779
Evaluated model with costs=41.08578401851065
Evaluated model with costs=41.05465305338928
Evaluated model with costs=41.01382020692043
================================================================================
Time: 1227.0121898150028
Iteration: 29
Costs
deformation=30.589570877294392
attach=10.424249329626036
Total cost=41.01382020692043
1e-10
Evaluated model with costs=41.01382020692043
Evaluated model with costs=41.09836510223293
Evaluated model with costs=40.99037720754164
Evaluated model with costs=40.96428082240265
Evaluated model with costs=40.92267147391429
Evaluated model with costs=40.98552424900085
Evaluated model with costs=40.90714221522084
Evaluated model with costs=40.88228349466121
Evaluated model with costs=42.51841254671502
Evaluated model with costs=40.8815587008754
Evaluated model with costs=40.86626320516089
Evaluated model with costs=40.82858673362135
Evaluated model with costs=40.81296174961304
Evaluated model with costs=41.31235979328513
Evaluated model with costs=40.80044137668909
Evaluated model with costs=40.77955965605355
Evaluated model with costs=40.75936892531236
Evaluated model with costs=40.736774403950946
Evaluated model with costs=40.7139689089013
Evaluated model with costs=40.750430231963584
Evaluated model with costs=40.70390618219858
Evaluated model with costs=40.68853054490403
Evaluated model with costs=40.6716009993874
Evaluated model with costs=40.64542458360872
Evaluated model with costs=40.651452062955954
Evaluated model with costs=40.63959306263182
================================================================================
Time: 1267.476771427002
Iteration: 30
Costs
deformation=30.102974025584036
attach=10.536619037047785
Total cost=40.63959306263182
1e-10
Evaluated model with costs=40.63959306263182
Evaluated model with costs=40.622339788073916
Evaluated model with costs=40.6165897728389
Evaluated model with costs=40.60361682241367
Evaluated model with costs=40.59653409344537
Evaluated model with costs=40.57345568573435
Evaluated model with costs=40.612395890179485
Evaluated model with costs=40.56935051493319
Evaluated model with costs=40.56087215090993
Evaluated model with costs=40.55329755444113
Evaluated model with costs=40.549752538775536
Evaluated model with costs=40.54225313076264
Evaluated model with costs=40.53761949864324
Evaluated model with costs=40.52878976081961
Evaluated model with costs=40.52286568474702
Evaluated model with costs=40.51865400308339
Evaluated model with costs=40.56852642881725
Evaluated model with costs=40.51564217965897
Evaluated model with costs=40.51350303171071
Evaluated model with costs=40.510572098922154
Evaluated model with costs=40.50874627133281
Evaluated model with costs=40.506400200335946
Evaluated model with costs=40.51295243871797
Evaluated model with costs=40.5042775550029
================================================================================
Time: 1305.1361427940028
Iteration: 31
Costs
deformation=29.782254101730484
attach=10.722023453272413
Total cost=40.5042775550029
1e-10
Evaluated model with costs=40.5042775550029
Evaluated model with costs=40.50197924683581
Evaluated model with costs=40.500294369060434
Evaluated model with costs=40.498854235090825
Evaluated model with costs=40.49770498668511
Evaluated model with costs=40.4971243707142
Evaluated model with costs=40.49635346195733
Evaluated model with costs=40.49541811533
Evaluated model with costs=40.494800868698725
Evaluated model with costs=40.49412640206093
Evaluated model with costs=40.49360117197313
Evaluated model with costs=40.49316774149105
Evaluated model with costs=40.49274970354354
Evaluated model with costs=40.492613370572315
Evaluated model with costs=40.4924125647126
Evaluated model with costs=40.4921490444401
Evaluated model with costs=40.49160094289583
Evaluated model with costs=40.49093409953562
Evaluated model with costs=40.491384090546944
Evaluated model with costs=40.49070805962507
Evaluated model with costs=40.489847260610134
Evaluated model with costs=40.489069502197644
================================================================================
Time: 1339.532778932
Iteration: 32
Costs
deformation=29.647153943785206
attach=10.841915558412438
Total cost=40.489069502197644
1e-10
Evaluated model with costs=40.489069502197644
Evaluated model with costs=40.491079247067965
Evaluated model with costs=40.48850604581813
Evaluated model with costs=40.48772704745387
Evaluated model with costs=40.48637407589463
Evaluated model with costs=40.48627223643034
Evaluated model with costs=40.4855268330911
Evaluated model with costs=40.483228061747724
Evaluated model with costs=40.48359309108822
Evaluated model with costs=40.48161482450418
Evaluated model with costs=40.478460547025904
Evaluated model with costs=40.59134966989103
Evaluated model with costs=40.47622460860567
Evaluated model with costs=40.47335558466736
Evaluated model with costs=40.471222278645726
Evaluated model with costs=40.4804676916922
Evaluated model with costs=40.469058316263535
Evaluated model with costs=40.4656406708995
Evaluated model with costs=40.46042218822339
Evaluated model with costs=40.45819993448488
Evaluated model with costs=40.45255264339675
Evaluated model with costs=40.45085517039382
Evaluated model with costs=40.450992020735725
Evaluated model with costs=40.44744638253421
Evaluated model with costs=40.44625675993606
================================================================================
Time: 1379.0321146800015
Iteration: 33
Costs
deformation=29.459360979638713
attach=10.986895780297345
Total cost=40.44625675993606
1e-10
Evaluated model with costs=40.44625675993606
Evaluated model with costs=40.58002561545017
Evaluated model with costs=40.44611594225583
Evaluated model with costs=40.4443242424681
Evaluated model with costs=40.44366325390728
Evaluated model with costs=40.44238941601503
Evaluated model with costs=40.44171590324791
Evaluated model with costs=40.441146642586865
Evaluated model with costs=40.44035975874398
Evaluated model with costs=40.439804533382244
Evaluated model with costs=40.43929377712189
Evaluated model with costs=40.4387648330882
Evaluated model with costs=40.44049256980044
Evaluated model with costs=40.43815748368702
Evaluated model with costs=40.43756302813607
Evaluated model with costs=40.43680164027272
Evaluated model with costs=40.43509558698311
Evaluated model with costs=40.468365639924876
Evaluated model with costs=40.43483621341716
Evaluated model with costs=40.433875113585515
Evaluated model with costs=40.43286128369695
Evaluated model with costs=40.4314090100353
Evaluated model with costs=40.42982696032467
Evaluated model with costs=40.43137251159011
Evaluated model with costs=40.429275747758695
================================================================================
Time: 1418.1731506480028
Iteration: 34
Costs
deformation=29.542661955913832
attach=10.886613791844866
Total cost=40.429275747758695
1e-10
Evaluated model with costs=40.429275747758695
Evaluated model with costs=40.42761774162774
Evaluated model with costs=40.42544062656973
Evaluated model with costs=40.42201615823491
Evaluated model with costs=41.18189518185699
Evaluated model with costs=40.425854935198906
Evaluated model with costs=40.42042293686821
Evaluated model with costs=40.418911413771326
Evaluated model with costs=40.41508216977466
Evaluated model with costs=40.413488698705315
Evaluated model with costs=40.43157372304701
Evaluated model with costs=40.41199956785512
Evaluated model with costs=40.40945056451747
Evaluated model with costs=40.40718498706558
Evaluated model with costs=40.403408125107106
Evaluated model with costs=40.409580665517154
Evaluated model with costs=40.40213580592324
Evaluated model with costs=40.400340200522386
Evaluated model with costs=40.39809385150496
Evaluated model with costs=40.40009022645942
Evaluated model with costs=40.39727829745796
Evaluated model with costs=40.39586968396456
Evaluated model with costs=40.39367032288955
Evaluated model with costs=40.395037365960405
Evaluated model with costs=40.39312226940936
================================================================================
Time: 1457.457755902
Iteration: 35
Costs
deformation=29.561652841324108
attach=10.831469428085256
Total cost=40.39312226940936
1e-10
Evaluated model with costs=40.39312226940936
Evaluated model with costs=40.391889619879734
Evaluated model with costs=40.38963526934608
Evaluated model with costs=40.39154797434478
Evaluated model with costs=40.38885074136433
Evaluated model with costs=40.38668306037328
Evaluated model with costs=40.38586902723088
Evaluated model with costs=40.38428288889739
Evaluated model with costs=40.3831797604946
Evaluated model with costs=40.381148531567675
Evaluated model with costs=40.38014172832745
Evaluated model with costs=40.379451802025116
Evaluated model with costs=40.37908678200988
Evaluated model with costs=40.37698313893844
Evaluated model with costs=40.3757401405402
Evaluated model with costs=40.373258193407715
Evaluated model with costs=40.373456408906094
Evaluated model with costs=40.372645627527696
Evaluated model with costs=40.36941143028106
Evaluated model with costs=40.36685205385261
Evaluated model with costs=40.36514081531558
Evaluated model with costs=40.360310129244425
Evaluated model with costs=40.357541776554584
Evaluated model with costs=40.355429629342616
================================================================================
Time: 1494.9098767610012
Iteration: 36
Costs
deformation=29.324390956424686
attach=11.03103867291793
Total cost=40.355429629342616
1e-10
Evaluated model with costs=40.355429629342616
Evaluated model with costs=40.35751932384082
Evaluated model with costs=40.35231246509031
Evaluated model with costs=40.35467051896385
Evaluated model with costs=40.35098231016834
Evaluated model with costs=40.348566579093465
Evaluated model with costs=40.345486244110546
Evaluated model with costs=40.34446611341173
Evaluated model with costs=40.34043856667109
Evaluated model with costs=40.33591014690655
Evaluated model with costs=40.37120631626611
Evaluated model with costs=40.331930236143066
Evaluated model with costs=40.321980584755636
Evaluated model with costs=40.31196730292274
Evaluated model with costs=40.32191250001159
Evaluated model with costs=40.30682415570384
Evaluated model with costs=40.30346436427926
Evaluated model with costs=40.301616549666804
Evaluated model with costs=40.29754810662379
Evaluated model with costs=40.28522046422296
Evaluated model with costs=40.27777132494804
Evaluated model with costs=40.25694030669058
Evaluated model with costs=40.44608705223525
Evaluated model with costs=40.25405179468538
Evaluated model with costs=40.2371142305554
================================================================================
Time: 1533.6400004310017
Iteration: 37
Costs
deformation=29.095291043492416
attach=11.141823187062982
Total cost=40.2371142305554
1e-10
Evaluated model with costs=40.2371142305554
Evaluated model with costs=40.239226717533455
Evaluated model with costs=40.22991800038196
Evaluated model with costs=40.32333495228728
Evaluated model with costs=40.22612925567643
Evaluated model with costs=40.244483329720296
Evaluated model with costs=40.22001223964406
Evaluated model with costs=40.21125271327321
Evaluated model with costs=40.19867453488483
Evaluated model with costs=40.18581443869649
Evaluated model with costs=40.18248186536234
Evaluated model with costs=40.17253538359792
Evaluated model with costs=40.14982707879294
Evaluated model with costs=40.118394762145186
Evaluated model with costs=40.24528249170353
Evaluated model with costs=40.10725737580044
Evaluated model with costs=40.094612378510526
Evaluated model with costs=40.072299654031525
Evaluated model with costs=40.06435826512403
Evaluated model with costs=40.04149108141338
Evaluated model with costs=40.02965600264104
Evaluated model with costs=40.001896914511676
Evaluated model with costs=39.98535688903566
Evaluated model with costs=39.953186937718485
Evaluated model with costs=39.95642447354382
Evaluated model with costs=39.935645404141965
================================================================================
Time: 1579.8837496560009
Iteration: 38
Costs
deformation=28.974955820879828
attach=10.960689583262138
Total cost=39.935645404141965
1e-10
Evaluated model with costs=39.935645404141965
Evaluated model with costs=39.91301674591763
Evaluated model with costs=39.88543649823859
Evaluated model with costs=39.86217152447477
Evaluated model with costs=39.851726780048324
Evaluated model with costs=39.82942785457769
Evaluated model with costs=39.803261500500085
Evaluated model with costs=39.798605077990814
Evaluated model with costs=39.76730296761346
Evaluated model with costs=39.74401218766989
Evaluated model with costs=39.738522263001634
Evaluated model with costs=39.728004566102605
Evaluated model with costs=39.71124552958449
Evaluated model with costs=41.189744876583724
Evaluated model with costs=39.710315286901036
Evaluated model with costs=39.70661711257897
Evaluated model with costs=39.68344889618977
Evaluated model with costs=39.67969028722513
Evaluated model with costs=39.66698668856758
Evaluated model with costs=39.62217383469433
Evaluated model with costs=39.584206347798855
Evaluated model with costs=39.55424526077323
Evaluated model with costs=40.96275266707568
Evaluated model with costs=39.550270624789036
Evaluated model with costs=39.52011712792472
================================================================================
Time: 1619.9313711409995
Iteration: 39
Costs
deformation=28.840842574011447
attach=10.679274553913274
Total cost=39.52011712792472
1e-10
Evaluated model with costs=39.52011712792472
Evaluated model with costs=21920.969455424856
Evaluated model with costs=266.33589916418595
Evaluated model with costs=42.72462429129714
Evaluated model with costs=39.56589089982292
Evaluated model with costs=39.514557086081574
Evaluated model with costs=39.50101026240188
Evaluated model with costs=39.47639554430022
Evaluated model with costs=39.4552282901026
Evaluated model with costs=39.43099922193098
Evaluated model with costs=39.448655829892765
Evaluated model with costs=39.40953438102946
Evaluated model with costs=39.40009345416125
Evaluated model with costs=39.39064994717077
Evaluated model with costs=39.38522243829882
Evaluated model with costs=39.374911459540996
Evaluated model with costs=39.36760265075522
Evaluated model with costs=39.350714696650925
Evaluated model with costs=39.34190650028569
Evaluated model with costs=39.32855390704469
Evaluated model with costs=39.320408194803136
Evaluated model with costs=39.30422517581505
Evaluated model with costs=39.2918988470156
Evaluated model with costs=39.28273512959578
Evaluated model with costs=39.27200202018439
================================================================================
Time: 1659.155333736002
Iteration: 40
Costs
deformation=28.865247833560076
attach=10.406754186624312
Total cost=39.27200202018439
1e-10
Evaluated model with costs=39.27200202018439
Evaluated model with costs=39.27701685597792
Evaluated model with costs=39.262716012624836
Evaluated model with costs=39.25719260914942
Evaluated model with costs=39.253169754330656
Evaluated model with costs=39.24690557405259
Evaluated model with costs=39.241861489357895
Evaluated model with costs=39.23566977935462
Evaluated model with costs=39.229213295143296
Evaluated model with costs=39.24127514709435
Evaluated model with costs=39.22531409940707
Evaluated model with costs=39.21882043039185
Evaluated model with costs=39.21415559908056
Evaluated model with costs=39.25115246226234
Evaluated model with costs=39.21256604050599
Evaluated model with costs=39.20735624646648
Evaluated model with costs=39.20388408616913
Evaluated model with costs=39.21568194090924
Evaluated model with costs=39.20265956454452
Evaluated model with costs=39.198394537669586
Evaluated model with costs=39.19744832680906
Evaluated model with costs=39.1945440644652
Evaluated model with costs=39.19396449490732
Evaluated model with costs=39.192788442021616
Evaluated model with costs=39.19834784232724
Evaluated model with costs=39.19192295971777
================================================================================
Time: 1699.5700920350027
Iteration: 41
Costs
deformation=29.049776960774462
attach=10.142145998943306
Total cost=39.19192295971777
1e-10
Evaluated model with costs=39.19192295971777
Evaluated model with costs=39.1911481131842
Evaluated model with costs=39.19033242909368
Evaluated model with costs=39.18988569320974
Evaluated model with costs=39.18952015098365
Evaluated model with costs=39.18938628531852
Evaluated model with costs=39.189195964476
Evaluated model with costs=39.18901953129694
Evaluated model with costs=39.188895042407786
Evaluated model with costs=39.188693571407654
Evaluated model with costs=40.68329666036514
Evaluated model with costs=39.19277812448226
Evaluated model with costs=39.1886285325622
Evaluated model with costs=39.1884875932426
Evaluated model with costs=39.18853836587298
Evaluated model with costs=39.188441588270976
Evaluated model with costs=39.18842167230375
Evaluated model with costs=39.18835020089498
Evaluated model with costs=39.18821375144182
Evaluated model with costs=39.18743297968071
Evaluated model with costs=39.186721998553416
Evaluated model with costs=39.18537519725643
Evaluated model with costs=39.18269099103989
Evaluated model with costs=39.17961510630328
================================================================================
Time: 1737.3231853649995
Iteration: 42
Costs
deformation=29.122726909780503
attach=10.056888196522777
Total cost=39.17961510630328
1e-10
Evaluated model with costs=39.17961510630328
Evaluated model with costs=39.19017347134651
Evaluated model with costs=39.174608289787784
Evaluated model with costs=39.16698223458192
Evaluated model with costs=39.156570404377085
Evaluated model with costs=39.15407177177629
Evaluated model with costs=39.148561590778584
Evaluated model with costs=39.16654701336498
Evaluated model with costs=39.13933704874208
Evaluated model with costs=39.117483315039266
Evaluated model with costs=39.10461797301951
Evaluated model with costs=39.07361155507415
Evaluated model with costs=39.04089413859318
Evaluated model with costs=40.985438987057286
Evaluated model with costs=39.02075686143459
Evaluated model with costs=39.01169732603738
Evaluated model with costs=38.941243567572435
Evaluated model with costs=38.81435504142626
Evaluated model with costs=39.13021741924638
Evaluated model with costs=38.750875640791904
Evaluated model with costs=38.701519387285316
Evaluated model with costs=38.678644686117764
Evaluated model with costs=38.64418057180154
Evaluated model with costs=38.624378060951415
Evaluated model with costs=38.59836966196933
================================================================================
Time: 1776.2990662990014
Iteration: 43
Costs
deformation=29.294148977179862
attach=9.30422068478947
Total cost=38.59836966196933
1e-10
Evaluated model with costs=38.59836966196933
Evaluated model with costs=38.565120309245984
Evaluated model with costs=38.55087256154476
Evaluated model with costs=38.540186876346866
Evaluated model with costs=38.53358309199403
Evaluated model with costs=38.51811056534049
Evaluated model with costs=38.48392305889723
Evaluated model with costs=38.48671780009883
Evaluated model with costs=38.47475294297065
Evaluated model with costs=38.45266737483369
Evaluated model with costs=38.44186249593251
Evaluated model with costs=38.43140221852643
Evaluated model with costs=38.416854436720335
Evaluated model with costs=38.45033834376285
Evaluated model with costs=38.41055071507397
Evaluated model with costs=38.399287750244135
Evaluated model with costs=38.37334547061465
Evaluated model with costs=38.36201186256328
Evaluated model with costs=38.36820978114545
Evaluated model with costs=38.3529880390511
Evaluated model with costs=38.341429031404545
Evaluated model with costs=38.329423582481716
Evaluated model with costs=38.316826640085836
Evaluated model with costs=38.30480254458483
Evaluated model with costs=38.29602379884163
================================================================================
Time: 1815.314332277001
Iteration: 44
Costs
deformation=29.229271591041464
attach=9.066752207800164
Total cost=38.29602379884163
1e-10
Evaluated model with costs=38.29602379884163
Evaluated model with costs=38.27705416247336
Evaluated model with costs=38.26957779698404
Evaluated model with costs=38.266910366228856
Evaluated model with costs=38.25160634292767
Evaluated model with costs=38.245713973475695
Evaluated model with costs=38.235327948587766
Evaluated model with costs=38.229353508895834
Evaluated model with costs=38.22241388329579
Evaluated model with costs=38.28222480227316
Evaluated model with costs=38.21484843159266
Evaluated model with costs=38.204882112686875
Evaluated model with costs=38.19919676452399
Evaluated model with costs=38.20613852761894
Evaluated model with costs=38.19408650012695
Evaluated model with costs=38.18959826304515
Evaluated model with costs=38.18422061352034
Evaluated model with costs=38.181192570919684
Evaluated model with costs=38.17502253279174
Evaluated model with costs=38.1714263857946
Evaluated model with costs=38.16483425726129
Evaluated model with costs=38.1606355579672
Evaluated model with costs=38.155093595455966
Evaluated model with costs=38.152672297653645
================================================================================
Time: 1852.647212783002
Iteration: 45
Costs
deformation=29.217263736352344
attach=8.935408561301301
Total cost=38.152672297653645
1e-10
Evaluated model with costs=38.152672297653645
Evaluated model with costs=38.1501712989227
Evaluated model with costs=38.14906018075175
Evaluated model with costs=38.146271885348575
Evaluated model with costs=38.14567374675792
Evaluated model with costs=38.14336726911098
Evaluated model with costs=38.14280959465882
Evaluated model with costs=38.14041720369447
Evaluated model with costs=38.13936815949731
Evaluated model with costs=39.30131665020594
Evaluated model with costs=38.141769592897134
Evaluated model with costs=38.138751345079974
Evaluated model with costs=38.13731556326402
Evaluated model with costs=38.13616322686406
Evaluated model with costs=38.13440786141043
Evaluated model with costs=38.13340307041064
Evaluated model with costs=38.132690077536836
Evaluated model with costs=38.13172000621989
Evaluated model with costs=38.129735363995536
Evaluated model with costs=38.19854250464552
Evaluated model with costs=38.12949437385639
Evaluated model with costs=38.126558881165685
Evaluated model with costs=38.14132907381284
Evaluated model with costs=38.12550381598637
Evaluated model with costs=38.1220710593489
================================================================================
Time: 1891.7555289120028
Iteration: 46
Costs
deformation=29.223647757914517
attach=8.898423301434377
Total cost=38.1220710593489
1e-10
Evaluated model with costs=38.1220710593489
Evaluated model with costs=38.11952703601783
Evaluated model with costs=38.158816339482385
Evaluated model with costs=38.11806094112494
Evaluated model with costs=38.115309077870904
Evaluated model with costs=38.11529168472789
Evaluated model with costs=38.11186904822681
Evaluated model with costs=38.10512412088654
Evaluated model with costs=38.104082487683
Evaluated model with costs=38.10264977887178
Evaluated model with costs=38.093743080667224
Evaluated model with costs=38.08545263319753
Evaluated model with costs=38.27716651938016
Evaluated model with costs=38.07745934653997
Evaluated model with costs=38.0716486271164
Evaluated model with costs=38.066626678040656
Evaluated model with costs=38.05714340533752
Evaluated model with costs=38.04528411741581
Evaluated model with costs=38.30206316088462
Evaluated model with costs=38.038277570674374
Evaluated model with costs=38.02541639608156
Evaluated model with costs=38.01321794325743
Evaluated model with costs=38.000979176303375
Evaluated model with costs=37.98509917176988
Evaluated model with costs=37.96888942294608
================================================================================
Time: 1930.8021036330028
Iteration: 47
Costs
deformation=29.207807500452322
attach=8.761081922493759
Total cost=37.96888942294608
1e-10
Evaluated model with costs=37.96888942294608
Evaluated model with costs=37.959053879762976
Evaluated model with costs=37.95021208847676
Evaluated model with costs=37.93415054225104
Evaluated model with costs=37.92618499812114
Evaluated model with costs=37.92143739889029
Evaluated model with costs=37.90990211833892
Evaluated model with costs=37.91584637115093
Evaluated model with costs=37.90299255766535
Evaluated model with costs=37.89513791608229
Evaluated model with costs=37.88527374086681
Evaluated model with costs=37.86979744593816
Evaluated model with costs=37.9305016146387
Evaluated model with costs=37.8670417506843
Evaluated model with costs=37.86184958439074
Evaluated model with costs=37.85478451936747
Evaluated model with costs=37.85509777124372
Evaluated model with costs=37.85203932056474
Evaluated model with costs=37.84461914220935
Evaluated model with costs=37.84589007209447
Evaluated model with costs=37.84284263865062
Evaluated model with costs=37.84026425783905
Evaluated model with costs=37.84006172655815
Evaluated model with costs=37.83930721552919
Evaluated model with costs=37.83785118273551
================================================================================
Time: 1969.7354753660002
Iteration: 48
Costs
deformation=29.208718390432814
attach=8.629132792302698
Total cost=37.83785118273551
1e-10
Evaluated model with costs=37.83785118273551
Evaluated model with costs=37.83577348587595
Evaluated model with costs=37.83727074555354
Evaluated model with costs=37.83478162206319
Evaluated model with costs=37.836971991919526
Evaluated model with costs=37.833817734877826
Evaluated model with costs=37.83805598509436
Evaluated model with costs=37.83337114927653
Evaluated model with costs=37.83257253161252
Evaluated model with costs=38.027909018637814
Evaluated model with costs=37.83234181200961
Evaluated model with costs=37.83093438690383
Evaluated model with costs=37.82998967847699
Evaluated model with costs=37.82890885447338
Evaluated model with costs=37.8645855810818
Evaluated model with costs=37.82808204145276
Evaluated model with costs=37.827176422099235
Evaluated model with costs=37.826300879546984
Evaluated model with costs=37.8257175334698
Evaluated model with costs=37.82490011760704
Evaluated model with costs=37.82409749162333
Evaluated model with costs=37.823641281161215
Evaluated model with costs=37.82327626005328
Evaluated model with costs=37.82283923767382
Evaluated model with costs=37.822071810375846
================================================================================
Time: 2009.012133473003
Iteration: 49
Costs
deformation=29.14105037337059
attach=8.681021437005256
Total cost=37.822071810375846
1e-10
Evaluated model with costs=37.822071810375846
Evaluated model with costs=37.8221625458003
Evaluated model with costs=37.82159615653908
Evaluated model with costs=37.82110042141762
Evaluated model with costs=37.823977078742416
Evaluated model with costs=37.82016841928571
Evaluated model with costs=37.81961783191355
Evaluated model with costs=37.81915154114303
Evaluated model with costs=37.81954647640661
Evaluated model with costs=37.81894096908702
Evaluated model with costs=37.818505775210994
Evaluated model with costs=37.81762539075099
Evaluated model with costs=37.81700279882498
Evaluated model with costs=37.81579768135188
Evaluated model with costs=37.82742987879081
Evaluated model with costs=37.815612150620616
Evaluated model with costs=37.814669330037795
Evaluated model with costs=37.81377833069338
Evaluated model with costs=38.31903423109732
Evaluated model with costs=37.81378351120579
Evaluated model with costs=37.813767233026184
Evaluated model with costs=37.81333669400429
Evaluated model with costs=37.81540870592258
Evaluated model with costs=37.813020523710236
Evaluated model with costs=37.812702397374004
================================================================================
Time: 2048.5198285650004
Iteration: 50
Costs
deformation=29.079163564152758
attach=8.733538833221246
Total cost=37.812702397374004
1e-10
Evaluated model with costs=37.812702397374004
Evaluated model with costs=37.81213564596885
Evaluated model with costs=37.81171241375485
Evaluated model with costs=37.81130241510587
Evaluated model with costs=37.942231273833286
Evaluated model with costs=37.8116173310413
Evaluated model with costs=37.810853377119805
Evaluated model with costs=37.81030505868199
Evaluated model with costs=37.80997315036255
Evaluated model with costs=37.80958389968853
Evaluated model with costs=37.80924001018437
Evaluated model with costs=37.808694837167224
Evaluated model with costs=37.814691296745515
Evaluated model with costs=37.80865476099775
Evaluated model with costs=37.80817644878786
Evaluated model with costs=37.810292921838
Evaluated model with costs=37.80796881934278
Evaluated model with costs=37.80754913115307
Evaluated model with costs=37.806416443991864
Evaluated model with costs=37.80549752068788
Evaluated model with costs=37.8064255979616
Evaluated model with costs=37.80499093647899
Evaluated model with costs=37.811574498535606
Evaluated model with costs=37.804137763088214
Evaluated model with costs=37.803269812762295
================================================================================
Time: 2087.421486757001
Iteration: 51
Costs
deformation=29.040606589447265
attach=8.76266322331503
Total cost=37.803269812762295
1e-10
Evaluated model with costs=37.803269812762295
Evaluated model with costs=37.801889319662266
Evaluated model with costs=37.81049566016044
Evaluated model with costs=37.80120093956994
Evaluated model with costs=37.80024981293146
Evaluated model with costs=37.8270681171553
Evaluated model with costs=37.79998276283562
Evaluated model with costs=37.79851147513139
Evaluated model with costs=37.7965897178788
Evaluated model with costs=37.79269328287833
Evaluated model with costs=49.76154923584169
Evaluated model with costs=37.911061845107405
Evaluated model with costs=37.79105455805916
Evaluated model with costs=37.7872844111147
Evaluated model with costs=37.78508274168252
Evaluated model with costs=37.78377613833789
Evaluated model with costs=37.781482242745525
Evaluated model with costs=37.77891434355988
Evaluated model with costs=37.774503139978606
Evaluated model with costs=37.76923014473496
Evaluated model with costs=37.87091951280645
Evaluated model with costs=37.767249920578266
Evaluated model with costs=37.763489511416125
Evaluated model with costs=37.760390162600686
Evaluated model with costs=37.759223340132365
================================================================================
Time: 2126.5304827889995
Iteration: 52
Costs
deformation=29.019259005641715
attach=8.73996433449065
Total cost=37.759223340132365
1e-10
Evaluated model with costs=37.759223340132365
Evaluated model with costs=37.7556038034989
Evaluated model with costs=37.75464803617756
Evaluated model with costs=37.754091742741835
Evaluated model with costs=37.751668150142464
Evaluated model with costs=37.749500267710985
Evaluated model with costs=37.75165071388743
Evaluated model with costs=37.74862488684252
Evaluated model with costs=37.746277239292354
Evaluated model with costs=37.744893091795255
Evaluated model with costs=37.75272796502282
Evaluated model with costs=37.744132350063
Evaluated model with costs=37.743361709761885
Evaluated model with costs=37.74272500633044
Evaluated model with costs=37.74662794541515
Evaluated model with costs=37.74256614006164
Evaluated model with costs=37.7420257619436
Evaluated model with costs=37.7415934700814
Evaluated model with costs=37.740881246034434
Evaluated model with costs=37.74032090718027
Evaluated model with costs=37.739758417093
Evaluated model with costs=37.73911641550356
Evaluated model with costs=37.73756055488978
Evaluated model with costs=37.73633323446023
Evaluated model with costs=37.73643750256619
Evaluated model with costs=37.73593125898085
================================================================================
Time: 2167.1565958810024
Iteration: 53
Costs
deformation=29.056902574853005
attach=8.679028684127843
Total cost=37.73593125898085
1e-10
Evaluated model with costs=37.73593125898085
Evaluated model with costs=37.735119505198426
Evaluated model with costs=37.8395263560252
Evaluated model with costs=37.73480515073176
Evaluated model with costs=37.73402435790522
Evaluated model with costs=37.737539359740694
Evaluated model with costs=37.732851203889034
Evaluated model with costs=37.731546098193505
Evaluated model with costs=37.73083571398282
Evaluated model with costs=37.73042149698634
Evaluated model with costs=37.72981847562572
Evaluated model with costs=37.72893344373061
Evaluated model with costs=37.72931361218118
Evaluated model with costs=37.72852430433323
Evaluated model with costs=37.72755234758806
Evaluated model with costs=37.72624161724383
Evaluated model with costs=37.72478229827753
Evaluated model with costs=37.725915357999035
Evaluated model with costs=37.72399149827061
Evaluated model with costs=37.7241646610923
Evaluated model with costs=37.72330466845337
Evaluated model with costs=37.722651072767334
Evaluated model with costs=37.727664371504176
Evaluated model with costs=37.72250367200491
Evaluated model with costs=37.721744434223545
================================================================================
Time: 2206.3517749560015
Iteration: 54
Costs
deformation=29.079493268858094
attach=8.642251165365451
Total cost=37.721744434223545
1e-10
Evaluated model with costs=37.721744434223545
Evaluated model with costs=37.72199192781432
Evaluated model with costs=37.721379941837924
Evaluated model with costs=37.720846902092475
Evaluated model with costs=37.71974600632801
Evaluated model with costs=37.71946270752924
Evaluated model with costs=37.71827125179222
Evaluated model with costs=37.71764527725054
Evaluated model with costs=37.71643190898769
Evaluated model with costs=37.71588069930001
Evaluated model with costs=37.727094102225905
Evaluated model with costs=37.71550881792306
Evaluated model with costs=37.714989638885996
Evaluated model with costs=37.71477650286349
Evaluated model with costs=37.71432276070873
Evaluated model with costs=37.713447354124476
Evaluated model with costs=37.71335922201433
Evaluated model with costs=37.713099131245606
Evaluated model with costs=37.71227471613096
Evaluated model with costs=37.71154154922582
Evaluated model with costs=37.720171423967834
Evaluated model with costs=37.71101416630975
Evaluated model with costs=37.7105278446654
Evaluated model with costs=37.710888100203825
Evaluated model with costs=37.709885957560054
================================================================================
Time: 2245.3981940190024
Iteration: 55
Costs
deformation=29.125209326343047
attach=8.584676631217008
Total cost=37.709885957560054
1e-10
Evaluated model with costs=37.709885957560054
Evaluated model with costs=37.70879475752148
Evaluated model with costs=37.70897416083201
Evaluated model with costs=37.70846866666234
Evaluated model with costs=37.70776021858538
Evaluated model with costs=37.70633986922812
Evaluated model with costs=37.71076161208837
Evaluated model with costs=37.706002672154874
Evaluated model with costs=37.705121869546325
Evaluated model with costs=37.7039972052728
Evaluated model with costs=37.711939323692945
Evaluated model with costs=37.70355889747135
Evaluated model with costs=37.702726261940384
Evaluated model with costs=37.70073638221324
Evaluated model with costs=37.70029718445876
Evaluated model with costs=37.79771598010611
Evaluated model with costs=37.69847890437961
Evaluated model with costs=37.69814462303236
Evaluated model with costs=37.697319337435296
Evaluated model with costs=37.69756435919368
Evaluated model with costs=37.69661134358395
Evaluated model with costs=37.69597857072453
Evaluated model with costs=37.695103500452916
Evaluated model with costs=37.693788614144026
Evaluated model with costs=37.69195928655642
================================================================================
Time: 2285.565279925002
Iteration: 56
Costs
deformation=29.136173390278024
attach=8.555785896278394
Total cost=37.69195928655642
1e-10
Evaluated model with costs=37.69195928655642
Evaluated model with costs=37.68988779301346
Evaluated model with costs=37.69952418822975
Evaluated model with costs=37.68911949698962
Evaluated model with costs=37.6868334866906
Evaluated model with costs=37.68156076091805
Evaluated model with costs=37.799371337954106
Evaluated model with costs=37.680754982315854
Evaluated model with costs=37.67701514719136
Evaluated model with costs=37.673126656495796
Evaluated model with costs=37.665989808856
Evaluated model with costs=37.662863385677966
Evaluated model with costs=37.661725492840034
Evaluated model with costs=37.65816507128406
Evaluated model with costs=37.65311692517685
Evaluated model with costs=37.65143392354676
Evaluated model with costs=37.64757473402668
Evaluated model with costs=37.641729253189425
Evaluated model with costs=37.63438582407794
Evaluated model with costs=37.628566841625144
Evaluated model with costs=37.623438487842726
Evaluated model with costs=37.61915306650665
Evaluated model with costs=1006.9464481367113
Evaluated model with costs=52.6464789460351
Evaluated model with costs=37.872628670170585
Evaluated model with costs=37.623931125598986
Evaluated model with costs=37.618986139903114
================================================================================
Time: 2329.4155588400026
Iteration: 57
Costs
deformation=29.13172509460097
attach=8.487261045302148
Total cost=37.618986139903114
1e-10
Evaluated model with costs=37.618986139903114
Evaluated model with costs=37.615193201035865
Evaluated model with costs=37.61096682512142
Evaluated model with costs=37.602661960654395
Evaluated model with costs=37.591425538422556
Evaluated model with costs=37.671682052611445
Evaluated model with costs=37.58646193387696
Evaluated model with costs=37.56791563457187
Evaluated model with costs=37.56340105247743
Evaluated model with costs=37.52990222404358
Evaluated model with costs=37.52232151456641
Evaluated model with costs=37.504381153815956
Evaluated model with costs=37.49613259095757
Evaluated model with costs=37.48969902455762
Evaluated model with costs=37.47974296370607
Evaluated model with costs=37.470013887546486
Evaluated model with costs=37.462893277431974
Evaluated model with costs=37.45712810278405
Evaluated model with costs=37.44921986783456
Evaluated model with costs=37.4398997934883
Evaluated model with costs=37.42192286341067
Evaluated model with costs=37.4012566972141
Evaluated model with costs=37.36366895325384
Evaluated model with costs=37.3657191767881
Evaluated model with costs=37.358954791615226
================================================================================
Time: 2373.385273767002
Iteration: 58
Costs
deformation=28.976589300563184
attach=8.382365491052042
Total cost=37.358954791615226
1e-10
Evaluated model with costs=37.358954791615226
Evaluated model with costs=37.34696946516567
Evaluated model with costs=37.33998488558052
Evaluated model with costs=37.330911272162695
Evaluated model with costs=37.313189016700164
Evaluated model with costs=37.29347449890136
Evaluated model with costs=37.31326456308145
Evaluated model with costs=37.28807680548297
Evaluated model with costs=37.2684927295488
Evaluated model with costs=37.258781132958276
Evaluated model with costs=37.24440608285116
Evaluated model with costs=37.240608452804985
Evaluated model with costs=37.235239753783915
Evaluated model with costs=37.220494027610684
Evaluated model with costs=37.21295309854423
Evaluated model with costs=37.205957877495074
Evaluated model with costs=37.19754087845938
Evaluated model with costs=37.18937005033077
Evaluated model with costs=37.18090077715729
Evaluated model with costs=37.17290613213791
Evaluated model with costs=37.16865041865144
Evaluated model with costs=37.1656776879382
Evaluated model with costs=37.15932045948243
Evaluated model with costs=37.15037985722467
================================================================================
Time: 2413.488415402
Iteration: 59
Costs
deformation=28.73118290411532
attach=8.41919695310935
Total cost=37.15037985722467
1e-10
Evaluated model with costs=37.15037985722467
Evaluated model with costs=37.31485246472934
Evaluated model with costs=37.14519644406295
Evaluated model with costs=37.1304449407359
Evaluated model with costs=37.69636392128648
Evaluated model with costs=37.125109420497346
Evaluated model with costs=37.1153100016911
Evaluated model with costs=37.107502960169555
Evaluated model with costs=37.10159677962908
Evaluated model with costs=37.089967904236445
Evaluated model with costs=37.083250452177005
Evaluated model with costs=37.14302605069162
Evaluated model with costs=37.07647957722511
Evaluated model with costs=37.07158619921296
Evaluated model with costs=37.06616727272361
Evaluated model with costs=37.06030943673243
Evaluated model with costs=37.05347719727747
Evaluated model with costs=37.04521163783497
Evaluated model with costs=37.04051199096561
Evaluated model with costs=37.03546228567067
Evaluated model with costs=37.030010968010146
Evaluated model with costs=37.02633443075075
Evaluated model with costs=37.02107502673286
Evaluated model with costs=37.01680095468987
================================================================================
Time: 2452.4616306280004
Iteration: 60
Costs
deformation=28.70104866709085
attach=8.315752287599025
Total cost=37.01680095468987
1e-10
Evaluated model with costs=37.01680095468987
Evaluated model with costs=37.01244223442119
Evaluated model with costs=37.00791824091176
Evaluated model with costs=37.004462254394326
Evaluated model with costs=37.00626907650624
Evaluated model with costs=37.0013283620212
Evaluated model with costs=36.998495111785644
Evaluated model with costs=36.99421675092742
Evaluated model with costs=36.989872924522615
Evaluated model with costs=37.006981261510845
Evaluated model with costs=36.988523357949944
Evaluated model with costs=36.9861753204896
Evaluated model with costs=36.981744775691126
Evaluated model with costs=36.97875965423923
Evaluated model with costs=36.97649256815652
Evaluated model with costs=36.97777783337535
Evaluated model with costs=36.974610732232634
Evaluated model with costs=36.9901749342911
Evaluated model with costs=36.97220139213597
Evaluated model with costs=36.971141922160996
Evaluated model with costs=36.96942318383002
Evaluated model with costs=36.96857807734413
Evaluated model with costs=36.967717189138966
Evaluated model with costs=36.96690418863608
Evaluated model with costs=36.966105207563785
================================================================================
Time: 2491.9891526829997
Iteration: 61
Costs
deformation=28.69579232060217
attach=8.27031288696162
Total cost=36.966105207563785
1e-10
Evaluated model with costs=36.966105207563785
Evaluated model with costs=36.96505780928436
Evaluated model with costs=36.963603812842194
Evaluated model with costs=36.96257457777596
Evaluated model with costs=36.96251601838419
Evaluated model with costs=36.96203053278953
Evaluated model with costs=36.961254410907344
Evaluated model with costs=36.96031599141823
Evaluated model with costs=36.95971679556628
Evaluated model with costs=36.96065114423173
Evaluated model with costs=36.95938173521219
Evaluated model with costs=118.7260222783303
Evaluated model with costs=38.14569217197445
Evaluated model with costs=36.97864009369534
Evaluated model with costs=36.9590436607002
Evaluated model with costs=36.9752388587459
Evaluated model with costs=36.958140856877165
Evaluated model with costs=36.95748469298546
Evaluated model with costs=36.9544487702649
Evaluated model with costs=36.956204498660355
Evaluated model with costs=36.95315863008109
Evaluated model with costs=36.951049418402064
Evaluated model with costs=36.9478215372326
Evaluated model with costs=36.952043397425804
Evaluated model with costs=36.94669079593789
================================================================================
Time: 2531.0983356120014
Iteration: 62
Costs
deformation=28.711037445917306
attach=8.235653350020584
Total cost=36.94669079593789
1e-10
Evaluated model with costs=36.94669079593789
Evaluated model with costs=36.94288752288686
Evaluated model with costs=36.970083341817514
Evaluated model with costs=36.94130973878707
Evaluated model with costs=36.93791510377356
Evaluated model with costs=36.93484068890169
Evaluated model with costs=36.935085437709105
Evaluated model with costs=36.93418087371201
Evaluated model with costs=36.93288761006572
Evaluated model with costs=36.93238243643675
Evaluated model with costs=36.931057873263654
Evaluated model with costs=75.47594033245203
Evaluated model with costs=37.48438190362711
Evaluated model with costs=36.937078696994874
Evaluated model with costs=36.93100432176351
Evaluated model with costs=36.9295645476616
Evaluated model with costs=36.927098459138975
Evaluated model with costs=36.94505182832903
Evaluated model with costs=36.92624960602601
Evaluated model with costs=36.92386374189256
Evaluated model with costs=36.920844964202054
Evaluated model with costs=36.91890699534752
Evaluated model with costs=36.939584769122355
Evaluated model with costs=36.91823591891229
Evaluated model with costs=36.91534654258867
================================================================================
Time: 2570.105022841002
Iteration: 63
Costs
deformation=28.661123949347534
attach=8.254222593241138
Total cost=36.91534654258867
1e-10
Evaluated model with costs=36.91534654258867
Evaluated model with costs=36.913100574246165
Evaluated model with costs=36.940330197070026
Evaluated model with costs=36.912234399736136
Evaluated model with costs=36.90994924850861
Evaluated model with costs=36.90775446540141
Evaluated model with costs=36.904285490130704
Evaluated model with costs=36.96949745003439
Evaluated model with costs=36.903247942496535
Evaluated model with costs=36.90179969635387
Evaluated model with costs=36.8997549333818
Evaluated model with costs=36.89862671240226
Evaluated model with costs=46.245380918038435
Evaluated model with costs=37.005854883087636
Evaluated model with costs=36.89868340500479
Evaluated model with costs=36.8983539647099
Evaluated model with costs=36.89740729420659
Evaluated model with costs=36.89725175529399
Evaluated model with costs=36.89572856190642
Evaluated model with costs=36.89414845439086
Evaluated model with costs=36.892204294540846
Evaluated model with costs=36.89279956184062
Evaluated model with costs=36.891135147816954
Evaluated model with costs=36.88989322225167
Evaluated model with costs=36.88860291157134
================================================================================
Time: 2609.412602700002
Iteration: 64
Costs
deformation=28.656224085429397
attach=8.23237882614194
Total cost=36.88860291157134
1e-10
Evaluated model with costs=36.88860291157134
Evaluated model with costs=36.88709153254627
Evaluated model with costs=36.885673569797916
Evaluated model with costs=36.8881778795764
Evaluated model with costs=36.88428770281658
Evaluated model with costs=36.88122979356476
Evaluated model with costs=36.879881068870404
Evaluated model with costs=36.88555427089423
Evaluated model with costs=36.87752472596332
Evaluated model with costs=36.87624280159266
Evaluated model with costs=36.87250687143595
Evaluated model with costs=36.870423112664845
Evaluated model with costs=36.863998653211574
Evaluated model with costs=36.86053484865539
Evaluated model with costs=36.84926349501922
Evaluated model with costs=37.503320806599525
Evaluated model with costs=36.84950262589465
Evaluated model with costs=36.84795879746126
Evaluated model with costs=36.84059035416384
Evaluated model with costs=36.82937056477281
Evaluated model with costs=36.8310752950546
Evaluated model with costs=36.827298995701426
Evaluated model with costs=36.81975087398455
Evaluated model with costs=36.81285272819392
Evaluated model with costs=36.89270395882202
Evaluated model with costs=36.805998197384625
================================================================================
Time: 2650.4448171469994
Iteration: 65
Costs
deformation=28.64417000663424
attach=8.161828190750384
Total cost=36.805998197384625
1e-10
Evaluated model with costs=36.805998197384625
Evaluated model with costs=36.798710666344036
Evaluated model with costs=36.79772735663836
Evaluated model with costs=36.795037683330236
Evaluated model with costs=36.78935586651983
Evaluated model with costs=36.78613872196954
Evaluated model with costs=36.7802096352204
Evaluated model with costs=36.77581167486238
Evaluated model with costs=36.772870242515005
Evaluated model with costs=36.77189232202007
Evaluated model with costs=36.770913925243356
Evaluated model with costs=36.76677063036234
Evaluated model with costs=36.76532381582379
Evaluated model with costs=36.76291579986906
Evaluated model with costs=36.761747541419524
Evaluated model with costs=36.7600666806637
Evaluated model with costs=36.759327245586164
Evaluated model with costs=36.757764203567376
Evaluated model with costs=36.757429950126976
Evaluated model with costs=36.757084523843226
Evaluated model with costs=36.75687467028757
Evaluated model with costs=36.75665822113621
Evaluated model with costs=36.75677935220809
Evaluated model with costs=36.75658052604079
================================================================================
Time: 2688.0479545180024
Iteration: 66
Costs
deformation=28.726004459337428
attach=8.030576066703361
Total cost=36.75658052604079
1e-10
Evaluated model with costs=36.75658052604079
Evaluated model with costs=36.75698995749144
Evaluated model with costs=36.756457033450644
Evaluated model with costs=36.75633685734546
Evaluated model with costs=36.756256262154594
Evaluated model with costs=36.756125717334605
Evaluated model with costs=36.75607513986235
Evaluated model with costs=134.2161995433138
Evaluated model with costs=38.39013862780721
Evaluated model with costs=36.78855324838547
Evaluated model with costs=36.7560498488745
Evaluated model with costs=36.75593160980766
Evaluated model with costs=36.755756997598624
Evaluated model with costs=36.755426385473285
Evaluated model with costs=36.755144087573164
Evaluated model with costs=36.755021802652955
Evaluated model with costs=36.75465663512877
Evaluated model with costs=36.75431363776659
Evaluated model with costs=36.75360875864563
Evaluated model with costs=36.752947680210454
Evaluated model with costs=36.751292389577884
Evaluated model with costs=36.75030081273559
Evaluated model with costs=36.74983629518528
Evaluated model with costs=36.747780141810196
Evaluated model with costs=36.74135608433692
================================================================================
Time: 2726.9476517820003
Iteration: 67
Costs
deformation=28.743745642411277
attach=7.997610441925644
Total cost=36.74135608433692
1e-10
Evaluated model with costs=36.74135608433692
Evaluated model with costs=37.090198424775224
Evaluated model with costs=36.74114761698228
Evaluated model with costs=36.7393671956727
Evaluated model with costs=36.73198998683745
Evaluated model with costs=36.71034112851508
Evaluated model with costs=36.69757017140946
Evaluated model with costs=42.0902366517286
Evaluated model with costs=36.74604082481433
Evaluated model with costs=36.69054056827426
Evaluated model with costs=36.677470209133645
Evaluated model with costs=36.68362475383402
Evaluated model with costs=36.64540510443574
Evaluated model with costs=37.5437275764358
Evaluated model with costs=36.63207487887343
Evaluated model with costs=36.62798253876444
Evaluated model with costs=36.6193289318232
Evaluated model with costs=36.619295733020735
Evaluated model with costs=36.612466557354594
Evaluated model with costs=36.60327014939622
Evaluated model with costs=36.592575210815326
Evaluated model with costs=36.585876306675914
Evaluated model with costs=36.57573181324489
Evaluated model with costs=36.5671246528596
Evaluated model with costs=36.55878654373867
================================================================================
Time: 2765.5060492449993
Iteration: 68
Costs
deformation=28.680887679203288
attach=7.877898864535382
Total cost=36.55878654373867
1e-10
Evaluated model with costs=36.55878654373867
Evaluated model with costs=36.551435839303885
Evaluated model with costs=39.27709794510058
Evaluated model with costs=36.59669613806826
Evaluated model with costs=36.550770209933006
Evaluated model with costs=36.54427721529607
Evaluated model with costs=36.542080002701105
Evaluated model with costs=36.536645150857495
Evaluated model with costs=36.53112415916371
Evaluated model with costs=36.527911257838404
Evaluated model with costs=36.5250061919601
Evaluated model with costs=36.52240384398176
Evaluated model with costs=36.51838687848496
Evaluated model with costs=36.51460244785995
Evaluated model with costs=36.511425871831136
Evaluated model with costs=36.50931829981365
Evaluated model with costs=36.50724129292213
Evaluated model with costs=36.505625646422736
Evaluated model with costs=132934.76447904576
Evaluated model with costs=110.48256502632815
Evaluated model with costs=37.70763365586129
Evaluated model with costs=36.5347496812133
Evaluated model with costs=36.505423722365855
Evaluated model with costs=36.50323849579758
Evaluated model with costs=36.50423061869923
Evaluated model with costs=36.502475746492905
================================================================================
Time: 2806.295414201002
Iteration: 69
Costs
deformation=28.710868523521977
attach=7.791607222970924
Total cost=36.502475746492905
1e-10
Evaluated model with costs=36.502475746492905
Evaluated model with costs=36.500285747734885
Evaluated model with costs=36.49819443039244
Evaluated model with costs=36.49753050211407
Evaluated model with costs=36.49565026189329
Evaluated model with costs=36.491704426935655
Evaluated model with costs=36.490017025359435
Evaluated model with costs=36.48852709681442
Evaluated model with costs=36.484443177849634
Evaluated model with costs=36.48245787081045
Evaluated model with costs=36.47967909740444
Evaluated model with costs=36.47615516599147
Evaluated model with costs=36.47207821188967
Evaluated model with costs=36.49305291470131
Evaluated model with costs=36.470205596009826
Evaluated model with costs=36.46590047227312
Evaluated model with costs=36.46247834773172
Evaluated model with costs=36.54672824252263
Evaluated model with costs=36.460802377849845
Evaluated model with costs=36.45938129853154
Evaluated model with costs=36.45755412719634
Evaluated model with costs=36.45623384721692
Evaluated model with costs=36.4549718129137
Evaluated model with costs=36.45384128694107
================================================================================
Time: 2845.1519399530007
Iteration: 70
Costs
deformation=28.70770433267583
attach=7.746136954265239
Total cost=36.45384128694107
1e-10
Evaluated model with costs=36.45384128694107
Evaluated model with costs=36.45328145582057
Evaluated model with costs=36.45276825471373
Evaluated model with costs=36.45224025197261
Evaluated model with costs=36.45131948654465
Evaluated model with costs=36.450378860946195
Evaluated model with costs=36.44873069772302
Evaluated model with costs=36.44560871664562
Evaluated model with costs=36.44789558650719
Evaluated model with costs=36.444445176561906
Evaluated model with costs=36.44305740177857
Evaluated model with costs=36.442332907023314
Evaluated model with costs=36.44095259533207
Evaluated model with costs=36.44398262191157
Evaluated model with costs=36.44049217914939
Evaluated model with costs=36.438931380466215
Evaluated model with costs=36.43864662227195
Evaluated model with costs=36.43842679745068
Evaluated model with costs=36.43731388307649
Evaluated model with costs=36.43644792815452
Evaluated model with costs=36.43595598912171
Evaluated model with costs=36.434632877432676
Evaluated model with costs=36.43593609334513
Evaluated model with costs=36.43403329838249
Evaluated model with costs=36.4333681430083
================================================================================
Time: 2891.473167811
Iteration: 71
Costs
deformation=28.658221286169756
attach=7.775146856838546
Total cost=36.4333681430083
1e-10
Evaluated model with costs=36.4333681430083
Evaluated model with costs=36.43230394922789
Evaluated model with costs=36.43163370392142
Evaluated model with costs=36.43138031357772
Evaluated model with costs=36.43084825346213
Evaluated model with costs=36.42961386547235
Evaluated model with costs=36.42830729770196
Evaluated model with costs=36.4259410239701
Evaluated model with costs=36.543851972209595
Evaluated model with costs=36.42562772413389
Evaluated model with costs=36.42517039588618
Evaluated model with costs=36.423709501954846
Evaluated model with costs=36.4218675861468
Evaluated model with costs=36.421522472868304
Evaluated model with costs=36.4211791468703
Evaluated model with costs=36.41999263070675
Evaluated model with costs=36.418461918907994
Evaluated model with costs=36.41652373633599
Evaluated model with costs=36.41484224572597
Evaluated model with costs=36.410682274062935
Evaluated model with costs=36.42312252022347
Evaluated model with costs=36.40935223430379
Evaluated model with costs=36.40676041187231
Evaluated model with costs=36.402256849519496
Evaluated model with costs=36.39897285773404
================================================================================
Time: 2937.832035292002
Iteration: 72
Costs
deformation=28.688158892020144
attach=7.710813965713896
Total cost=36.39897285773404
1e-10
Evaluated model with costs=36.39897285773404
Evaluated model with costs=36.392130846096684
Evaluated model with costs=106659.28150999508
Evaluated model with costs=94.92679617031702
Evaluated model with costs=37.14767306046312
Evaluated model with costs=36.39756892095515
Evaluated model with costs=36.39211484843524
Evaluated model with costs=36.386093323933714
Evaluated model with costs=36.38178487051353
Evaluated model with costs=36.94758341021534
Evaluated model with costs=36.38085761859949
Evaluated model with costs=36.37839489637697
Evaluated model with costs=36.37446138860064
Evaluated model with costs=36.3711025042623
Evaluated model with costs=36.36732547903926
Evaluated model with costs=36.36488071918838
Evaluated model with costs=36.3613047435738
Evaluated model with costs=36.35634119277547
Evaluated model with costs=78058.60934798389
Evaluated model with costs=36.35633735419164
Evaluated model with costs=166.0601082755371
Evaluated model with costs=37.901891220226936
Evaluated model with costs=36.365988159089945
Evaluated model with costs=36.35471492716757
Evaluated model with costs=36.348093212520276
================================================================================
Time: 2983.416161068002
Iteration: 73
Costs
deformation=28.71089737150391
attach=7.637195841016364
Total cost=36.348093212520276
1e-10
Evaluated model with costs=36.348093212520276
Evaluated model with costs=39.218379987841786
Evaluated model with costs=36.3950970353255
Evaluated model with costs=36.34802174682676
Evaluated model with costs=36.34401487211146
Evaluated model with costs=36.49732222940118
Evaluated model with costs=36.337803000985396
Evaluated model with costs=36.333969799200815
Evaluated model with costs=36.32876771702878
Evaluated model with costs=36.3240426794327
Evaluated model with costs=36.32078835580441
Evaluated model with costs=36.315712583678206
Evaluated model with costs=36.31205651601665
Evaluated model with costs=36.32372083257032
Evaluated model with costs=36.309204899990426
Evaluated model with costs=36.30497734452618
Evaluated model with costs=36.300914480810306
Evaluated model with costs=36.298040849347146
Evaluated model with costs=36.296701700583064
Evaluated model with costs=36.29384026450773
Evaluated model with costs=36.28978296729769
Evaluated model with costs=36.28436984655798
Evaluated model with costs=51.16556123161282
Evaluated model with costs=36.3945247285822
Evaluated model with costs=36.28436270715684
================================================================================
Time: 3028.974822866003
Iteration: 74
Costs
deformation=28.913359101826067
attach=7.37100360533077
Total cost=36.28436270715684
1e-10
Evaluated model with costs=36.28436270715684
Evaluated model with costs=36.283171730008036
Evaluated model with costs=36.27899961182648
Evaluated model with costs=36.27587073467859
Evaluated model with costs=36.27310158756687
Evaluated model with costs=36.27547170239184
Evaluated model with costs=36.270645404658055
Evaluated model with costs=36.26773469026177
Evaluated model with costs=36.26518728951072
Evaluated model with costs=36.262047146536894
Evaluated model with costs=36.25999452003827
Evaluated model with costs=36.25617709672374
Evaluated model with costs=36.252898546213736
Evaluated model with costs=36.2525273629887
Evaluated model with costs=36.25097607636033
Evaluated model with costs=36.24869167852962
Evaluated model with costs=36.24640743177144
Evaluated model with costs=36.24462700546641
Evaluated model with costs=36.24377240056211
Evaluated model with costs=36.24187426199498
Evaluated model with costs=36.24017262541793
Evaluated model with costs=36.23856184493962
Evaluated model with costs=36.23638641877521
================================================================================
Time: 3070.909592115
Iteration: 75
Costs
deformation=29.03609306830519
attach=7.2002933504700195
Total cost=36.23638641877521
1e-10
Evaluated model with costs=36.23638641877521
Evaluated model with costs=36.234514158427146
Evaluated model with costs=36.23342073189954
Evaluated model with costs=36.230193828165895
Evaluated model with costs=36.23243538961199
Evaluated model with costs=36.22831587701571
Evaluated model with costs=36.2257725715897
Evaluated model with costs=36.232622576489916
Evaluated model with costs=36.224785698485846
Evaluated model with costs=36.22319803315894
Evaluated model with costs=36.224374207245866
Evaluated model with costs=36.22242561538374
Evaluated model with costs=36.22068712220669
Evaluated model with costs=36.21980198028875
Evaluated model with costs=36.218380263940105
Evaluated model with costs=36.21782536564025
Evaluated model with costs=36.21561880125208
Evaluated model with costs=36.212174253612986
Evaluated model with costs=36.21176201291578
Evaluated model with costs=36.31072260498088
Evaluated model with costs=36.20910606027172
Evaluated model with costs=36.207864180602485
Evaluated model with costs=36.207008181512634
Evaluated model with costs=36.20647111724875
Evaluated model with costs=36.205748285220324
================================================================================
Time: 3117.212440497002
Iteration: 76
Costs
deformation=29.117716637129636
attach=7.088031648090691
Total cost=36.205748285220324
1e-10
Evaluated model with costs=36.205748285220324
Evaluated model with costs=36.20466814445923
Evaluated model with costs=36.20347827014636
Evaluated model with costs=36.202482238688205
Evaluated model with costs=36.20132355976905
Evaluated model with costs=36.19947301938458
Evaluated model with costs=36.27904732261507
Evaluated model with costs=36.19919702020918
Evaluated model with costs=36.19891890674556
Evaluated model with costs=36.19779042507676
Evaluated model with costs=36.195483461827465
Evaluated model with costs=36.19710092301148
Evaluated model with costs=36.19504896232124
Evaluated model with costs=36.193905557798516
Evaluated model with costs=36.192067925526885
Evaluated model with costs=36.190858882662766
Evaluated model with costs=36.18954328063331
Evaluated model with costs=36.37481484654099
Evaluated model with costs=36.18931771608613
Evaluated model with costs=36.188284423756
Evaluated model with costs=36.187168288722404
Evaluated model with costs=36.18590308509844
Evaluated model with costs=36.18526641031307
Evaluated model with costs=36.18458702653594
Evaluated model with costs=36.18304192317586
================================================================================
Time: 3162.607292259003
Iteration: 77
Costs
deformation=29.210662527328473
attach=6.972379395847383
Total cost=36.18304192317586
1e-10
Evaluated model with costs=36.18304192317586
Evaluated model with costs=36.18013925517739
Evaluated model with costs=36.181111517522645
Evaluated model with costs=36.17847571083489
Evaluated model with costs=36.17552478293029
Evaluated model with costs=36.17327098404586
Evaluated model with costs=36.185022832559795
Evaluated model with costs=36.1717809809899
Evaluated model with costs=36.16969696998295
Evaluated model with costs=36.168096596741236
Evaluated model with costs=36.17029440765121
Evaluated model with costs=36.164220240585685
Evaluated model with costs=36.16026355069479
Evaluated model with costs=36.15403998589609
Evaluated model with costs=36.168624843076685
Evaluated model with costs=36.15090946232743
Evaluated model with costs=36.1474090409756
Evaluated model with costs=36.14210436238951
Evaluated model with costs=36.14232360007184
Evaluated model with costs=36.138977109860996
Evaluated model with costs=36.13485517157211
Evaluated model with costs=36.13120389645487
Evaluated model with costs=36.129294064817444
Evaluated model with costs=36.126118153418176
Evaluated model with costs=36.12398101174547
================================================================================
Time: 3208.748084218001
Iteration: 78
Costs
deformation=29.18787496885996
attach=6.936106042885513
Total cost=36.12398101174547
1e-10
Evaluated model with costs=36.12398101174547
Evaluated model with costs=36.12126383796991
Evaluated model with costs=36.11884562855447
Evaluated model with costs=36.11622108703216
Evaluated model with costs=36.112216335503184
Evaluated model with costs=36.10781637790469
Evaluated model with costs=36.104422457571445
Evaluated model with costs=36.10033261611466
Evaluated model with costs=36.105283757755544
Evaluated model with costs=36.09595920092637
Evaluated model with costs=36.090390954329564
Evaluated model with costs=36.085851426608166
Evaluated model with costs=36.07927988793555
Evaluated model with costs=36.073703260910946
Evaluated model with costs=36.06631908539113
Evaluated model with costs=36.06465185494325
Evaluated model with costs=36.05906132422864
Evaluated model with costs=36.05368368582599
Evaluated model with costs=36.04671813839683
Evaluated model with costs=36.0380039686767
Evaluated model with costs=36.02673322637394
Evaluated model with costs=247377.39235312215
Evaluated model with costs=117816.13986545544
Evaluated model with costs=36.02669422887262
Evaluated model with costs=107.08670566686914
Evaluated model with costs=36.76540328386745
Evaluated model with costs=36.02375661321254
================================================================================
Time: 3259.299568950002
Iteration: 79
Costs
deformation=29.083304309205925
attach=6.940452304006612
Total cost=36.02375661321254
1e-10
Evaluated model with costs=36.02375661321254
Evaluated model with costs=36.0371563002969
Evaluated model with costs=36.01878902473851
Evaluated model with costs=36.00977319613854
Evaluated model with costs=35.994618753419765
Evaluated model with costs=35.98409807808388
Evaluated model with costs=35.970877731244144
Evaluated model with costs=35.96207507500891
Evaluated model with costs=35.991281193676244
Evaluated model with costs=35.952908115022765
Evaluated model with costs=35.94392506162707
Evaluated model with costs=35.932701410962714
Evaluated model with costs=35.92151298765064
Evaluated model with costs=35.93347996289425
Evaluated model with costs=35.916750449697645
Evaluated model with costs=35.90821148158305
Evaluated model with costs=35.895659481627334
Evaluated model with costs=35.89094663530244
Evaluated model with costs=35.8907178486603
Evaluated model with costs=35.87456145854
Evaluated model with costs=35.860837700843646
Evaluated model with costs=35.82299080489382
Evaluated model with costs=2193.3005147316894
Evaluated model with costs=64.49195545398139
Evaluated model with costs=36.28213180063701
Evaluated model with costs=35.82914772274474
Evaluated model with costs=35.82181006837319
================================================================================
Time: 3309.017995428003
Iteration: 80
Costs
deformation=28.95804933265
attach=6.86376073572319
Total cost=35.82181006837319
1e-10
Evaluated model with costs=35.82181006837319
Evaluated model with costs=35.8297730811425
Evaluated model with costs=35.81077917706179
Evaluated model with costs=36.21702605670056
Evaluated model with costs=35.77872909048808
Evaluated model with costs=35.753657004421896
Evaluated model with costs=35.725042866115885
Evaluated model with costs=35.70322382391127
Evaluated model with costs=35.70332047836627
Evaluated model with costs=35.693210641148035
Evaluated model with costs=35.67672983044653
Evaluated model with costs=35.67635466720949
Evaluated model with costs=35.66102136330747
Evaluated model with costs=35.65329493585408
Evaluated model with costs=35.64195693881945
Evaluated model with costs=35.92812870965812
Evaluated model with costs=35.636371393853764
Evaluated model with costs=35.610068030834135
Evaluated model with costs=35.59728611034295
Evaluated model with costs=35.65500350028541
Evaluated model with costs=35.59076112767541
Evaluated model with costs=35.57741652312052
Evaluated model with costs=54.50660004979749
Evaluated model with costs=35.817824084321956
Evaluated model with costs=35.57581119578495
================================================================================
Time: 3355.0857688070028
Iteration: 81
Costs
deformation=29.20556940353813
attach=6.370241792246816
Total cost=35.57581119578495
1e-10
Evaluated model with costs=35.57581119578495
Evaluated model with costs=35.554692004807706
Evaluated model with costs=35.5470681810656
Evaluated model with costs=35.53098832580049
Evaluated model with costs=35.52578936669306
Evaluated model with costs=35.51926194960407
Evaluated model with costs=35.49675625624603
Evaluated model with costs=35.42835973633332
Evaluated model with costs=35.39730490045997
Evaluated model with costs=35.3781581665279
Evaluated model with costs=36.31358504901013
Evaluated model with costs=35.322906318671556
Evaluated model with costs=35.291435677482774
Evaluated model with costs=35.28062169699471
Evaluated model with costs=35.24537262574076
Evaluated model with costs=35.302017337007435
Evaluated model with costs=35.23573022874396
Evaluated model with costs=35.21832456118665
Evaluated model with costs=35.189651234603865
Evaluated model with costs=35.16629933156128
Evaluated model with costs=35.14962615126582
Evaluated model with costs=35.155279336291215
Evaluated model with costs=35.13874368959253
Evaluated model with costs=35.131721410073695
Evaluated model with costs=35.13110911890742
================================================================================
Time: 3400.370825152
Iteration: 82
Costs
deformation=29.33515089013433
attach=5.795958228773088
Total cost=35.13110911890742
1e-10
Evaluated model with costs=35.13110911890742
Evaluated model with costs=35.12772659898471
Evaluated model with costs=35.12440175283052
Evaluated model with costs=35.11947642316903
Evaluated model with costs=35.116492582294086
Evaluated model with costs=35.11296954186455
Evaluated model with costs=35.10700211751147
Evaluated model with costs=35.10076183275238
Evaluated model with costs=35.097270699293865
Evaluated model with costs=35.09602245319395
Evaluated model with costs=35.093019381275624
Evaluated model with costs=35.0908757289572
Evaluated model with costs=35.088310552557594
Evaluated model with costs=35.392724693822736
Evaluated model with costs=35.088001783275644
Evaluated model with costs=35.08596228235258
Evaluated model with costs=35.083860399060384
Evaluated model with costs=35.08136757652825
Evaluated model with costs=35.07889344506203
Evaluated model with costs=35.07610660682364
Evaluated model with costs=35.07409229771753
Evaluated model with costs=35.07530501698471
Evaluated model with costs=35.072998372746746
Evaluated model with costs=35.07228845976016
Evaluated model with costs=35.07169734771258
================================================================================
Time: 3446.3222972229996
Iteration: 83
Costs
deformation=29.31401110993709
attach=5.757686237775488
Total cost=35.07169734771258
1e-10
Evaluated model with costs=35.07169734771258
Evaluated model with costs=35.071159260735485
Evaluated model with costs=35.07035593519669
Evaluated model with costs=35.06895819759901
Evaluated model with costs=35.0706609576532
Evaluated model with costs=35.068160476114706
Evaluated model with costs=35.066297082223585
Evaluated model with costs=35.063509193420145
Evaluated model with costs=35.060445856016415
Evaluated model with costs=35.07688506648718
Evaluated model with costs=35.05895333739362
Evaluated model with costs=35.0571553617627
Evaluated model with costs=35.05677169374579
Evaluated model with costs=35.0546402494263
Evaluated model with costs=35.05264211755792
Evaluated model with costs=35.051047199635406
Evaluated model with costs=35.04912115077131
Evaluated model with costs=35.0473909262778
Evaluated model with costs=35.04546691795262
Evaluated model with costs=35.051756670579195
Evaluated model with costs=35.044499425892795
Evaluated model with costs=35.04328654960447
Evaluated model with costs=35.0403845665698
Evaluated model with costs=35.043290716262725
Evaluated model with costs=35.03975530341272
================================================================================
Time: 3492.4361957540023
Iteration: 84
Costs
deformation=29.361853198432115
attach=5.677902104980603
Total cost=35.03975530341272
1e-10
Evaluated model with costs=35.03975530341272
Evaluated model with costs=35.03752444490122
Evaluated model with costs=35.035448394082294
Evaluated model with costs=35.05171038652453
Evaluated model with costs=35.03432012608437
Evaluated model with costs=35.03261925326336
Evaluated model with costs=35.03000430773699
Evaluated model with costs=35.33764648813338
Evaluated model with costs=35.03050030601526
Evaluated model with costs=35.029941254315375
Evaluated model with costs=35.02965208107959
Evaluated model with costs=35.102512104438325
Evaluated model with costs=35.027572227340144
Evaluated model with costs=35.026782229668925
Evaluated model with costs=35.02626633112047
Evaluated model with costs=35.026045475050864
Evaluated model with costs=35.025804314083594
Evaluated model with costs=35.02536414454656
Evaluated model with costs=35.02510031130748
Evaluated model with costs=35.02468808643778
Evaluated model with costs=35.024561094715835
Evaluated model with costs=35.02446753305782
Evaluated model with costs=35.02393712004921
Evaluated model with costs=35.023377412304356
Evaluated model with costs=35.02678753658641
Evaluated model with costs=35.02310351643105
================================================================================
Time: 3539.750016831
Iteration: 85
Costs
deformation=29.305291617345986
attach=5.7178118990850635
Total cost=35.02310351643105
1e-10
Evaluated model with costs=35.02310351643105
Evaluated model with costs=35.022301784973095
Evaluated model with costs=35.021177619823575
Evaluated model with costs=35.023525259356724
Evaluated model with costs=35.02046063237382
Evaluated model with costs=35.021111474926656
Evaluated model with costs=35.018803895416106
Evaluated model with costs=35.0178483177464
Evaluated model with costs=35.01594670191487
Evaluated model with costs=35.014941223908785
Evaluated model with costs=35.01273525260814
Evaluated model with costs=35.00987025001528
Evaluated model with costs=35.007272481661204
Evaluated model with costs=35.03333055024449
Evaluated model with costs=35.0054825451902
Evaluated model with costs=35.00288225518113
Evaluated model with costs=34.99864342120379
Evaluated model with costs=35.05422767007492
Evaluated model with costs=34.99747795217559
Evaluated model with costs=34.99347873944825
Evaluated model with costs=34.99024110626664
Evaluated model with costs=34.99062217488019
Evaluated model with costs=34.987549313860086
Evaluated model with costs=34.987375810611454
Evaluated model with costs=34.98445045643433
================================================================================
Time: 3585.295334857001
Iteration: 86
Costs
deformation=29.231194086935773
attach=5.753256369498558
Total cost=34.98445045643433
1e-10
Evaluated model with costs=34.98445045643433
Evaluated model with costs=34.98334400219751
Evaluated model with costs=34.98173459546737
Evaluated model with costs=34.98158472319186
Evaluated model with costs=34.9808917236949
Evaluated model with costs=34.98020771199751
Evaluated model with costs=34.979490203334365
Evaluated model with costs=34.978473694654745
Evaluated model with costs=34.9800493708965
Evaluated model with costs=34.97782626360849
Evaluated model with costs=34.976965845863546
Evaluated model with costs=34.975805670909864
Evaluated model with costs=34.97500035951532
Evaluated model with costs=34.974351260631494
Evaluated model with costs=34.977317817055976
Evaluated model with costs=34.973732525174015
Evaluated model with costs=34.97315721089146
Evaluated model with costs=34.97303963652391
Evaluated model with costs=34.97256499664591
Evaluated model with costs=34.97219437717267
Evaluated model with costs=34.97158397142155
Evaluated model with costs=34.97106248817535
Evaluated model with costs=34.97049733902679
================================================================================
Time: 3627.3347167169995
Iteration: 87
Costs
deformation=29.219752616417846
attach=5.7507447226089425
Total cost=34.97049733902679
1e-10
Evaluated model with costs=34.97049733902679
Evaluated model with costs=34.970264129627864
Evaluated model with costs=34.9697706701686
Evaluated model with costs=34.96953385677711
Evaluated model with costs=34.97261419965376
Evaluated model with costs=34.96949827757708
Evaluated model with costs=34.969261001246124
Evaluated model with costs=34.96917701981074
Evaluated model with costs=34.969091500403906
Evaluated model with costs=34.96903413155299
Evaluated model with costs=34.969019587654806
Evaluated model with costs=34.968986854669886
Evaluated model with costs=34.96895656990517
Evaluated model with costs=34.9689250250855
Evaluated model with costs=34.968893695048095
Evaluated model with costs=34.968856041925946
Evaluated model with costs=34.96879007245206
Evaluated model with costs=34.96869689466201
Evaluated model with costs=34.96856617603074
Evaluated model with costs=34.968426614116964
Evaluated model with costs=34.96902507136142
Evaluated model with costs=34.968374436513244
Evaluated model with costs=34.96826972490564
================================================================================
Time: 3669.433112056002
Iteration: 88
Costs
deformation=29.224916946824326
attach=5.7433527780813165
Total cost=34.96826972490564
1e-10
Evaluated model with costs=34.96826972490564
Evaluated model with costs=34.96809397348084
Evaluated model with costs=34.97067143617542
Evaluated model with costs=34.968082024405824
Evaluated model with costs=34.967975749173945
Evaluated model with costs=34.9687869127997
Evaluated model with costs=34.967924685478586
Evaluated model with costs=34.967805875321105
Evaluated model with costs=34.96765033253918
Evaluated model with costs=34.96750966922215
Evaluated model with costs=34.967468374106126
Evaluated model with costs=34.967261189810905
Evaluated model with costs=34.96751831928114
Evaluated model with costs=34.96706846723875
Evaluated model with costs=34.96676502061355
Evaluated model with costs=34.96633708425993
Evaluated model with costs=34.96587613594992
Evaluated model with costs=34.96539244644442
Evaluated model with costs=34.96519892612605
Evaluated model with costs=34.96485736286397
Evaluated model with costs=34.96431531373616
Evaluated model with costs=34.96383707014893
Evaluated model with costs=34.963128197447986
Evaluated model with costs=34.96270358182202
Evaluated model with costs=34.96285107514239
Evaluated model with costs=34.96226712697901
================================================================================
Time: 3716.9395644210017
Iteration: 89
Costs
deformation=29.23150401282694
attach=5.730763114152069
Total cost=34.96226712697901
1e-10
Evaluated model with costs=34.96226712697901
Evaluated model with costs=34.961710486437546
Evaluated model with costs=34.97049062735865
Evaluated model with costs=34.96126559929209
Evaluated model with costs=34.96032368499605
Evaluated model with costs=34.96039401972391
Evaluated model with costs=34.958214049929005
Evaluated model with costs=34.95626806229541
Evaluated model with costs=34.96537368827185
Evaluated model with costs=34.95468911724532
Evaluated model with costs=34.951865427543396
Evaluated model with costs=34.9485538636889
Evaluated model with costs=34.94564164634585
Evaluated model with costs=34.982684451076025
Evaluated model with costs=34.94391106321441
Evaluated model with costs=34.941237770644804
Evaluated model with costs=34.93672866772893
Evaluated model with costs=34.94535283611815
Evaluated model with costs=34.93543147184054
Evaluated model with costs=34.93084066680299
Evaluated model with costs=34.92840367983088
Evaluated model with costs=34.92476903874393
Evaluated model with costs=34.94757169687867
Evaluated model with costs=34.92145707003476
Evaluated model with costs=34.9173713836846
================================================================================
Time: 3762.899123985
Iteration: 90
Costs
deformation=29.26174282286791
attach=5.6556285608166945
Total cost=34.9173713836846
1e-10
Evaluated model with costs=34.9173713836846
Evaluated model with costs=34.930001263993105
Evaluated model with costs=34.91312900133879
Evaluated model with costs=34.90993637886095
Evaluated model with costs=34.98261981488255
Evaluated model with costs=34.90166622524286
Evaluated model with costs=34.89441165648497
Evaluated model with costs=34.88582981697764
Evaluated model with costs=34.98269006293122
Evaluated model with costs=34.88003745517897
Evaluated model with costs=34.87244961041149
Evaluated model with costs=34.86943624830356
Evaluated model with costs=34.85783527719102
Evaluated model with costs=34.93477174317813
Evaluated model with costs=34.852599918878354
Evaluated model with costs=34.845367466214704
Evaluated model with costs=34.83666408450792
Evaluated model with costs=34.83174763078327
Evaluated model with costs=34.87957489184974
Evaluated model with costs=34.829339509789726
Evaluated model with costs=34.82374925042009
Evaluated model with costs=34.80946720462387
Evaluated model with costs=35.27626783112562
Evaluated model with costs=34.80435019503986
Evaluated model with costs=34.796448471613694
================================================================================
Time: 3808.746615080003
Iteration: 91
Costs
deformation=29.51623519908724
attach=5.280213272526453
Total cost=34.796448471613694
1e-10
Evaluated model with costs=34.796448471613694
Evaluated model with costs=34.78548256908512
Evaluated model with costs=34.805975956876665
Evaluated model with costs=34.77634240973187
Evaluated model with costs=34.766968287389936
Evaluated model with costs=34.759226272270155
Evaluated model with costs=34.77862149452784
Evaluated model with costs=34.75315561371012
Evaluated model with costs=34.74557023035658
Evaluated model with costs=34.73418990707508
Evaluated model with costs=34.73131921638738
Evaluated model with costs=34.740979825035865
Evaluated model with costs=34.72767226117402
Evaluated model with costs=34.7218196377939
Evaluated model with costs=34.71491099809854
Evaluated model with costs=34.71090846968387
Evaluated model with costs=34.712318643850026
Evaluated model with costs=34.7081213907882
Evaluated model with costs=34.70473126395656
Evaluated model with costs=34.98128579997413
Evaluated model with costs=34.701674860523994
Evaluated model with costs=34.703725560204006
Evaluated model with costs=34.68723287126797
Evaluated model with costs=34.67580525008351
Evaluated model with costs=34.664237808095294
================================================================================
Time: 3853.9643999380023
Iteration: 92
Costs
deformation=29.352206192082324
attach=5.312031616012973
Total cost=34.664237808095294
1e-10
Evaluated model with costs=34.664237808095294
Evaluated model with costs=34.996326303916646
Evaluated model with costs=34.65633770131496
Evaluated model with costs=34.63632377119539
Evaluated model with costs=34.61499807994946
Evaluated model with costs=34.636762510639926
Evaluated model with costs=34.61290987287142
Evaluated model with costs=34.6013462092089
Evaluated model with costs=34.596467067652476
Evaluated model with costs=34.590728115829975
Evaluated model with costs=34.61971159843011
Evaluated model with costs=34.588133407872704
Evaluated model with costs=34.5857048505863
Evaluated model with costs=34.578904773708764
Evaluated model with costs=34.57400285661971
Evaluated model with costs=34.572219318247924
Evaluated model with costs=34.56803703963163
Evaluated model with costs=34.564276876631524
Evaluated model with costs=34.56186126973276
Evaluated model with costs=34.55748538088677
Evaluated model with costs=34.5533360765011
Evaluated model with costs=34.54963956900903
Evaluated model with costs=34.55632992168766
Evaluated model with costs=34.54864227241919
Evaluated model with costs=34.54458705549725
================================================================================
Time: 3899.6778883549996
Iteration: 93
Costs
deformation=29.64608499401904
attach=4.8985020614782115
Total cost=34.54458705549725
1e-10
Evaluated model with costs=34.54458705549725
Evaluated model with costs=34.545301795767905
Evaluated model with costs=34.54093350351697
Evaluated model with costs=34.53838877097019
Evaluated model with costs=34.523949788973795
Evaluated model with costs=34.510292303667605
Evaluated model with costs=34.565258287655716
Evaluated model with costs=34.49274680500551
Evaluated model with costs=34.466208573297436
Evaluated model with costs=35.257438930662204
Evaluated model with costs=34.44733359637159
Evaluated model with costs=34.60031978918251
Evaluated model with costs=34.41381970311312
Evaluated model with costs=34.40091827704557
Evaluated model with costs=34.39148498993502
Evaluated model with costs=34.387170386816244
Evaluated model with costs=34.38307628114046
Evaluated model with costs=34.379404678733046
Evaluated model with costs=34.37607175225434
Evaluated model with costs=34.37395575333036
Evaluated model with costs=34.372106544902095
Evaluated model with costs=34.36898411126753
Evaluated model with costs=34.36596931541773
Evaluated model with costs=34.35799811583864
Evaluated model with costs=34.34039447219581
================================================================================
Time: 3945.3580352320023
Iteration: 94
Costs
deformation=29.319869223940227
attach=5.020525248255581
Total cost=34.34039447219581
1e-10
Evaluated model with costs=34.34039447219581
Evaluated model with costs=125.63380808882923
Evaluated model with costs=35.69399990591991
Evaluated model with costs=34.36386850976342
Evaluated model with costs=34.33702748006748
Evaluated model with costs=34.32169132194737
Evaluated model with costs=34.30967359491957
Evaluated model with costs=72.1776178505257
Evaluated model with costs=34.60471094645556
Evaluated model with costs=34.3095732393038
Evaluated model with costs=34.29891658444055
Evaluated model with costs=34.3390341341005
Evaluated model with costs=34.29443948100163
Evaluated model with costs=34.287938221072
Evaluated model with costs=34.298845421025
Evaluated model with costs=34.2787629962048
Evaluated model with costs=34.27189499774006
Evaluated model with costs=34.26229136384125
Evaluated model with costs=34.24741904298104
Evaluated model with costs=34.27028919270185
Evaluated model with costs=34.23923292516049
Evaluated model with costs=34.23646150440369
Evaluated model with costs=34.22741053966289
Evaluated model with costs=34.21647188545627
Evaluated model with costs=34.20691078232342
================================================================================
Time: 3991.1472108609996
Iteration: 95
Costs
deformation=29.59490804381933
attach=4.6120027385040885
Total cost=34.20691078232342
1e-10
Evaluated model with costs=34.20691078232342
Evaluated model with costs=34.210130609697075
Evaluated model with costs=34.202791601626046
Evaluated model with costs=34.19507063563948
Evaluated model with costs=34.18933370371655
Evaluated model with costs=34.18271090520659
Evaluated model with costs=34.18588128665585
Evaluated model with costs=34.17783624035603
Evaluated model with costs=34.17337782060454
Evaluated model with costs=34.16958176166099
Evaluated model with costs=34.16389961256637
Evaluated model with costs=34.15879941334514
Evaluated model with costs=34.15855792367996
Evaluated model with costs=34.15293508874155
Evaluated model with costs=34.14841089081317
Evaluated model with costs=34.144124876179966
Evaluated model with costs=34.137992169329145
Evaluated model with costs=34.133907375682206
Evaluated model with costs=34.152012535640665
Evaluated model with costs=34.131662483776196
Evaluated model with costs=34.127838583434695
Evaluated model with costs=34.12258147039282
Evaluated model with costs=34.13283477540628
Evaluated model with costs=34.12043057126704
Evaluated model with costs=34.118926052704346
================================================================================
Time: 4036.7738983130002
Iteration: 96
Costs
deformation=29.647402367207114
attach=4.471523685497232
Total cost=34.118926052704346
1e-10
Evaluated model with costs=34.118926052704346
Evaluated model with costs=34.11567167509397
Evaluated model with costs=34.11281933350421
Evaluated model with costs=34.1105370426356
Evaluated model with costs=34.10805144404228
Evaluated model with costs=34.10606889883294
Evaluated model with costs=34.105803825291716
Evaluated model with costs=34.10240092694388
Evaluated model with costs=34.097045972681
Evaluated model with costs=34.0886465980259
Evaluated model with costs=34.10374872803145
Evaluated model with costs=34.08568686266626
Evaluated model with costs=34.07513210934966
Evaluated model with costs=34.06519937249331
Evaluated model with costs=34.11991899808855
Evaluated model with costs=34.054271352047294
Evaluated model with costs=34.04205484901177
Evaluated model with costs=34.25670769223755
Evaluated model with costs=34.031175055102736
Evaluated model with costs=34.020543136586184
Evaluated model with costs=34.01471913656246
Evaluated model with costs=34.00911968155546
Evaluated model with costs=34.006158088737
Evaluated model with costs=34.00493929446063
Evaluated model with costs=34.002451726306354
================================================================================
Time: 4082.5569941210015
Iteration: 97
Costs
deformation=29.46842214835302
attach=4.5340295779533335
Total cost=34.002451726306354
1e-10
Evaluated model with costs=34.002451726306354
Evaluated model with costs=33.99940009276868
Evaluated model with costs=33.99719777313457
Evaluated model with costs=33.99243785327782
Evaluated model with costs=33.99197804844137
Evaluated model with costs=33.99033110871328
Evaluated model with costs=33.989010820879905
Evaluated model with costs=33.986195314738794
Evaluated model with costs=33.98352462376521
Evaluated model with costs=33.97952917978796
Evaluated model with costs=34.01894278792682
Evaluated model with costs=33.97568560359747
Evaluated model with costs=33.96913786545832
Evaluated model with costs=33.96489204393173
Evaluated model with costs=33.95258347820181
Evaluated model with costs=33.993805981360524
Evaluated model with costs=33.948417588121046
Evaluated model with costs=33.937407895426006
Evaluated model with costs=33.9454645943992
Evaluated model with costs=33.93360413314841
Evaluated model with costs=33.93050424035458
Evaluated model with costs=33.92898965140305
Evaluated model with costs=33.92174723098792
Evaluated model with costs=33.94105466887673
Evaluated model with costs=33.91752033644366
================================================================================
Time: 4128.437577727
Iteration: 98
Costs
deformation=29.56778113225369
attach=4.349739204189973
Total cost=33.91752033644366
1e-10
Evaluated model with costs=33.91752033644366
Evaluated model with costs=33.910484259719865
Evaluated model with costs=33.99303244032644
Evaluated model with costs=33.904418125634216
Evaluated model with costs=33.89912227145524
Evaluated model with costs=33.89141031515441
Evaluated model with costs=33.891111185555886
Evaluated model with costs=33.888254021641934
Evaluated model with costs=33.883237680833595
Evaluated model with costs=33.88134282067307
Evaluated model with costs=33.874453951535415
Evaluated model with costs=33.87784979227162
Evaluated model with costs=33.86667166243949
Evaluated model with costs=33.862786081201904
Evaluated model with costs=33.8587522210489
Evaluated model with costs=33.85451664946156
Evaluated model with costs=33.861862714710526
Evaluated model with costs=33.8502509471754
Evaluated model with costs=33.846103819174644
Evaluated model with costs=33.84127201475252
Evaluated model with costs=33.837375028312906
Evaluated model with costs=34.03135184467503
Evaluated model with costs=33.83667186804675
Evaluated model with costs=33.836018599750275
Evaluated model with costs=33.834521775526824
================================================================================
Time: 4174.349066442002
Iteration: 99
Costs
deformation=29.53110446406937
attach=4.303417311457451
Total cost=33.834521775526824
================================================================================
Optimisation process exited with message: Total number of iterations reached.
Final cost=33.834521775526824
Model evaluation count=2473
Time elapsed = 4174.349574388001
Compute optimized deformation trajectory.
intermediates = {}
with torch.autograd.no_grad():
deformed = refit_model.compute_deformed(shoot_solver, shoot_it, intermediates=intermediates)
deformed_shape = deformed[0][0]
deformed_growth = intermediates['states'][-1][2].gd[0]
deformed_growth_rot = intermediates['states'][-1][2].gd[1]
Plot results.
plt.subplot(1, 3, 1)
plt.title("Source")
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), '-')
plt.plot(points_growth[:, 0].numpy(), points_growth[:, 1].numpy(), '.')
plt.axis(aabb_target.totuple())
plt.axis('equal')
plt.subplot(1, 3, 2)
plt.title("Deformed source")
plt.plot(deformed_shape[:, 0], deformed_shape[:, 1], '-')
plt.axis(aabb_target.totuple())
plt.axis('equal')
plt.subplot(1, 3, 3)
plt.title("Deformed source and target")
plt.plot(shape_target[:, 0].numpy(), shape_target[:, 1].numpy(), '-')
plt.plot(deformed_shape[:, 0], deformed_shape[:, 1], '-')
plt.plot(deformed_growth[:, 0], deformed_growth[:, 1], '.')
plt.axis(aabb_target.totuple())
plt.axis('equal')
plt.show()
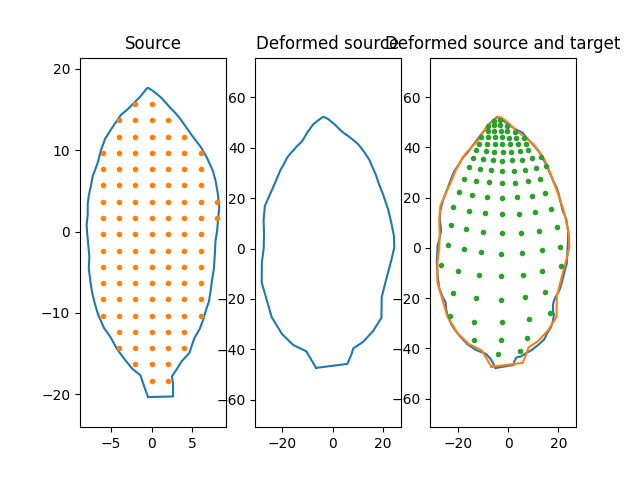
Recompute the learned deformation trajectory this time with the grid deformation to visualize growth.
modules = imodal.DeformationModules.CompoundModule(copy.copy(refit_model.modules))
modules.manifold.fill(refit_model.init_manifold)
square_size = 1.
grid_resolution = [math.floor(aabb_source.width/square_size),
math.floor(aabb_source.height/square_size)]
deformation_grid = imodal.DeformationModules.DeformationGrid(aabb_source, growth_grid_resolution)
controls = [control[1:] for control in intermediates['controls']]
deformable_shape = imodal.Models.DeformablePoints(shape_source)
deformable_shape.silent_module.manifold.cotan = refit_model.init_manifold[0].cotan
deformable_grid = imodal.Models.DeformableGrid(aabb_source, grid_resolution)
intermediates = {}
with torch.autograd.no_grad():
imodal.Models.deformables_compute_deformed([deformable_shape, deformable_grid], modules[1:], shoot_solver, shoot_it, intermediates=intermediates, controls=controls)
Plot the growth trajectory.
indices = [0, 3, 7, 10]
fig = plt.figure(figsize=[5.*len(indices), 5.])
for i, index in enumerate(indices):
state = intermediates['states'][index]
ax = plt.subplot(1, len(indices), i + 1)
deformable_grid.silent_module.manifold.fill_gd(state[1].gd)
grid_x, grid_y = deformable_grid.silent_module.togrid()
imodal.Utilities.plot_grid(ax, grid_x, grid_y, color='xkcd:light blue', lw=0.4)
plt.plot(shape_source[:, 0].numpy(), shape_source[:, 1].numpy(), color='black')
plt.plot(shape_target[:, 0].numpy(), shape_target[:, 1].numpy(), color='red')
plt.plot(state[0].gd[:, 0].numpy(), state[0].gd[:, 1].numpy())
plt.axis('equal')
plt.axis('off')
fig.tight_layout()
plt.show()
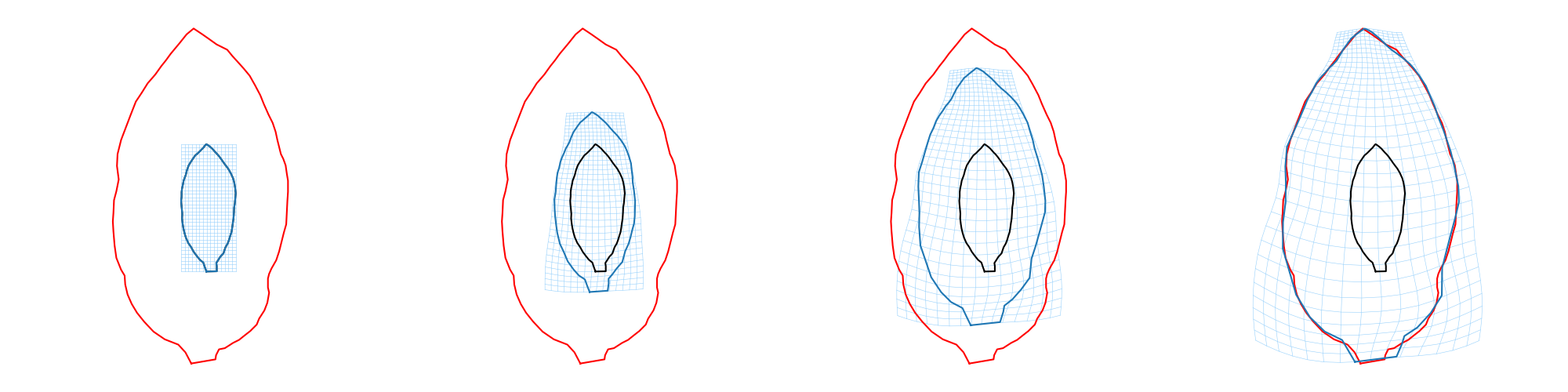
Total running time of the script: ( 105 minutes 2.094 seconds)